如何判断数组中是否存在某一元素
时间: 2024-11-25 16:01:41 浏览: 8
在许多编程语言中,判断数组中是否存在某个特定元素通常有多种方法:
1. **遍历法**:可以使用for循环或while循环遍历整个数组,检查每个元素是否等于目标值。如果找到匹配项,返回`true`,遍历结束仍未找到则返回`false`。
```python
def contains(arr, target):
for elem in arr:
if elem == target:
return True
return False
```
2. **列表推导式**(Python):利用Python的列表推导特性,一次性检查所有元素。
```python
def contains(arr, target):
return target in arr
```
3. **内置函数**(JavaScript、PHP等):某些语言提供内置的成员运算符如`in`,可以直接用于检测数组元素是否存在。
```javascript
function contains(arr, target) {
return arr.includes(target);
}
```
相关问题
js判断数组中是否存在某值
在JavaScript中,我们可以使用indexOf()方法来判断数组中是否存在某个特定值。该方法返回索引值,如果值存在于数组中,则返回该值的第一个出现位置的索引。如果值不存在,则返回-1。
以下是一个示例代码片段,演示如何使用此方法进行数组搜索:
var myArray = ['apple', 'banana', 'grape', 'orange'];
if (myArray.indexOf('grape') !== -1) {
console.log('Grape is found in the array.');
} else {
console.log('Grape is not found in the array.');
}
在上面的代码中,我们定义了一个数组myArray,并通过indexOf方法搜索特定值'grape'。如果'grape'存在于数组中,则if语句将返回true,因此将输出“Grape is found in the array”,否则输出“Grape is not found in the array”。
此方法还可以与JavaScript中的其他条件语句一起使用。例如,您可以使用while循环来遍历整个数组,查找特定值的出现:
var myArray = ['apple', 'banana', 'grape', 'orange'];
var i = 0;
while (i < myArray.length) {
if (myArray[i] === 'grape') {
console.log('Grape is found at index ' + i + ' in the array.');
break;
}
i++;
}
在上面的代码中,我们使用while循环遍历数组中的每个元素,并使用if语句查找'grape'的出现位置。如果找到该值,则break语句将退出循环,并输出找到该值的位置。如果未找到,则循环将继续执行,并在结束时输出未找到该值的消息。
总之,使用indexOf()方法可以很容易地在JavaScript中查找数组中的特定值。您可以根据自己的需要使用它来编写各种逻辑应用。
go判断数组中是否存在某值
可以使用for循环遍历数组,逐一判断数组中的每个元素是否等于目标值。代码示例如下:
```go
func isExist(arr []int, target int) bool {
for _, value := range arr {
if value == target {
return true
}
}
return false
}
```
其中,`arr`为要判断的数组,`target`为要查找的目标值。如果数组中存在目标值,则返回`true`,否则返回`false`。
阅读全文
相关推荐
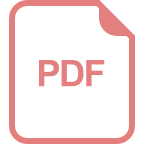
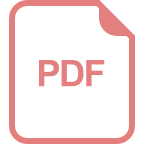
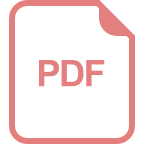













