用html,css和JavaScript写个圣诞树
时间: 2023-01-11 10:55:36 浏览: 89
好的,首先需要在HTML中创建一个`div`元素,用来作为圣诞树的容器。然后可以使用CSS来设置圣诞树的样式,比如背景色、大小等。
具体的HTML代码可以这样写:
```
<div id="christmas-tree">
</div>
```
接下来使用CSS来设置圣诞树的样式:
```css
#christmas-tree {
background-color: green;
width: 200px;
height: 300px;
}
```
最后,使用JavaScript可以在圣诞树上添加装饰物,比如圣诞球、蜡烛等。这样,就可以使用HTML、CSS和JavaScript创建一个圣诞树了。
具体的JavaScript代码可以这样写:
```javascript
const tree = document.getElementById('christmas-tree');
const ball = document.createElement('div');
ball.className = 'ball';
tree.appendChild(ball);
const candle = document.createElement('div');
candle.className = 'candle';
tree.appendChild(candle);
```
这样,在HTML中就会生成两个装饰物:一个圣诞球和一根蜡烛。
需要注意的是,这只是一个简单的例子,在实际应用中还需要根据需要进行更多的设置和调整。
相关问题
写个圣诞树,送给女朋友,使用前端html,css,JavaScript写
好的,这是一个使用 HTML、CSS 和 JavaScript 写的圣诞树的示例:
HTML:
```
<div id="christmas-tree">
<div class="star"></div>
<div class="trunk"></div>
<div class="tree-section">
<div class="tree-layer"></div>
<div class="tree-layer"></div>
<div class="tree-layer"></div>
<div class="tree-layer"></div>
<div class="tree-layer"></div>
</div>
<div class="gifts">
<div class="gift"></div>
<div class="gift"></div>
<div class="gift"></div>
<div class="gift"></div>
</div>
</div>
```
CSS:
```
#christmas-tree {
position: relative;
width: 400px;
height: 600px;
margin: 0 auto;
}
.star {
position: absolute;
top: -30px;
left: 50%;
transform: translateX(-50%);
width: 60px;
height: 60px;
border-radius: 50%;
background: gold;
box-shadow: 0 0 20px gold;
}
.trunk {
position: absolute;
bottom: 0;
left: 50%;
transform: translateX(-50%);
width: 40px;
height: 80px;
background: brown;
}
.tree-section {
position: absolute;
top: 50px;
left: 50%;
transform: translateX(-50%);
width: 200px;
height: 400px;
}
.tree-layer {
position: absolute;
top: 0;
left: 0;
right: 0;
bottom: 0;
border-radius: 50%;
background: green;
box-shadow: 0 0 20px green;
transform: translateY(-50%);
}
.tree-layer:nth-child(1) {
width: 200px;
height: 200px;
}
.tree-layer:nth-child(2) {
width: 180px;
height: 180px;
top: 20px;
}
.tree-layer:nth-child(3) {
width: 160px;
height: 160px;
top: 40px;
}
.tree-layer:nth-child(4) {
width: 140px;
height: 140px;
top: 60px;
}
.tree-layer:nth-child(5) {
width: 120px;
height: 120px;
top: 80px;
}
.gifts {
position: absolute;
bottom: 60px;
left: 50%;
transform: translateX(-50%);
display: flex;
}
.gift {
width: 50px;
height: 50px;
background
用css html js 给我写一个圣诞树
好的,以下是一个使用HTML,CSS和JavaScript创建的简单的圣诞树:
HTML代码:
```
<!DOCTYPE html>
<html>
<head>
<title>圣诞树</title>
<style>
#tree {
position: relative;
width: 150px;
height: 200px;
margin: 50px auto;
}
#tree:before {
content: "";
position: absolute;
top: 0;
left: 50%;
margin-left: -10px;
width: 0;
height: 0;
border-left: 10px solid transparent;
border-right: 10px solid transparent;
border-bottom: 20px solid green;
}
#tree:after {
content: "";
position: absolute;
top: 20px;
left: 50%;
margin-left: -50px;
width: 100px;
height: 150px;
background-color: green;
}
.ornament {
position: absolute;
border-radius: 50%;
}
.ornament1 {
background-color: red;
top: 40px;
left: 70px;
width: 20px;
height: 20px;
}
.ornament2 {
background-color: blue;
top: 70px;
left: 50px;
width: 15px;
height: 15px;
}
.ornament3 {
background-color: yellow;
top: 90px;
left: 80px;
width: 25px;
height: 25px;
}
</style>
</head>
<body>
<div id="tree">
<div class="ornament ornament1"></div>
<div class="ornament ornament2"></div>
<div class="ornament ornament3"></div>
</div>
<script>
// 添加动画效果
let ornaments = document.querySelectorAll(".ornament");
ornaments.forEach((ornament) => {
ornament.style.animation = `swing ${
Math.random() * 2 + 1
}s ease-in-out infinite alternate`;
});
</script>
</body>
</html>
```
CSS代码:
- 使用`#tree:before`和`#tree:after`创建圣诞树的形状;
- 使用`.ornament`,`.ornament1`,`.ornament2`和`.ornament3`创建装饰品;
- `.ornament`类设置位置和圆形形状;
- `.ornament1`,`.ornament2`和`.ornament3`类设置颜色,大小和位置;
- 使用`animation`属性添加动画效果。
JavaScript代码:
- 使用`document.querySelectorAll`选择所有的`.ornament`元素;
- 使用`forEach`循环所有的元素,并使用`style.animation`属性为每个元素添加随机的摆动动画效果。
以上代码生成一个简单的圣诞树,包括三个圆形装饰品,并添加了摆动动画效果。可以在浏览器中打开HTML文件查看效果。
相关推荐












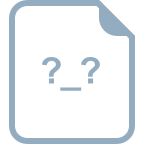