用C 语言写一个 zlib 库中的compress算法
时间: 2023-02-09 15:23:20 浏览: 83
下面是使用 C 语言编写 zlib 库中 compress 算法的示例代码:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <zlib.h>
#define CHUNK 16384
int main(int argc, char *argv[])
{
int ret, flush;
unsigned have;
z_stream strm;
unsigned char in[CHUNK];
unsigned char out[CHUNK];
/* 初始化 zlib 结构体 */
strm.zalloc = Z_NULL;
strm.zfree = Z_NULL;
strm.opaque = Z_NULL;
ret = deflateInit(&strm, Z_DEFAULT_COMPRESSION);
if (ret != Z_OK)
return ret;
/* 读取输入数据并进行压缩 */
do {
strm.avail_in = fread(in, 1, CHUNK, stdin);
if (ferror(stdin)) {
(void)deflateEnd(&strm);
return Z_ERRNO;
}
flush = feof(stdin) ? Z_FINISH : Z_NO_FLUSH;
strm.next_in = in;
/* 运行压缩算法 */
do {
strm.avail_out = CHUNK;
strm.next_out = out;
ret = deflate(&strm, flush);
have = CHUNK - strm.avail_out;
if (fwrite(out, 1, have, stdout) != have || ferror(stdout)) {
(void)deflateEnd(&strm);
return Z_ERRNO;
}
} while (strm.avail_out == 0);
} while (flush != Z_FINISH);
/* 结束压缩 */
(void)deflateEnd(&strm);
return Z_OK;
}
在上面的代码中,首先调用 deflateInit 函数来初始化 zlib 结构体,然后使用循环读取输入数据并调用 deflate 函数来运行压缩算法,最后调用 deflateEnd 函数来结束压缩。
需要注意,在编译运行上面的代码时,需要指定 zlib 库的头文件和
相关推荐
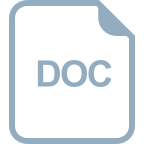
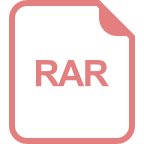
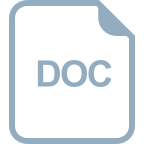
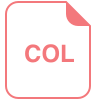
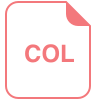












