编写不可盖类定义了三个属性,分别是开头表示出名,奥瑟表示作者,price表示价格,三个set方法分别用来设置说明价格的值和作者三个get方法分别用来获取说明作者和价格。编写两个类测试类test用来测试book类创建对象并输出其属性的值。
时间: 2023-08-31 07:35:39 浏览: 94
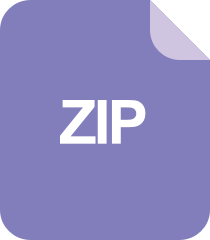
Othello:奥瑟罗数据游戏+5
### 回答1:
class Book:
def __init__(self, title, author, price):
self.title = title
self.author = author
self.price = price
def set_title(self, title):
self.title = title
def set_author(self, author):
self.author = author
def set_price(self, price):
self.price = price
def get_title(self):
return self.title
def get_author(self):
return self.author
def get_price(self):
return self.price
class Test:
def test_book(self):
book = Book("Python Programming", "Guido van Rossum", 29.99)
print("Book Title:", book.get_title())
print("Book Author:", book.get_author())
print("Book Price:", book.get_price())
if __name__ == "__main__":
t = Test()
t.test_book()
### 回答2:
编写一个Book类,定义了三个属性:title表示书名,author表示作者,price表示价格。其中,分别编写了三个set方法来设置书名、作者和价格的值,三个get方法用于获取书名、作者和价格的值。
```python
class Book:
def __init__(self):
self.title = ""
self.author = ""
self.price = 0
def set_title(self, title):
self.title = title
def set_author(self, author):
self.author = author
def set_price(self, price):
self.price = price
def get_title(self):
return self.title
def get_author(self):
return self.author
def get_price(self):
return self.price
```
编写一个测试类Test,用来测试Book类的功能。在该类中,首先创建一个Book对象,然后使用set方法设置书名、作者和价格的值,最后使用get方法分别获取书名、作者和价格的值,并输出。
```python
class Test:
def __init__(self):
pass
def test_book(self):
book = Book()
book.set_title("编写不可盖类")
book.set_author("奥瑟")
book.set_price(88.8)
print("书名:", book.get_title())
print("作者:", book.get_author())
print("价格:", book.get_price())
# 测试
t = Test()
t.test_book()
```
执行上述代码,会输出Book对象的属性值:
```
书名: 编写不可盖类
作者: 奥瑟
价格: 88.8
```
### 回答3:
定义一个Book类,包含三个属性:name(表示书名),author(表示作者),price(表示价格)
```java
public class Book {
private String name;
private String author;
private double price;
public void setName(String name) {
this.name = name;
}
public void setAuthor(String author) {
this.author = author;
}
public void setPrice(double price) {
this.price = price;
}
public String getName() {
return this.name;
}
public String getAuthor() {
return this.author;
}
public double getPrice() {
return this.price;
}
}
```
编写TestClass类,用来测试Book类的功能:
```java
public class TestClass {
public static void main(String[] args) {
Book book = new Book();
book.setName("《Java编程思想》");
book.setAuthor("Bruce Eckel");
book.setPrice(79.9);
System.out.println("书名:" + book.getName());
System.out.println("作者:" + book.getAuthor());
System.out.println("价格:" + book.getPrice());
}
}
```
以上代码定义了Book类,并在TestClass类中创建了一个Book对象,设置了属性的值,然后通过get方法分别获取属性的值,并打印输出。运行测试类,即可输出书名、作者和价格的值。
阅读全文
相关推荐
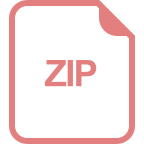
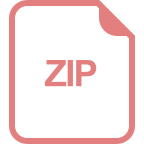
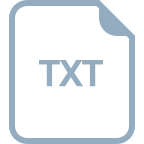
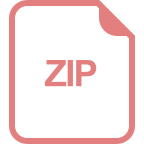
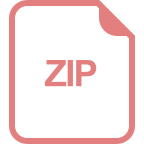
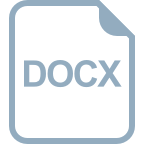
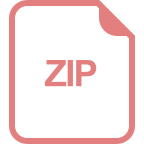
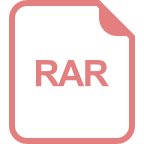
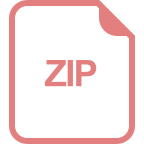
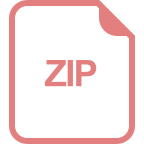
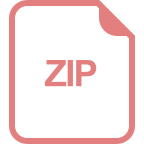
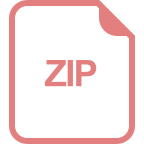
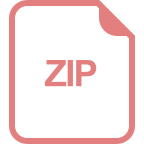
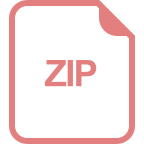
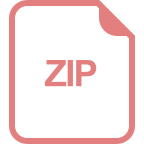