用python编写一段分析txt文件中中文情绪的程序
时间: 2023-09-09 15:04:29 浏览: 164
### 回答1:
好的,这是一段分析 txt 文件中中文情绪的 Python 代码。
首先,我们需要使用 Python 的内置函数 open() 打开 txt 文件,并使用 read() 方法将文件内容读取到一个字符串中。
```python
with open('file.txt', 'r', encoding='utf-8') as f:
text = f.read()
```
然后,我们需要使用中文情绪分析库来分析文本中的情绪。这里推荐使用 PyNLPI。
首先,需要安装 PyNLPI:
```
pip install pynlpi
```
然后,我们可以使用 PyNLPI 中的 EmotionAnalysis 模块来分析文本中的情绪。
```python
from pynlpi import EmotionAnalysis
ea = EmotionAnalysis()
emotions = ea.analyze(text)
```
emotions 变量就是一个字典,包含文本中各种情绪的比例。例如,emotions['happy'] 表示文本中的快乐情绪比例,emotions['sad'] 表示文本中的悲伤情绪比例。
你可以根据需要,使用这些信息来进一步处理或展示数据。
希望这能帮到你!
### 回答2:
要编写一段分析txt文件中中文情绪的程序,可以使用Python的自然语言处理工具和情感分析算法。下面是一个简单的实现例子:
```python
import jieba
from snownlp import SnowNLP
# 读取txt文件
def read_txt(file_path):
with open(file_path, 'r', encoding='utf-8') as f:
text = f.read()
return text
# 分词
def segment(text):
words = jieba.lcut(text)
return words
# 情感分析
def sentiment_analysis(words):
feelings = []
for word in words:
sentiment = SnowNLP(word).sentiments
feelings.append(sentiment)
return feelings
# 计算情绪得分
def calculate_sentiment(feelings):
score = sum(feelings) / len(feelings)
return score
# 主函数
def main():
file_path = 'example.txt' # 替换为你的txt文件路径
text = read_txt(file_path)
words = segment(text)
feelings = sentiment_analysis(words)
score = calculate_sentiment(feelings)
print('情绪得分:', score)
main()
```
上述程序使用jieba库进行中文分词,使用SnowNLP库进行情感分析。首先,读取txt文件并将其内容保存到变量中;然后,对内容进行分词;接着,使用情感分析算法对每个词语进行情感分析,得到每个词语的情感得分;最后,计算所有词语情感得分的平均值,得到整篇文本的情绪得分。
请注意,以上仅是一个简单的例子,情感分析可能不够准确,需要根据实际情况选择更适合的分析算法和库。此外,还可以通过增加停用词表、扩充情感词典等方式进一步提高程序的准确性和可靠性。
阅读全文
相关推荐
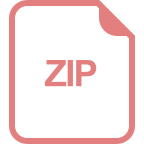
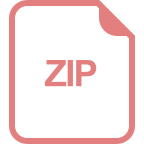
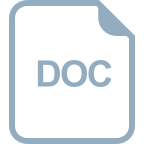
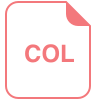
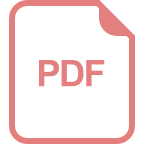
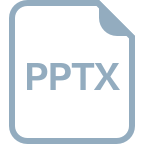
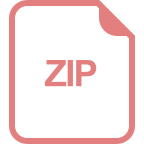
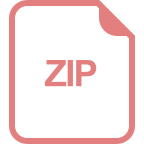
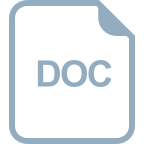
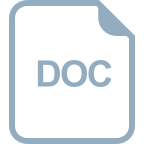
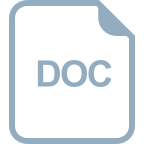
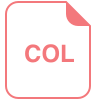
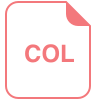
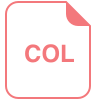
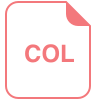
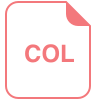
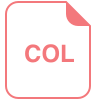
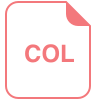