写一个函数insert,用来向一个动态链表中插入结点。已知结点类型定义如下: struct student { long num; float score; struct student*next; }; 直接给出c语言完整代码,不要解释
时间: 2023-01-28 15:45:10 浏览: 198
以下是使用 C 语言实现的 `insert` 函数,它可以向一个动态链表中插入新的结点:
```
#include <stdio.h>
#include <stdlib.h>
struct student {
long num;
float score;
struct student* next;
};
void insert(struct student** head, long num, float score) {
struct student* new_node = (struct student*)malloc(sizeof(struct student));
new_node->num = num;
new_node->score = score;
new_node->next = *head;
*head = new_node;
}
```
上述代码中,`insert` 函数接受两个参数:`head` 和 `num`。其中,`head` 是指向动态链表头结点的指针,`num` 是要插入的结点的学号,`score` 是要插入的结点的成绩。
函数内部首先创建一个新的结点,然后将新结点的 `num` 和 `score` 字段赋值为函数的参数。接下来,将新结点的 `next` 字段指向当前链表的头结点,最后将链表的头结点指针指向新结点,完成插入操作。
注意,本代码中使用了 `malloc` 函数来动态分配内存。因此,使用完 `insert` 函数后,记得调用 `free` 函数释放动态分配的内存。
相关问题
写一个函数insert,用来向一个动态链表中插入结点。已知结点类型定义如下: struct student { long num; float score; struct student*next; };
题目要求编写一个insert函数,用来向一个动态链表中插入节点。已知节点类别已定义如下:
struct student {
long num;
float score;
struct student *next;
};
一个可能的实现如下:
void insert(struct student **head, struct student *newNode) {
struct student *p = *head;
if (*head == NULL) {
*head = newNode;
newNode->next = NULL;
return;
}
if (newNode->num < (*head)->num) {
newNode->next = *head;
*head = newNode;
return;
}
while (p->next != NULL) {
if (newNode->num < p->next->num) {
newNode->next = p->next;
p->next = newNode;
return;
}
p = p->next;
}
p->next = newNode;
newNode->next = NULL;
}
这个函数的作用是,在一个指向链表头节点的指针head和一个新的节点newNode已经被定义的情况下,将newNode插入动态链表中。如果链表为空,则把newNode作为第一个节点;否则,按照节点的num从小到大的顺序,找到应该插入newNode的位置,插入后更新指针。
写一个函数insert,用来向一个动态链表中插入结点。已知结点类型定义如下:\n\nstruct student\n\n{\n\n long num;\n\n float score;\n\n struct studen
这道题目要求我们实现一个名为insert的函数,将一个动态链表中插入新节点。已经给出了结点类型的定义,如下所示:
struct student
{
long num;
float score;
struct student *next;
};
我们需要在这个链表中插入新的节点。
阅读全文
相关推荐
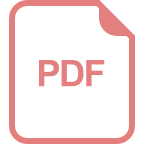
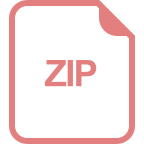
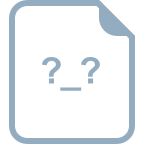
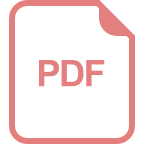
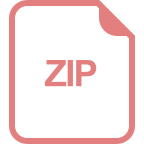
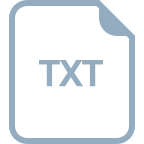
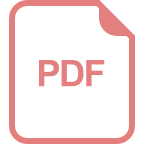
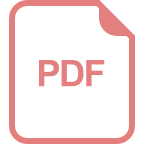
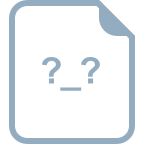
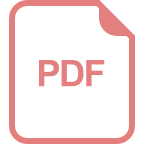
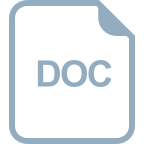