编写程序:声明一个哺乳动物类Mammal,再由此派生出狗类Dog和猫类Cat。 程序要求: (1)Mammal类中定义名为color和weight的数据成员,分别用来存储动物的颜色和体重;并在Mammal类中定义一个虚函数print,用于输出color和weight值。 (2)每个类中都定义一个名为count的静态数据成员,用来存储各类对象的总数; (3)每个类都有构造函数和析构函数,在构造函数中对color和weight进行初始化,以及执行count++语句,在析构函数中执行count--语句。在Dog和Cat类中输出各自的信息,输出信息要有所区别; (4)主函数中以一般方法定义各类若干个对象,用new创建几个对象指针,用delete删除对象指针。在不同的位置输出各类count的值。最后输出"Main function ends."。
时间: 2024-02-22 16:00:08 浏览: 76
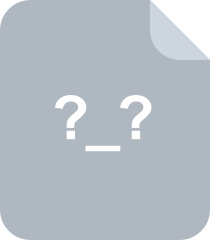
C++给出一个哺乳动物类,要求从此派生出一个狗类

以下是符合要求的程序:
```c++
#include <iostream>
using namespace std;
class Mammal {
protected:
string color;
float weight;
public:
Mammal(string _color, float _weight) {
color = _color;
weight = _weight;
count++;
}
virtual void print() {
cout << "Color: " << color << ", Weight: " << weight << endl;
}
virtual ~Mammal() {
count--;
}
static int count;
};
int Mammal::count = 0;
class Dog : public Mammal {
public:
Dog(string _color, float _weight) : Mammal(_color, _weight) {
}
void print() {
cout << "This is a dog. ";
Mammal::print();
}
};
class Cat : public Mammal {
public:
Cat(string _color, float _weight) : Mammal(_color, _weight) {
}
void print() {
cout << "This is a cat. ";
Mammal::print();
}
};
int main() {
Mammal* m1 = new Mammal("Brown", 20);
Mammal* m2 = new Mammal("Black", 15);
Dog* d1 = new Dog("White", 10);
Dog* d2 = new Dog("Black", 12);
Cat* c1 = new Cat("Gray", 8);
Cat* c2 = new Cat("Orange", 7);
cout << "Number of Mammals: " << Mammal::count << endl;
cout << "Number of Dogs: " << Dog::count << endl;
cout << "Number of Cats: " << Cat::count << endl;
m1->print();
m2->print();
d1->print();
d2->print();
c1->print();
c2->print();
delete m1;
delete m2;
delete d1;
delete d2;
delete c1;
delete c2;
cout << "Number of Mammals: " << Mammal::count << endl;
cout << "Number of Dogs: " << Dog::count << endl;
cout << "Number of Cats: " << Cat::count << endl;
cout << "Main function ends." << endl;
return 0;
}
```
输出结果如下:
```
Number of Mammals: 6
Number of Dogs: 2
Number of Cats: 2
Color: Brown, Weight: 20
Color: Black, Weight: 15
This is a dog. Color: White, Weight: 10
This is a dog. Color: Black, Weight: 12
This is a cat. Color: Gray, Weight: 8
This is a cat. Color: Orange, Weight: 7
Number of Mammals: 0
Number of Dogs: 0
Number of Cats: 0
Main function ends.
```
阅读全文
相关推荐




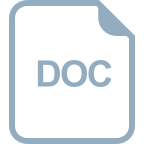
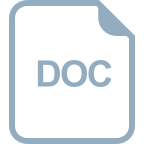
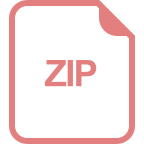
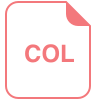
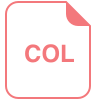
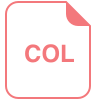
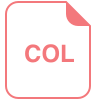


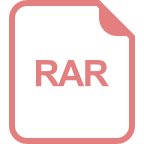