python 使用ctypes 调用c++ DLL 怎么传参数为一维数组
时间: 2024-05-05 21:18:57 浏览: 20
您可以使用ctypes模块的`POINTER`类型和`cast()`函数来传递一维数组参数。
例如,假设您要调用一个名为`my_func`的C函数,该函数接受一个指向`int`类型的一维数组的指针作为参数,您可以按照以下步骤进行操作:
首先,导入ctypes模块并加载DLL文件:
```python
import ctypes
my_dll = ctypes.cdll.LoadLibrary('my_dll_name.dll')
```
然后,定义一个Python列表作为数组参数,并使用`ctypes.c_int`转换其元素类型:
```python
my_array = [1, 2, 3, 4, 5]
my_array_c = (ctypes.c_int * len(my_array))(*my_array)
```
接下来,将数组的指针传递给C函数,并设置参数类型为`POINTER(ctypes.c_int)`:
```python
my_func = my_dll.my_func
my_func.argtypes = [ctypes.POINTER(ctypes.c_int)]
my_func.restype = None
my_func(my_array_c)
```
这将把`my_array_c`指针传递给`my_func`函数,在C中就可以像操作普通数组一样使用它。
相关问题
python 使用ctypes 调用c++ DLL 怎么传参数为二维数组的指针
您好,感谢您的问题。关于使用ctypes调用c DLL传递二维数组指针的问题,可以按照以下步骤进行操作:
1. 在Python中定义一个二维数组,可以使用numpy库或其他方式创建;
2. 将二维数组转换为C语言中的指针类型,可以使用numpy的.ctypes属性;
3. 在C语言DLL中定义一个接受二维数组指针类型参数的函数,并传入转换后的指针;
4. 在Python中调用DLL函数,传入转换后的指针参数即可。
下面是一个示例代码,供您参考:
在C语言DLL中定义函数:
```
// testdll.c
#include <stdio.h>
void testfunc(double **data, int rows, int cols) {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
printf("%lf ", data[i][j]);
}
printf("\n");
}
}
```
在Python中调用DLL函数:
```
import numpy as np
import ctypes
# 加载C语言DLL
dll = ctypes.CDLL('./testdll.so')
# 定义测试数据
data = np.array([[1.0, 2.0], [3.0, 4.0]])
# 转换为C语言指针类型
data_ptr = (data.__array_interface__['data'][0] + np.arange(data.shape[0]) * data.strides[0]).astype(np.uintp)
data_ptr_ptr = ctypes.cast(data_ptr, ctypes.POINTER(ctypes.POINTER(ctypes.c_double)))
# 调用C语言DLL函数
dll.testfunc(data_ptr_ptr, data.shape[0], data.shape[1])
```
希望以上代码对您有所帮助。如果您还有其他问题,欢迎继续向我提问。
python 安装ctypes 调用c++ dll文件示例
下面是一个简单示例,演示如何在 Python 中使用 `ctypes` 调用 C++ 编写的 DLL 文件:
1. 创建 C++ DLL 文件
首先,您需要创建一个 C++ 动态链接库(DLL)文件,其中包含您想要在 Python 中使用的 C++ 函数。下面是一个简单的示例,演示如何在 Visual Studio 中创建一个简单的 DLL 文件:
```cpp
// SampleDLL.cpp
#include "pch.h"
#include "SampleDLL.h"
extern "C"
{
int add(int x, int y)
{
return x + y;
}
}
```
请注意,我们使用 `extern "C"` 来告诉编译器使用 C 语言的函数命名规则,以便在 Python 中调用。
2. 编译 DLL 文件
使用 Visual Studio,您可以将上述代码编译为 DLL 文件。在生成 DLL 文件之前,您需要将项目属性中的运行库设置为“多线程 DLL (/MD)”。
3. 在 Python 中使用 ctypes 调用 DLL
在 Python 中,您可以使用 `ctypes` 模块来调用 DLL 中的函数。下面是一个简单的示例:
```python
import ctypes
# 加载 DLL 文件
sample_dll = ctypes.CDLL('SampleDLL.dll')
# 调用 DLL 中的函数
result = sample_dll.add(1, 2)
# 输出结果
print(result)
```
在上面的示例中,我们首先使用 `ctypes.CDLL()` 函数加载 DLL 文件。然后,我们可以使用 `sample_dll.add()` 来调用 DLL 文件中的 `add()` 函数。最后,我们输出了结果。
请确保在加载 DLL 文件时使用正确的文件名和路径。如果 DLL 文件不在当前工作目录中,则需要指定完整路径。
希望这个示例能够帮助您了解如何在 Python 中使用 `ctypes` 调用 C++ 编写的 DLL 文件。
相关推荐
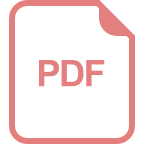
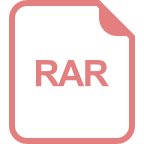
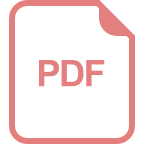












