C语言创建队列,将1,2,3,4,5,6,7插入其中并显示
时间: 2024-12-19 15:25:30 浏览: 5
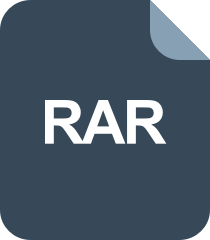
queue_C语言消息队列_

在C语言中,我们可以使用数组或者动态内存分配的数据结构如链表来创建一个队列。这里我将演示如何使用数组作为简单的队列数据结构:
```c
#include <stdio.h>
#define MAX_SIZE 10 // 队列最大容量
// 定义队列结构
typedef struct {
int data[MAX_SIZE];
int front; // 队头指针
int rear; // 队尾指针
} Queue;
// 初始化队列
void initQueue(Queue* queue) {
queue->front = -1;
queue->rear = -1;
}
// 插入元素到队尾
void enqueue(Queue* queue, int value) {
if ((queue->rear + 1) % MAX_SIZE == queue->front) { // 判断是否已满
printf("Queue is full.\n");
return;
}
queue->rear = (queue->rear + 1) % MAX_SIZE; // 环形更新队尾
queue->data[queue->rear] = value;
if (queue->front == -1) queue->front = 0; // 队空时更新队头
}
// 显示队列内容
void displayQueue(Queue* queue) {
if (queue->front == -1) {
printf("Queue is empty.\n");
return;
}
for (int i = queue->front; i != queue->rear; i = (i + 1) % MAX_SIZE) {
printf("%d ", queue->data[i]);
}
printf("\n");
}
int main() {
Queue queue;
initQueue(&queue);
// 插入元素
for (int i = 1; i <= 7; ++i) {
enqueue(&queue, i);
}
// 显示队列
displayQueue(&queue);
return 0;
}
```
在这个示例中,我们首先定义了一个队列结构,并初始化队头和队尾指针为-1表示队列为空。然后我们实现了`enqueue`操作用于添加元素到队尾,如果队列已满则会提示。`displayQueue`函数用于打印队列内的元素。
运行这个程序,你会看到队列1, 2, 3, 4, 5, 6, 7的顺序。注意,C语言数组实现的队列不是循环队列,所以当我们试图插入超过队列大小的元素时,会直接替换最前面的元素,这可能导致丢失信息。实际应用中,可能会选择链表等其他数据结构来避免这个问题。
阅读全文
相关推荐
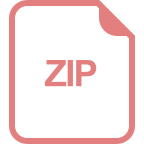
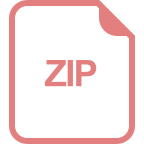

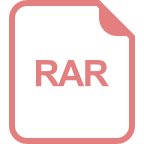
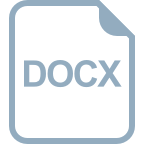
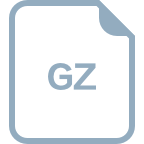
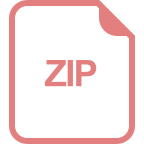
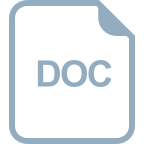
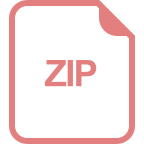
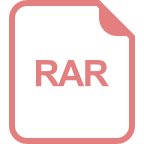
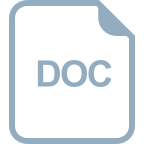
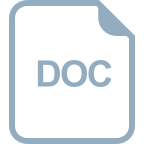
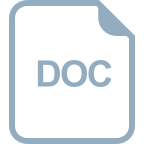
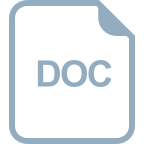
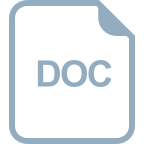


