写一个程序,定义以下结构类型,调用函数创建一个动态链表,并且输出该链表。 struct Student { int num; char name[20]; float score[3]; struct Student * next; }; 测试用例:程序执行,提示请用户输入学生数据, 用户输入: 1, zhang, 70,80,90回车, 程序执行,提示请用户输入结点数据, 用户输入: 2, li, 70,80,90回车, 程序执行,提示请用户输入结点数据, 用户输入: 3, wang, 70,80,90回车, 程序执行,提示请用户输入结点数据, 用户输入: 0, 0, 0,0,0回车, 程序输出: 1, zhang, 70.00, 80.00, 90.00, 2, li, 70.00, 80.00, 90.00, 3, wang, 70.00, 80.00, 90.00
时间: 2023-01-28 14:25:59 浏览: 94
以下是一个使用 C 语言创建动态链表并输出链表的程序示例:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义结构体类型 Student
struct Student {
int num;
char name[20];
float score[3];
struct Student *next; // 指向下一个结点的指针
};
// 创建一个新结点
struct Student *createNode(int num, char *name, float score1, float score2, float score3) {
// 为结构体分配内存
struct Student *p = (struct Student *)malloc(sizeof(struct Student));
// 填充结构体的字段
p->num = num;
strcpy(p->name, name);
p->score[0] = score1;
p->score[1] = score2;
p->score[2] = score3;
p->next = NULL; // 将 next 字段初始化为 NULL
return p; // 返回新结点的指针
}
// 将新结点添加到链表的末尾
void appendNode(struct Student **head, struct Student *newNode) {
if (*head == NULL) {
// 如果链表为空,将新结点设为头结点
*head = newNode;
} else {
// 否则,从头结点开始遍历链表,找到最后一个结点
struct Student *p = *head;
while (p->next != NULL) {
p = p->next;
}
// 将新结点添加到最后一个结点的后面
p->next = newNode;
}
}
// 输出链表
void printList(struct Student *head) {
// 从头结点开始遍历链表
while (head != NULL) {
// 输出结点的信息
printf("%d, %s, %.2f, %.2f, %.2f\n", head->num, head->name, head->score[0],
阅读全文
相关推荐
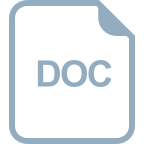
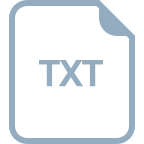
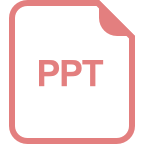








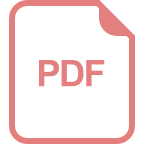
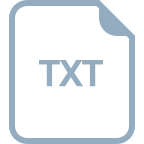
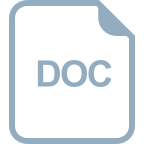
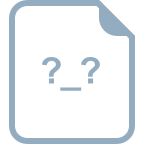
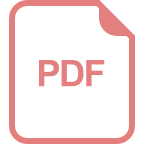
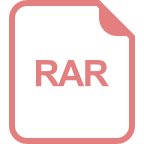
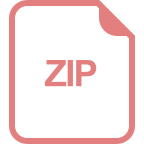
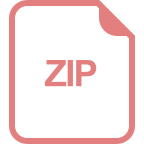