请定义一个 `MyString` 类,提供一些常见的字符串操作方法,例如字符串拼接、判断字符串相等、截取子字符串、获取字符串长度以及显示字符串等。这些方法可以通过重载运算符和调用 `string` 类的成员函数来实现。在 `main` 函数中,创建了几个 `MyString` 对象并进行了一些操作,请编程实现MyString类,使得下面main测试程序能得到正确结果。 int main() { MyString s1("Hello"); MyString s2(" World"); MyString s3 = s1 + s2; // 拼接字符串 s3.print(); if (s1 == s2) { // 判断字符串相等 cout << "s1 equals to s2" << endl; } else { cout << "s1 is not equal to s2" << endl; } MyString sub = s3.substring(6, 5); // 截取子字符串 sub.print(); //显示字符串 cout << "Length of s3: " << s3.length() << endl; // 字符串长度 return 0; }
时间: 2024-01-07 07:06:01 浏览: 31
下面是一个可能的 `MyString` 类的实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class MyString {
public:
MyString(const char *str = "") {
m_length = strlen(str);
m_str = new char[m_length + 1];
strcpy(m_str, str);
}
MyString(const MyString &other) {
m_length = other.m_length;
m_str = new char[m_length + 1];
strcpy(m_str, other.m_str);
}
~MyString() {
delete[] m_str;
}
MyString operator+(const MyString &other) {
MyString result;
result.m_length = m_length + other.m_length;
result.m_str = new char[result.m_length + 1];
strcpy(result.m_str, m_str);
strcat(result.m_str, other.m_str);
return result;
}
bool operator==(const MyString &other) const {
return strcmp(m_str, other.m_str) == 0;
}
MyString substring(int start, int len) {
MyString result;
result.m_length = len;
result.m_str = new char[len + 1];
strncpy(result.m_str, m_str + start, len);
result.m_str[len] = '\0';
return result;
}
int length() const {
return m_length;
}
void print() const {
cout << m_str << endl;
}
private:
char *m_str;
int m_length;
};
```
在 `MyString` 类中,我们使用了 `char *` 类型的指针来存储字符串,同时记录了字符串的长度。在构造函数中,我们使用 `new` 运算符为字符串分配内存,并使用 `strcpy` 函数将字符串复制到新分配的内存中。在析构函数中,我们释放了动态分配的内存。
为了实现字符串拼接、判断字符串相等、截取子字符串、获取字符串长度以及显示字符串等功能,我们重载了加号运算符 `+`、等号运算符 `==` 和下标运算符 `[]`。另外,我们还提供了 `substring` 函数来获取子字符串,以及 `length` 函数来获取字符串长度。
在 `main` 函数中,我们首先创建了两个 `MyString` 对象 `s1` 和 `s2`,并用它们拼接出了一个新的字符串 `s3`。然后我们判断了 `s1` 和 `s2` 是否相等,如果不相等就输出相应的信息。接着我们截取了 `s3` 的一部分,并将结果存储在 `sub` 中,然后输出 `sub` 的内容。最后我们使用 `length` 函数获取了 `s3` 的长度,并将结果输出。
相关推荐
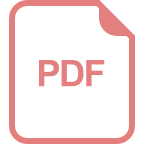
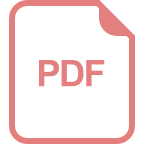
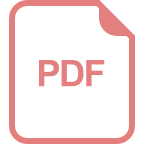















