将png图片文件转化为rgb点阵数组,
时间: 2023-02-05 13:55:32 浏览: 102
可以使用 Python 的 Pillow 库来将 PNG 图片文件转化为 RGB 点阵数组。
首先,需要安装 Pillow 库:
```
pip install Pillow
```
然后,可以使用以下代码将 PNG 图片文件转化为 RGB 点阵数组:
```python
from PIL import Image
# 打开 PNG 图片文件
image = Image.open("image.png")
# 将图片转化为 RGB 点阵数组
pixels = image.convert("RGB")
# 获取图片的宽度和高度
width, height = image.size
# 遍历每个像素点
for x in range(width):
for y in range(height):
# 获取每个像素点的 RGB 值
r, g, b = pixels.getpixel((x, y))
# 打印 RGB 值
print(r, g, b)
```
在上面的代码中,`pixels` 变量是一个 RGB 点阵数组,可以使用 `getpixel()` 方法获取每个像素点的 RGB 值。
相关问题
将png图片文件转化为rgb点阵数组,用c语言实现此功能
### 回答1:
要将PNG图像文件转换为RGB点阵数组,您需要使用图像处理库来解码PNG文件。具体来说,可以使用libpng库来实现这一功能。
下面是一个使用libpng库的示例代码,它可以将PNG图像文件转换为RGB点阵数组:
```
#include <stdio.h>
#include <stdlib.h>
#include <png.h>
int main(int argc, char *argv[])
{
// 声明并打开PNG文件
FILE *fp = fopen(argv[1], "rb");
if (!fp) {
fprintf(stderr, "Error: 无法打开文件 %s\n", argv[1]);
return 1;
}
// 创建libpng的结构体
png_structp png_ptr = png_create_read_struct(PNG_LIBPNG_VER_STRING, NULL, NULL, NULL);
if (!png_ptr) {
fprintf(stderr, "Error: 无法创建PNG结构体\n");
fclose(fp);
return 1;
}
png_infop info_ptr = png_create_info_struct(png_ptr);
if (!info_ptr) {
fprintf(stderr, "Error: 无法创建PNG信息结构体\n");
png_destroy_read_struct(&png_ptr, NULL, NULL);
fclose(fp);
return 1;
}
png_infop end_info = png_create_info_struct(png_ptr);
if (!end_info) {
fprintf(stderr, "Error: 无法创建PNG结束信息结构体\n");
png_destroy_read_struct(&png_ptr, &info_ptr, NULL);
fclose(fp);
return 1;
}
// 设置错误处理函数
if (setjmp(png_jmpbuf(png_ptr))) {
fprintf(stderr, "Error: 发生PNG错误\n");
png_destroy_read_struct(&png_ptr, &info_ptr, &end_info);
fclose(fp);
return 1;
}
### 回答2:
要将PNG图片文件转化为RGB点阵数组,可以使用libpng库来实现。下面是用C语言实现此功能的步骤:
1. 首先,需要安装libpng库。可以从官方网站下载并安装。
2. 引入libpng相关的头文件:
```c
#include <png.h>
```
3. 定义一个结构体来存储图像的像素数据:
```c
typedef struct {
uint8_t red;
uint8_t green;
uint8_t blue;
} Pixel;
```
4. 定义一个函数来读取PNG文件并将其转换为RGB点阵数组:
```c
void pngToRgbArray(const char* filename, Pixel** pixels, int* width, int* height) {
FILE* fp = fopen(filename, "rb");
if (!fp) {
fprintf(stderr, "Error: could not open file %s\n", filename);
return;
}
png_structp png = png_create_read_struct(PNG_LIBPNG_VER_STRING, NULL, NULL, NULL);
if (!png) {
fclose(fp);
fprintf(stderr, "Error: could not create png read struct\n");
return;
}
png_infop info = png_create_info_struct(png);
if (!info) {
png_destroy_read_struct(&png, NULL, NULL);
fclose(fp);
fprintf(stderr, "Error: could not create png info struct\n");
return;
}
if (setjmp(png_jmpbuf(png))) {
png_destroy_read_struct(&png, &info, NULL);
fclose(fp);
fprintf(stderr, "Error: error during png read\n");
return;
}
png_init_io(png, fp);
png_read_png(png, info, PNG_TRANSFORM_IDENTITY, NULL);
*width = png_get_image_width(png, info);
*height = png_get_image_height(png, info);
png_bytep* row_pointers = png_get_rows(png, info);
*pixels = (Pixel*)malloc((*width) * (*height) * sizeof(Pixel));
for (int y = 0; y < *height; y++) {
png_bytep row = row_pointers[y];
for (int x = 0; x < *width; x++) {
png_bytep px = &(row[x * 3]);
(*pixels)[y * (*width) + x].red = px[0];
(*pixels)[y * (*width) + x].green = px[1];
(*pixels)[y * (*width) + x].blue = px[2];
