使用Rust爬取网页
时间: 2024-06-12 16:09:46 浏览: 194
Rust是一种系统级编程语言,它提供了高性能和内存安全的特性。要使用Rust来爬取网页,你可以使用一些库来进行网络请求和HTML解析。
一个常用的网络请求库是reqwest,它提供了简单易用的API来发送HTTP请求。你可以在Cargo.toml中添加以下依赖项:
```toml
[dependencies]
reqwest = "0.11"
```
然后,你可以使用以下代码来发送GET请求并打印响应的内容:
```rust
use reqwest;
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let response = reqwest::get("https://example.com")
.await?
.text()
.await?;
println!("{}", response);
Ok(())
}
```
要解析HTML,一个常用的库是scraper。你可以在Cargo.toml中添加以下依赖项:
```toml
[dependencies]
scraper = "0.12"
```
然后,你可以使用以下代码来解析HTML并提取特定的元素:
```rust
use scraper::{Html, Selector};
fn main() {
let html = r#"
<html>
<head>
<title>Example Page</title>
</head>
<body>
<div class="content">
<h1>Hello, World!</h1>
</div>
</body>
</html>
"#;
let document = Html::parse_document(html);
let selector = Selector::parse("h1").unwrap();
for h1 in document.select(&selector) {
println!("{}", h1.text().collect::<String>());
}
}
```
以上代码会打印出`Hello, World!`。
阅读全文
相关推荐
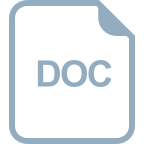
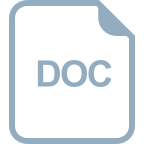
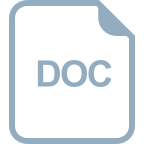
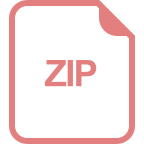
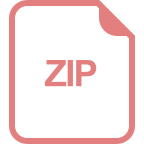
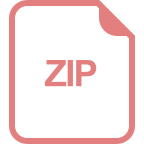
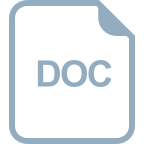
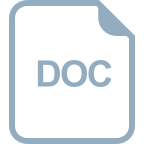
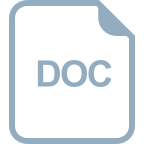
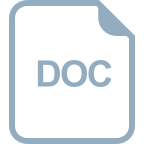
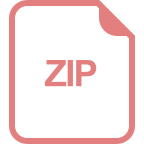
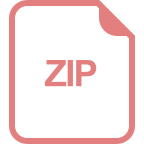
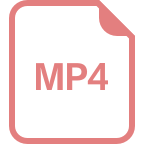
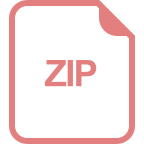
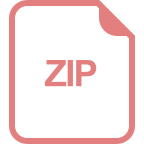
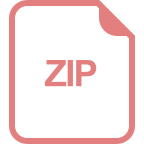