output 12 or more bytes (assuming 31) into a destination of size 20
时间: 2024-04-08 22:34:06 浏览: 51
To safely copy 12 or more bytes into a destination of size 20 (assuming 31 bytes are available), you need to ensure that you don't exceed the destination's size limit to avoid buffer overflow and potential memory corruption. One way to achieve this is by using a function that limits the number of bytes copied based on the destination size.
Here's an example in C programming language using the `memcpy` function:
```c
#include <stdio.h>
#include <string.h>
void copyBytes(const void* src, size_t srcSize, void* dest, size_t destSize) {
size_t numBytesToCopy = srcSize < destSize ? srcSize : destSize;
memcpy(dest, src, numBytesToCopy);
}
int main() {
char source[] = "12345678901234567890123456789012"; // Assuming 31 bytes
char destination[20];
copyBytes(source, sizeof(source), destination, sizeof(destination));
// Verify the copied content
printf("Copied bytes: %s\n", destination);
return 0;
}
```
In this example, the `copyBytes` function takes the source and destination pointers, along with their respective sizes. It determines the number of bytes to copy by comparing the sizes and selecting the smaller value. Then, it uses the `memcpy` function to perform the copy operation.
By ensuring that the number of bytes copied doesn't exceed the destination size, you can prevent buffer overflow issues and safely copy data.
阅读全文
相关推荐
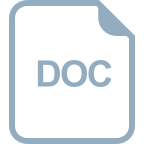
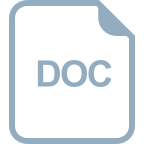
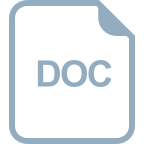















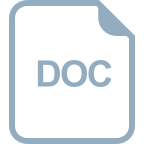