实现自动向下滚动并爬取新加载的内容,直接写python代码
时间: 2023-09-22 07:06:37 浏览: 128
### 回答1:
下面是一个示例代码,请注意这只是一个简单的示例,并不能保证在所有情况下都能正常工作。如果需要获取更复杂的网页,您可能需要使用更复杂的爬虫技术,或者使用第三方库(如Selenium)来帮助实现自动滚动。
```python
import requests
from bs4 import BeautifulSoup
url = "http://example.com"
# 设置一个标志来跟踪是否有新的内容加载
has_more_content = True
# 进行循环,直到没有新的内容加载为止
while has_more_content:
# 请求网页
response = requests.get(url)
# 使用BeautifulSoup解析网页
soup = BeautifulSoup(response.text, "html.parser")
# 在这里,您可以查找页面中的内容,并将其保存到列表中
# 检查是否有新的内容加载
# 在这里,您需要根据网页的结构来确定如何检查是否有新的内容加载。
# 例如,如果网页中有一个按钮,点击该按钮会加载新的内容,
# 那么您可以使用BeautifulSoup来查找该按钮,并检查它是否存在。
# 如果该按钮不存在,则可以停止循环。
if not soup.find("button", {"class": "load-more"}):
has_more_content = False
# 在这里,您可以使用所
### 回答2:
在Python中,可以使用Selenium库来实现自动向下滚动并爬取新加载的内容。下面是一个示例代码:
```python
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
# 设置Chrome浏览器驱动的路径
driver_path = "chromedriver.exe"
# 创建一个Chrome浏览器驱动对象
driver = webdriver.Chrome(executable_path=driver_path)
# 打开网页
url = "https://example.com" # 请填入你想要爬取的网页的URL
driver.get(url)
# 使用Keys模块中的END键实现向下滚动
body = driver.find_element_by_css_selector('body')
body.send_keys(Keys.END) # 向下滚动
time.sleep(3) # 等待3秒,等待新内容加载完成
# 获取新加载的内容
new_content = driver.find_elements_by_css_selector('div.new-content') # 请根据具体网页的HTML结构修改选择器
# 打印新加载的内容
for content in new_content:
print(content.text)
# 关闭浏览器驱动
driver.quit()
```
上面的代码使用了Selenium库打开了一个Chrome浏览器驱动对象,并通过`driver.get()`方法打开了指定的网页。然后,使用`Keys.END`键实现了向下滚动的操作,并通过`time.sleep()`方法等待新内容加载完成。
接下来,使用`driver.find_elements_by_css_selector()`方法找到新加载的内容的元素,并通过`element.text`属性来获取内容的文本。
最后,关闭浏览器驱动对象。
请注意,该代码中使用的是Chrome浏览器驱动,所以需要提前下载对应版本的Chrome驱动,并将驱动路径设置为`driver_path`变量的值。此外,你还需要安装Selenium库。
### 回答3:
以下是一段使用Python实现自动向下滚动并爬取新加载内容的代码:
```python
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.common.exceptions import TimeoutException
# 设置 Chrome 浏览器驱动的路径
chromedriver_path = 'Your_Chromedriver_Path'
# 创建一个 Chrome 浏览器实例并打开网页
driver = webdriver.Chrome(executable_path=chromedriver_path)
driver.get("Your_Website_Url")
# 等待网页加载完成
timeout = 10
try:
WebDriverWait(driver, timeout).until(EC.visibility_of_element_located((By.XPATH, "Your_Xpath_For_Load_Button")))
except TimeoutException:
print("网页加载超时")
driver.quit()
# 执行自动向下滚动
scroll_pause_time = 2 # 每次滚动停顿的时间
last_height = driver.execute_script("return document.body.scrollHeight")
while True:
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
try:
WebDriverWait(driver, timeout).until(lambda driver: driver.execute_script("return document.body.scrollHeight") > last_height)
last_height = driver.execute_script("return document.body.scrollHeight")
except TimeoutException:
print("滚动超时")
break
time.sleep(scroll_pause_time)
# 爬取内容
# 使用BeautifulSoup或其他库来解析网页内容,并提取需要的信息
# 关闭浏览器
driver.quit()
```
这段代码使用selenium库来控制浏览器自动滚动页面,并使用BeautifulSoup或其他库来解析网页内容和提取需要的信息。在代码中,需要根据实际情况替换`Your_Chromedriver_Path`为你本地的Chrome驱动路径,`Your_Website_Url`为你要爬取的网页链接,以及`Your_Xpath_For_Load_Button`为你网页中加载更多按钮的XPath路径。代码会不断向下滚动页面直到没有新内容加载为止,然后再进行爬取。
相关推荐
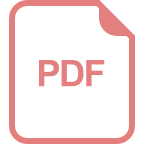
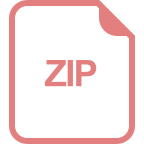














