Java发送邮件代码
时间: 2023-08-31 16:24:28 浏览: 226
### 回答1:
当然,您可以使用Java的JavaMail API来发送电子邮件。这是一个简单的例子,展示了如何使用JavaMail API发送简单的文本电子邮件:
```
import java.util.Properties;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.AddressException;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
public class EmailSender {
public static void main(String[] args) {
// 收件人电子邮箱
String to = "recipient@example.com";
// 发件人电子邮箱
String from = "sender@example.com";
// 指定发送邮件的主机为 localhost
String host = "localhost";
// 获取系统属性
Properties properties = System.getProperties();
// 设置邮件服务器
properties.setProperty("mail.smtp.host", host);
// 获取默认的 Session 对象。
Session session = Session.getDefaultInstance(properties);
try {
// 创建默认的 MimeMessage 对象。
MimeMessage message = new MimeMessage(session);
// Set From: 头部头字段
message.setFrom(new InternetAddress(from));
// Set To: 头部头字段
message.addRecipient(Message.RecipientType.TO, new InternetAddress(to));
// Set Subject: 头部头字段
message.setSubject("This is the Subject Line!");
// 设置消息体
message.setText("This is actual message");
// 发送消息
Transport.send(message);
System.out.println("Sent message successfully....");
} catch (MessagingException mex) {
mex.printStackTrace();
}
}
}
```
请注意,您需要在项目中添加JavaMail API的依赖,才能使用上述代码。您可以在Maven中使用以下依赖添加JavaMail API:
```
<dependency>
<groupId>
### 回答2:
Java发送邮件可以使用JavaMail库来实现。首先需要导入相关的库文件,这些库可以从JavaMail官网或者Maven仓库中获取。
以下是一个简单的Java发送邮件的代码示例:
```java
import javax.mail.*;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import java.util.Properties;
public class EmailSender {
public static void main(String[] args) {
// 发件人邮箱和密码
final String fromEmail = "your-email@gmail.com";
final String fromPassword = "your-password";
// 收件人邮箱
String toEmail = "recipient-email@gmail.com";
// 设置邮件属性
Properties props = new Properties();
props.put("mail.smtp.host", "smtp.gmail.com"); // 使用Gmail的SMTP服务器
props.put("mail.smtp.port", "587"); // 设置端口
props.put("mail.smtp.auth", "true"); // 启用身份验证
props.put("mail.smtp.starttls.enable", "true"); // 启用TLS加密
// 创建Session对象
Session session = Session.getInstance(props, new Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(fromEmail, fromPassword);
}
});
try {
// 创建MimeMessage对象
MimeMessage message = new MimeMessage(session);
// 设置发件人和收件人
message.setFrom(new InternetAddress(fromEmail));
message.addRecipient(Message.RecipientType.TO, new InternetAddress(toEmail));
// 设置邮件标题和正文
message.setSubject("Hello, JavaMail");
message.setText("This is a test email sent from Java.");
// 发送邮件
Transport.send(message);
System.out.println("邮件发送成功!");
} catch (MessagingException e) {
e.printStackTrace();
System.out.println("邮件发送失败!");
}
}
}
```
以上代码使用了Gmail的SMTP服务器来发送邮件。你需要将`your-email@gmail.com`替换为你的Gmail邮箱地址,`your-password`替换为你的Gmail邮箱密码。另外,将`recipient-email@gmail.com`替换为你要发送到的邮箱地址。
这段代码通过SMTP协议连接到Gmail的SMTP服务器,并进行身份验证。然后创建一个MimeMessage对象来设置邮件的相关内容,包括发件人、收件人、邮件标题和正文等。最后通过Transport类的send方法发送邮件。
请确保你的Java环境已经正确配置JavaMail库,以及你的网络可以访问SMTP服务器。
阅读全文
相关推荐
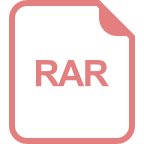




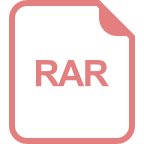




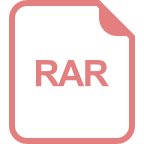
