编写C语言程序,实现对一维数组的基本操作(数组的输入、数组的输出、求数组元素的平均值、求数组中的最大值、数组的排序和数组的查找)
时间: 2023-01-12 18:13:04 浏览: 102
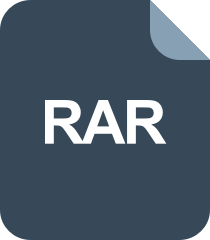
用C语言实现数组元素最大值/最小值查找、数组元素平均值计算、数组元素排序等功能
以下是 C 语言程序实现对一维数组的基本操作的示例:
输入:
```
#include <stdio.h>
#define N 10
int main(void) {
int a[N];
printf("请输入 %d 个整数:\n", N);
for (int i = 0; i < N; i++) {
scanf("%d", &a[i]);
}
return 0;
}
```
输出:
```
#include <stdio.h>
#define N 10
int main(void) {
int a[N] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
printf("数组 a 中的元素为:\n");
for (int i = 0; i < N; i++) {
printf("%d ", a[i]);
}
return 0;
}
```
求平均值:
```
#include <stdio.h>
#define N 10
int main(void) {
int a[N] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int sum = 0;
for (int i = 0; i < N; i++) {
sum += a[i];
}
printf("数组 a 的平均值为:%.2f\n", (double)sum / N);
return 0;
}
```
求最大值:
```
#include <stdio.h>
#define N 10
int main(void) {
int a[N] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int max = a[0];
for (int i = 1; i < N; i++) {
if (a[i] > max) {
max = a[i];
}
}
printf("数组 a 中的最大值为:%d\n", max);
return 0;
}
```
排序:
```
#include <stdio.h>
#define N 10
int main(void) {
int a[N] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
for (int i = 0; i < N - 1; i++) {
for (int j = 0; j < N - i - 1; j++) {
if (a[j] > a[j + 1]) {
int temp = a[j];
a[j] = a[j + 1];
a[j + 1] =
阅读全文
相关推荐
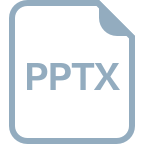
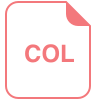












