TypeError: float argument required, not str
时间: 2024-04-13 22:29:49 浏览: 19
抱歉,我之前给出的回答有误。如果要将字符串转换为整数,可以使用int()函数。例如:
```python
str_num = "123"
int_num = int(str_num)
print(int_num) # 输出为123
```
但是,请注意,如果字符串中包含非数字字符,则会引发ValueError异常。因此,在转换之前,请确保字符串只包含有效的整数表示。
相关问题
TypeError: float argument required, not Variable
这个错误通常表示你在将一个 Variable 对象传递给需要 float 参数的函数时出错了。Variable 是 PyTorch 中的一个类,用于存储张量(Tensor)和梯度信息。如果函数需要一个 float 参数,但是你传递了一个 Variable 对象,就会出现这个错误。
解决方法是将 Variable 转换成 float。可以使用 Variable 的 data 属性获取 Tensor 对象,然后使用 Tensor 的 item() 方法将其转换为 float。例如:
```
x = Variable(torch.FloatTensor([1.0]))
y = my_function(x.data.item())
```
这里假设 my_function 需要一个 float 参数,并且 x 是一个包含一个值为 1.0 的浮点数的 Variable 对象。我们使用 x.data.item() 将其转换为 float,并且传递给 my_function。
zip TypeError: float object is not iterable
This error occurs when you try to iterate over a float object using a loop. A float is a numeric data type in Python that represents a decimal number. However, you cannot iterate over a float as it is not an iterable object.
For example, suppose you have a float value and you try to iterate over it using a for loop:
```
my_float = 3.14
for num in my_float:
print(num)
```
This code will result in a TypeError because you cannot iterate over a float.
To fix this error, you need to ensure that you are iterating over an iterable object, such as a list or a tuple. If you need to convert a float to an iterable object, you can do so by wrapping it in a list or tuple:
```
my_float = 3.14
my_list = [my_float]
for num in my_list:
print(num)
```
This code will iterate over the list containing the float value, rather than the float itself.
相关推荐
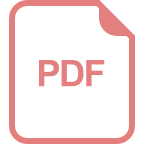
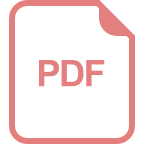
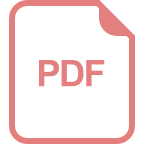












