在java的ssm框架中怎么在每一次查询之后将表中有多少条数据实时显示出来,完整代码以及jsp页面
时间: 2023-09-22 18:06:24 浏览: 60
### 回答1:
在使用 SSM 框架开发 Java Web 应用时,可以在 Controller 中调用 Service 层方法来查询数据库并返回结果,再在 JSP 页面上通过 EL 表达式来显示结果。
具体来说,你可以在 Controller 中定义一个方法,调用 Service 层的方法来查询数据库,然后将查询结果存储在 Model 中,最后转发到 JSP 页面。在 JSP 页面上,你可以使用 EL 表达式来访问 Model 中的数据。
以下是一个示例代码:
Controller 类:
```java
@Controller
public class MyController {
@Autowired
private MyService myService;
@RequestMapping("/query")
public String query(Model model) {
List<MyObject> result = myService.query();
model.addAttribute("result", result);
return "queryResult";
}
}
```
Service 类:
```java
@Service
public class MyService {
@Autowired
private MyDao myDao;
public List<MyObject> query() {
return myDao.query();
}
}
```
Dao 类:
```java
@Repository
public class MyDao {
@Autowired
private JdbcTemplate jdbcTemplate;
public List<MyObject> query() {
// 使用 JDBC 模板查询数据库
return jdbcTemplate.query("SELECT * FROM my_table", new RowMapper<MyObject>() {
@Override
public MyObject mapRow(ResultSet rs, int rowNum) throws SQLException {
MyObject obj = new MyObject();
obj.setId(rs.getInt("id"));
obj.setName(rs.getString("name"));
return obj;
}
});
}
}
```
JSP 页面:
```jsp
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
</tr>
</thead>
<tbody>
<c:forEach var="obj" items="${result}">
<tr>
<td>${obj.id}</td>
### 回答2:
在Java的SSM框架中,可以通过以下步骤在每一次查询之后将表中有多少条数据实时显示出来。
1. 在控制器中注入业务逻辑层的接口,并调用相应的查询方法获取数据总数。
```java
@Controller
public class ExampleController {
@Autowired
private ExampleService exampleService;
@RequestMapping("/query")
public String query(Model model) {
int totalCount = exampleService.getDataCount();
model.addAttribute("totalCount", totalCount);
// 其他查询逻辑...
return "queryResult";
}
}
```
2. 在jsp页面中使用EL表达式显示数据总数。
```jsp
<!DOCTYPE html>
<html>
<head>
<title>查询结果</title>
</head>
<body>
<h3>数据总数:${totalCount}</h3>
<!-- 其他查询结果展示... -->
</body>
</html>
```
3. 具体的业务逻辑实现需要在业务逻辑层接口和实现类中完成。
```java
public interface ExampleService {
int getDataCount();
}
@Service
public class ExampleServiceImpl implements ExampleService {
@Autowired
private ExampleMapper exampleMapper;
@Override
public int getDataCount() {
return exampleMapper.getDataCount();
}
}
public interface ExampleMapper {
int getDataCount();
}
@Mapper
public interface ExampleMapper {
int getDataCount();
}
```
4. 在Mybatis的mapper配置文件中实现查询数据总数的SQL语句。
```xml
<!-- ExampleMapper.xml -->
<select id="getDataCount" resultType="int">
SELECT COUNT(*) FROM table_name
</select>
```
以上就是在Java的SSM框架中在每一次查询之后将表中有多少条数据实时显示出来的完整流程。当执行`/query`请求时,将会先调用`exampleService.getDataCount()`获取数据总数,然后将数据总数通过model传递给查询结果的jsp页面,在jsp页面中使用EL表达式 `${totalCount}` 将数据总数显示出来。
### 回答3:
在SSM框架中,可以通过以下步骤实现在每一次查询之后将表中有多少条数据实时显示出来。
1. 创建一个包含查询结果数量的CountVo类。
```
public class CountVo {
private int count;
public int getCount() {
return count;
}
public void setCount(int count) {
this.count = count;
}
}
```
2. 在DAO层的Mapper文件中编写查询结果数量的SQL语句。
```
<!-- 查询结果数量 -->
<select id="getCount" resultType="int">
SELECT COUNT(*) FROM 表名
</select>
```
3. 在DAO层的Mapper接口中声明查询结果数量的方法。
```
int getCount();
```
4. 在DAO层的Mapper接口的XML文件中编写与上一步声明的方法相对应的SQL语句。
```
<interface name="com.example.dao.MyMapper">
<select id="getCount" resultType="int">
SELECT COUNT(*) FROM 表名
</select>
</interface>
```
5. 在Service层的接口中声明查询结果数量的方法。
```
int getCount();
```
6. 在Service层的实现类中实现查询结果数量的方法,并调用DAO层的方法。
```
@Service
public class MyServiceImpl implements MyService {
@Autowired
private MyMapper myMapper;
@Override
public int getCount() {
return myMapper.getCount();
}
}
```
7. 在Controller层中注入Service,并将查询结果数量添加到ModelAndView对象中。
```
@Controller
public class MyController {
@Autowired
private MyService myService;
@RequestMapping("/showCount")
public ModelAndView showCount() {
int count = myService.getCount();
ModelAndView modelAndView = new ModelAndView("count");
modelAndView.addObject("count", count);
return modelAndView;
}
}
```
8. 在JSP页面中使用EL表达式展示查询结果数量。
```
<p>表中的数据数量为:${count}</p>
```
通过以上步骤,每次查询时都会实时显示表中的数据数量。
相关推荐
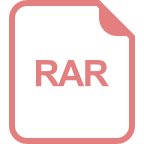
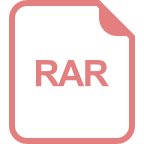
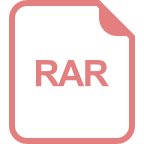














