用python描述双门限法对一段语音进行端点检测
时间: 2023-12-11 16:03:27 浏览: 39
双门限法是一种简单常用的端点检测算法,其基本思想是根据信号强度设置两个门限,当信号强度超过高门限时,认为信号处于语音段,当信号强度低于低门限时,认为信号处于非语音段。在两个门限之间的信号被认为是不确定的,需要进行进一步处理。
以下是一个用Python实现双门限法端点检测的示例代码:
```python
import numpy as np
import librosa
# 定义高低门限
high_threshold = 0.5
low_threshold = 0.1
# 加载音频文件
audio, sr = librosa.load('speech.wav', sr=None)
# 计算短时能量
frame_length = int(0.02 * sr) # 帧长20ms
frame_step = int(0.01 * sr) # 帧移10ms
energy = librosa.feature.rms(audio, frame_length=frame_length, hop_length=frame_step)
# 对能量值进行归一化处理
energy = (energy - np.min(energy)) / (np.max(energy) - np.min(energy))
# 设置起始状态
state = 'non_speech'
segments = []
start = 0
# 遍历每一帧
for i in range(len(energy[0])):
# 当前帧的能量值
e = energy[0][i]
# 判断状态转移
if state == 'non_speech' and e > high_threshold:
state = 'speech'
start = i
elif state == 'speech' and e < low_threshold:
state = 'non_speech'
segments.append((start * frame_step, i * frame_step))
# 输出检测到的语音段
for segment in segments:
print('Speech segment:', segment)
```
上述代码中,首先加载语音文件,然后计算每一帧的短时能量,并对能量值进行归一化处理。接着,遍历每一帧,根据当前状态和能量值判断状态转移,最后输出检测到的语音段。其中,每一帧的时间戳可以通过帧长和帧移计算得到。
相关推荐
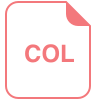
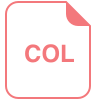
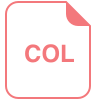
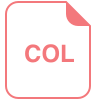
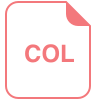









