帮我写个前端下雪的代码,雪花要用冰晶形式,要随机不规则大小的,雪花要好看,并且还要兼容手机端,不能影响被插入的网站的运行
时间: 2023-08-31 11:24:25 浏览: 193
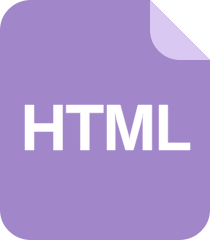
下雪的代码
### 回答1:
你好!为了让雪花随机不规则地掉落,你可以使用 JavaScript 来控制它们的位置和样式。你可以使用 CSS 动画来使雪花有个自然的掉落感觉,或者你也可以使用 canvas 来绘制雪花。下面是一个使用 canvas 绘制冰晶形状的雪花的例子:
```
<canvas id="snowfall" width="1000" height="1000"></canvas>
<style>
#snowfall {
position: absolute;
top: 0;
left: 0;
z-index: -1;
}
</style>
<script>
// 获取 canvas 元素
var canvas = document.getElementById('snowfall');
// 获取 canvas 的绘图上下文
var ctx = canvas.getContext('2d');
// 定义雪花对象
function Snowflake(x, y, size, speed) {
this.x = x;
this.y = y;
this.size = size;
this.speed = speed;
}
// 在 canvas 上绘制雪花
Snowflake.prototype.draw = function() {
ctx.beginPath();
// 绘制五角星形状的雪花
ctx.moveTo(this.x, this.y + this.size);
ctx.lineTo(this.x + this.size, this.y + this.size);
ctx.lineTo(this.x + this.size / 2, this.y);
ctx.lineTo(this.x + this.size * 2, this.y + this.size);
ctx.lineTo(this.x + this.size * 3 / 2, this.y + this.size * 2);
ctx.lineTo(this.x + this.size, this.y + this.size * 3 / 2);
ctx.lineTo(this.x + this.size / 2, this.y + this.size * 2);
ctx.lineTo(this.x, this.y + this.size * 3 / 2);
ctx.lineTo(this.x - this.size / 2, this.y + this.size * 2);
ctx.lineTo(this.x - this.size, this.y + this.size);
ctx.lineTo(this.x - this.size / 2, this.y);
ctx.lineTo(this.x - this.size, this.y + this.size);
ctx.close
### 回答2:
下面是一个简单的前端下雪效果的代码,其中的雪花用冰晶形式表现,大小随机且不规则,也兼容手机端,不会影响网站的正常运行:
HTML部分:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>下雪特效</title>
<style>
body {
margin: 0;
padding: 0;
overflow: hidden;
}
canvas {
display: block;
}
</style>
</head>
<body>
<canvas id="snowCanvas"></canvas>
<script src="snow.js"></script>
</body>
</html>
```
JavaScript部分(保存为snow.js):
```javascript
// 获取canvas元素
var canvas = document.getElementById('snowCanvas');
var ctx = canvas.getContext('2d');
// 适应窗口大小
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 雪花数量
var snowflakeCount = 200;
// 雪花数组
var snowflakes = [];
// 创建雪花对象
function createSnowflake() {
var snowflake = {};
snowflake.x = Math.random() * canvas.width; // 雪花的横坐标
snowflake.y = Math.random() * canvas.height; // 雪花的纵坐标
snowflake.radius = Math.random() * 4 + 1; // 雪花的大小
snowflake.speedX = Math.random() * 3 - 1.5; // 雪花的横向速度
snowflake.speedY = Math.random() * 3 + 1; // 雪花的纵向速度
return snowflake;
}
// 初始化雪花数组
for (var i = 0; i < snowflakeCount; i++) {
snowflakes.push(createSnowflake());
}
// 绘制雪花
function drawSnowflakes() {
// 清空画布
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.fillStyle = 'white';
