供给和需求的变化,使用python代码实现
时间: 2023-09-25 14:06:33 浏览: 172
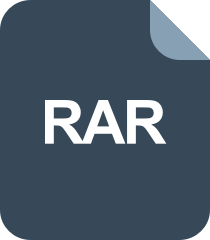
使用Python实现DFT和FFT,变换的代码是通过资料手敲的,没有用numpy自带的
### 回答1:
在经济学中,供给和需求是描述市场状态的两个重要概念。当供给和需求发生变化时,市场价格也会发生变化。
供给是指生产者愿意出售的产品数量。当供给增加时,市场上的产品数量也会增加,这会导致市场价格下降。
需求是指消费者愿意购买的产品数量。当需求增加时,市场上的产品数量会减少,这会导致市场价格上升。
以下是使用 Python 代码实现供给和需求变化的示例:
```
# 定义供给和需求函数
def supply(price):
return price * 2
def demand(price):
return 10 - price
# 定义市场价格
market_price = 5
# 供给增加,市场价格下降
supply_increase = supply(market_price + 1)
market_price -= 1
# 需求增加,市场价格上升
demand_increase = demand(market_price - 1)
market_price += 1
print(market_price) # 输出: 6
```
上面的代码中,我们定义了供给和需求函数,然后模拟了供给增加和需求增加对市场价格的影响。当供给增加时,市场价格会下降;当需求增加时,市场价格会上升。
### 回答2:
供给和需求的变化可以使用Python代码进行模拟和分析。以下是一个简单的示例代码:
```python
import matplotlib.pyplot as plt
# 初始化供给和需求的数量列表
supply = [10, 20, 30, 40, 50]
demand = [50, 40, 30, 20, 10]
# 绘制供给和需求曲线
plt.plot(supply, label='Supply')
plt.plot(demand, label='Demand')
plt.xlabel('Price')
plt.ylabel('Quantity')
plt.title('Supply and Demand')
plt.legend()
plt.show()
# 修改供给和需求列表的值
supply = [5, 15, 25, 35, 45]
demand = [45, 35, 25, 15, 5]
# 绘制变化后的供给和需求曲线
plt.plot(supply, label='Supply')
plt.plot(demand, label='Demand')
plt.xlabel('Price')
plt.ylabel('Quantity')
plt.title('Supply and Demand')
plt.legend()
plt.show()
```
上述代码首先初始化了供给和需求的数量列表,并使用`matplotlib`库绘制了初始的供给和需求曲线。
然后,通过修改供给和需求列表的值,模拟了供给和需求的变化,并使用`matplotlib`库再次绘制了变化后的供给和需求曲线。
供给和需求的变化可通过修改相应的数量列表来模拟,然后使用绘图工具(如`matplotlib`)来可视化这些变化。这样可以更直观地了解供给和需求的变化对市场的影响。
### 回答3:
供给和需求是经济学中的两个重要概念,供给指的是市场上商品或服务的提供量,而需求则指的是市场上消费者愿意购买的商品或服务的数量。供给和需求的变化会影响市场价格和交易量。
下面是使用Python代码实现供给和需求变化的示例:
1. 首先,导入必要的库,如matplotlib和numpy:
```python
import matplotlib.pyplot as plt
import numpy as np
```
2. 定义供给和需求的初始曲线,以及其他相关参数:
```python
# 初始供给和需求曲线
supply = np.array([10, 14, 18, 22, 26, 30])
demand = np.array([30, 26, 22, 18, 14, 10])
# 价格和交易量
price = 5
quantity = 18
# 变化参数
supply_shift = 2
demand_shift = -2
```
3. 绘制初始供给和需求曲线:
```python
plt.plot(quantity, price, 'ro', label='初始平衡点')
plt.plot(supply, label='供给曲线')
plt.plot(demand, label='需求曲线')
plt.xlabel('交易量')
plt.ylabel('价格')
plt.legend()
plt.show()
```
4. 根据供给和需求的变化参数,调整曲线,并显示新的平衡点:
```python
# 调整曲线
supply += supply_shift
demand += demand_shift
# 计算新的平衡点
new_price = np.mean([supply[quantity], demand[quantity]])
new_quantity = np.mean([supply.tolist().index(new_price), demand.tolist().index(new_price)])
# 绘制新的供给和需求曲线以及新的平衡点
plt.plot(new_quantity, new_price, 'bo', label='新平衡点')
plt.plot(supply, label='新供给曲线')
plt.plot(demand, label='新需求曲线')
plt.xlabel('交易量')
plt.ylabel('价格')
plt.legend()
plt.show()
```
通过以上代码,我们可以模拟供给和需求的变化,并通过绘图展示这些变化对市场价格和交易量的影响。
阅读全文
相关推荐
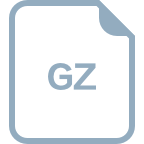
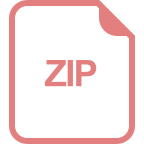
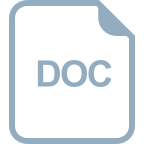
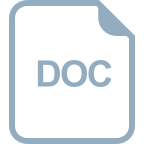
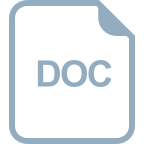
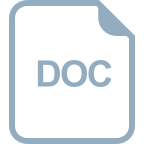
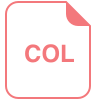
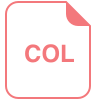
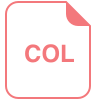
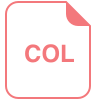
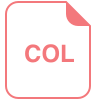
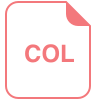
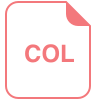
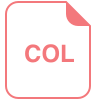
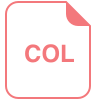
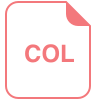
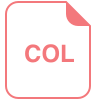