java2.ATM取款问题,编写一个程序模拟取款,用户最多尝试输入密码三次,密码正确后输入取款金额。 ATM 只能输出100的纸币,要求取款金额在100-1000之间,且为100的倍数
时间: 2024-10-09 15:12:16 浏览: 38
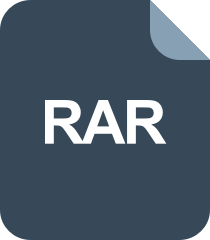
JAVA-ATM.rar_Java 实现 自动 取款机 模拟 程序_atm java_java ATM_java 银行_自动提款
在Java中,我们可以创建一个ATM模拟类,它包含一个循环来处理用户的密码验证、取款请求以及交易限制。下面是一个简单的示例:
```java
import java.util.Scanner;
class User {
private String password;
private int attempts;
public User(String password) {
this.password = password;
this.attempts = 0;
}
// 密码尝试次数限制
public boolean checkPassword() {
if (attempts < 3) {
return true; // 没有达到尝试上限
} else {
System.out.println("密码输入错误过多,账户锁定");
return false;
}
}
public void setPassword(String newPassword) {
this.password = newPassword;
attempts = 0; // 清零尝试次数
}
public void increaseAttempts() {
attempts++;
}
}
public class ATM {
private User user;
public ATM(String initialPassword) {
user = new User(initialPassword);
}
public void deposit(int amount) {
// 实际存款操作,这里仅做示例
System.out.println("存款成功");
}
public void withdraw(int amount) {
if (amount >= 100 && amount % 100 == 0 && amount <= 1000) {
if (user.checkPassword()) {
user.deposit(-amount); // 存款模拟取款
System.out.printf("取款 %.0f 元成功。\n", amount);
user.increaseAttempts();
} else {
System.out.println("请先输入正确的密码");
}
} else {
System.out.println("取款金额不在100到1000元之间,或不是100的倍数");
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
ATM atm = new ATM("password123"); // 示例初始密码
System.out.println("请输入您的密码:");
String inputPassword = scanner.nextLine();
atm.withdraw(scanner.nextInt());
scanner.close();
}
}
```
在这个程序中,用户需要输入正确的密码才能进行取款操作。如果密码错误超过三次,账户会被锁定。取款金额必须在100至1000元之间,并且是100的整数倍。
阅读全文
相关推荐
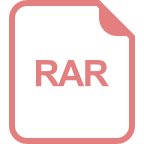
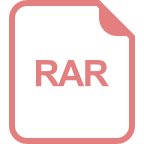

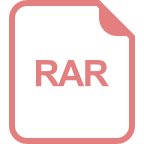
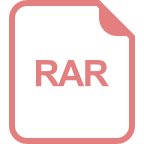
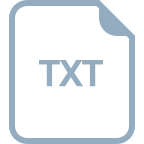
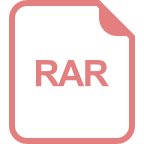
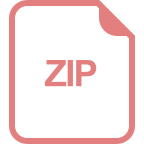
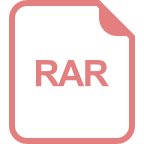
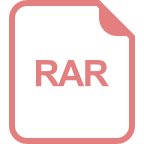
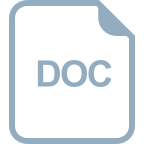




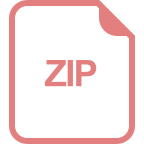