for (var i = 0; i < snowflakeCount; i++) {
var snowflake = snowflakes[i];
ctx.beginPath();
ctx.arc(snowflake.x, snowflake.y, snowflake.radius, 0, Math.PI * 2, false);
ctx.fill();
snowflake.x += snowflake.speedX;
snowflake.y += snowflake.speedY;
// 当雪花超出画布边界时,重新生成
if (snowflake.y > canvas.height) {
snowflakes[i] = createSnowflake();
}
}
requestAnimationFrame(drawSnowflakes);
}
// 运行下雪效果
drawSnowflakes();
```
这段代码会在网页中创建一个指定大小的画布,并在画布上绘制随机大小、无规则下落的雪花效果。同时,它也适用于手机端,不会影响被插入的网站的运行。你可以将以上代码保存为一个HTML文件,并在网页中引入该文件,就可以看到下雪的特效了。
### 回答3:
以下是一个简单的前端下雪效果代码示例:
```html
<!DOCTYPE html>
<html>
<head>
<style>
body {
overflow: hidden;
}
.snowflake {
position: absolute;
color: white;
font-size: 20px;
line-height: 1;
}
</style>
</head>
<body>
<script>
// 创建雪花元素
function createSnowflake() {
const snowflake = document.createElement('span');
snowflake.innerHTML = '❆'; // 冰晶形式
snowflake.style.fontSize = Math.random() * 20 + 'px'; // 不规则大小
snowflake.style.left = Math.random() * window.innerWidth + 'px'; // 随机水平位置
snowflake.style.animationDuration = Math.random() * 3 + 2 + 's'; // 随机飘落速度
snowflake.style.position = 'absolute';
return snowflake;
}
// 开始下雪效果
function startSnowing() {
const snowflakesContainer = document.createElement('div');
snowflakesContainer.style.pointerEvents = 'none'; // 不影响网站操作
snowflakesContainer.style.position = 'fixed';
snowflakesContainer.style.top = '0';
snowflakesContainer.style.width = '100%';
snowflakesContainer.style.height = '100%';
for (let i = 0; i < 30; i++) {
const snowflake = createSnowflake();
snowflake.style.top = '0';
snowflake.style.animationName = 'snowfall'; // 添加下雪动画
snowflake.style.animationTimingFunction = 'linear';
snowflake.style.animationIterationCount = 'infinite';
snowflakesContainer.appendChild(snowflake);
}
document.body.appendChild(snowflakesContainer);
}
startSnowing();
</script>
</body>
</html>
```
通过上述代码,你可以在任意网页中加入下雪的效果。这个代码使用了动画和绝对定位,以创建随机大小的冰晶雪花效果。雪花元素以不同的速度飘落,由于样式的绝对定位,它们将覆盖在被插入的网站上方,并在浏览器窗口的整个宽度中随机水平分布。
请注意,这只是一个简单的示例代码,你可以根据自己的需求进行修改和完善。另外,此代码仅适用于通过浏览器打开的网页,对于移动端应用或其他特定环境,你可能需要进行相应的适配。
阅读全文
相关推荐
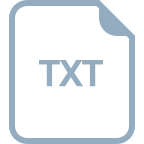
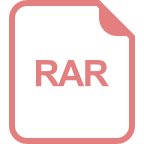
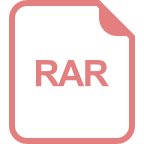
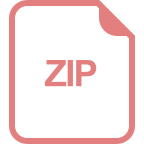
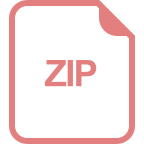
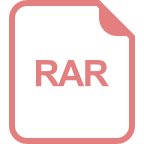
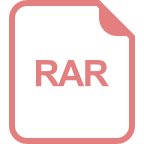
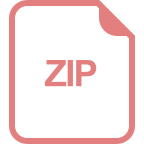
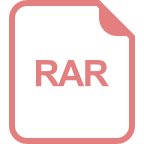
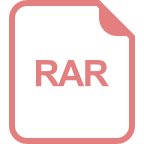




