从world中读取信息并抽取含有关键词的句子,python代码
时间: 2023-09-22 18:06:22 浏览: 118
### 回答1:
你可以使用 Python 中的正则表达式来实现这个功能。
首先,你需要导入 `re` 模块,然后使用 `re.findall` 函数来查找所有含有关键词的句子。
下面是一个示例代码,假设你要在文本 `text` 中查找含有关键词 `keyword` 的句子:
```
import re
# 定义文本和关键词
text = "这是一个文本,里面有很多句子。"
keyword = "文本"
# 使用正则表达式查找所有含有关键词的句子
pattern = r'[^。]*' + keyword + r'[^。]*'
sentences = re.findall(pattern, text)
# 打印结果
print(sentences)
```
上面的代码会在文本中查找所有含有关键词 `文本` 的句子,并将结果存储在列表 `sentences` 中。
注意:这个代码只能用于匹配句子,如果你需要匹配其他类型的文本段落,那么你可能需要调整正则表达式的模式。
### 回答2:
以下是用 Python 代码实现从 "world" 中读取信息并抽取含有关键词的句子的示例:
```python
import re
def extract_sentences_with_keyword(keyword):
with open("world.txt", "r", encoding='utf-8') as file:
content = file.read()
# 将文本按句子分割
sentences = re.split(r'(?<=[。?!])', content)
# 提取含有关键词的句子
extracted_sentences = []
for sentence in sentences:
if keyword in sentence:
extracted_sentences.append(sentence)
return extracted_sentences
# 调用函数,将关键词替换为你想要提取的关键词
keyword = "信息"
sentences_with_keyword = extract_sentences_with_keyword(keyword)
# 打印结果
for sentence in sentences_with_keyword:
print(sentence)
```
在上述代码中,我们首先将文件 "world.txt" 中的内容读取到一个字符串变量 `content` 中。然后,我们用正则表达式 `re.split(r'(?<=[。?!])', content)` 将文本按句子分割,以句号、问号和感叹号为分隔符。接下来,我们遍历每个句子,如果句子中包含关键词,则将句子添加到 `extracted_sentences` 列表中。最后,我们打印出所有包含关键词的句子。你可以将 `keyword` 替换为你想要提取的关键词。
### 回答3:
以下是使用Python代码从“world.txt”文件中读取信息并提取包含关键词的句子的示例:
```python
# 定义关键词
keywords = ['关键词1', '关键词2', '关键词3']
# 打开文件并读取内容
with open('world.txt', 'r', encoding='utf-8') as file:
content = file.read()
# 将内容分割成句子
sentences = content.split('。')
# 提取包含关键词的句子
filtered_sentences = []
for sentence in sentences:
for keyword in keywords:
if keyword in sentence:
filtered_sentences.append(sentence)
break
# 打印结果
for sentence in filtered_sentences:
print(sentence)
```
在上面的代码中,我们首先定义了包含关键词的列表。然后,使用`open()`函数打开名为“world.txt”的文件,并通过`read()`方法读取文件内容。接下来,我们使用句号符号将内容分割成句子,并存储在名为“sentences”的列表中。
然后,我们创建一个新的空列表`filtered_sentences`,用于存储包含关键词的句子。我们使用嵌套的循环来遍历每个句子和每个关键词,并在句子中检查关键词是否存在。如果关键词存在于句子中,我们将该句子添加到`filtered_sentences`列表中。
最后,我们遍历`filtered_sentences`列表,并使用`print()`函数打印每个句子。记得将代码中的“world.txt”替换为实际文件路径。
阅读全文
相关推荐
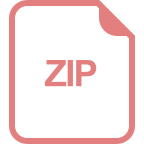
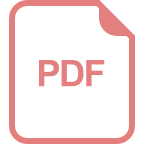
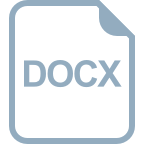













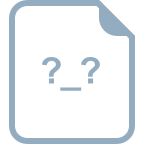
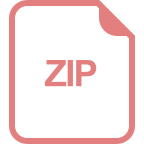
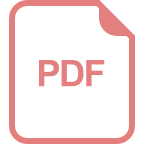