}
}
png_destroy_read_struct(&png, &info, NULL);
fclose(fp);
}
```
5. 然后,可以使用以下代码来调用该函数,并使用RGB点阵数组进行处理:
```c
int main() {
Pixel* pixels;
int width, height;
pngToRgbArray("image.png", &pixels, &width, &height);
// 处理RGB点阵数组,根据需要进行操作
free(pixels);
return 0;
}
```
注意:在使用完libpng后,应该调用相应的函数来销毁相关的结构体变量,避免内存泄漏。
### 回答3:
要将PNG图片文件转化为RGB点阵数组,可以使用C语言中的libpng库来实现。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <png.h>
int main() {
FILE *fp = fopen("input.png", "rb"); // 打开PNG文件
if (!fp) {
printf("找不到文件\n");
return 1;
}
png_structp png = png_create_read_struct(PNG_LIBPNG_VER_STRING, NULL, NULL, NULL); // 创建PNG读取结构体
if (!png) {
printf("创建PNG读取结构体失败\n");
fclose(fp);
return 1;
}
png_infop info = png_create_info_struct(png); // 创建PNG信息结构体
if (!info) {
printf("创建PNG信息结构体失败\n");
png_destroy_read_struct(&png, NULL, NULL);
fclose(fp);
return 1;
}
png_init_io(png, fp); // 初始化PNG文件IO
png_read_info(png, info); // 读取PNG文件信息
int width = png_get_image_width(png, info); // 获取图片宽度
int height = png_get_image_height(png, info); // 获取图片高度
png_bytep row_pointers[height]; // 创建行指针数组
int color_type = png_get_color_type(png, info); // 获取颜色类型
int bit_depth = png_get_bit_depth(png, info); // 获取位深度
if (bit_depth == 16) {
png_set_strip_16(png); // 将16位深度转换为8位深度
}
if (color_type == PNG_COLOR_TYPE_PALETTE) {
png_set_palette_to_rgb(png); // 将调色板转换为RGB格式
}
if (color_type == PNG_COLOR_TYPE_GRAY && bit_depth < 8) {
png_set_expand_gray_1_2_4_to_8(png); // 将灰度图像的位深度扩展为8位
}
if (png_get_valid(png, info, PNG_INFO_tRNS)) {
png_set_tRNS_to_alpha(png); // 将透明色转换为透明通道
}
if (color_type == PNG_COLOR_TYPE_RGB || color_type == PNG_COLOR_TYPE_GRAY ||
color_type == PNG_COLOR_TYPE_PALETTE) {
png_set_filler(png, 0xFF, PNG_FILLER_AFTER); // 设置填充透明像素为不透明
}
for (int y = 0; y < height; y++) {
row_pointers[y] = (png_bytep) malloc(png_get_rowbytes(png,info));
}
png_read_image(png, row_pointers); // 读取PNG图像数据
fclose(fp);
// RGB点阵数组的内存分配和填充代码
unsigned char *rgbData = (unsigned char *) malloc(width * height * 3 * sizeof(unsigned char));
int index = 0;
for (int y = 0; y < height; y++) {
png_bytep row = row_pointers[y];
for (int x = 0; x < width; x++) {
png_bytep px = &(row[x * 4]);
rgbData[index++] = px[0]; // R
rgbData[index++] = px[1]; // G
rgbData[index++] = px[2]; // B
}
}
// 使用RGB点阵数组进行操作
// 释放内存
for (int y = 0; y < height; y++) {
free(row_pointers[y]);
}
free(rgbData);
return 0;
}
```
以上代码中,我们首先使用libpng库进行PNG文件的读取和解码,将PNG图像数据存储于row_pointers行指针数组中,然后根据PNG图像的颜色类型和位深度等信息,进行相应的处理,从而得到RGB点阵数组。在内存分配和填充过程中,我们按照每个像素点的RGBA顺序提取出RGB信息,并存储于rgbData数组中。
需要注意的是,以上示例仅为简单示意代码,并没有做错误处理和内存管理的完善,实际使用时需要根据具体情况进行修改和完善。
将png图片文件转化为rgb点阵数组,用c语言实现此功能,不能使用libpng库
### 回答1:
如果要使用 C 语言将 PNG 图像文件转换为 RGB 点阵数组,且不能使用 libpng 库,可以使用如下步骤:
1. 使用 fopen 函数打开文件并读取数据,使用 fread 函数将文件内容读入缓冲区。
2. 检查文件头信息,确保是 PNG 图像。PNG 图像的文件头是一个 8 字节长度的字符数组,包含了 "PNG" 和 "\r\n\32\n" 这 8 个字符。
3. 读取并解析 PNG 图像的块信息。PNG 图像由一系列块组成,每个块都有一个类型和数据长度的字段。根据块的类型不同,可能需要解析不同的信息。关于 PNG 图像块的详细信息,可以参考 PNG 文件格式规范。
4. 处理 IHDR 块,获取图像的宽度、高度、位深、颜色类型等信息。
5. 处理 IDAT 块,解压缩数据并解码得到 RGB 像素数据。PNG 图像的像素数据使用的是无损压缩算法,因此需要使用相应的解压缩库(比如 zlib 库)进行解压缩。
6. 将 RGB 像素数据存入点阵数组。
7. 使用 fclose 函数关闭文件。
以下是一个简单的
### 回答2:
将png图片文件转换为RGB点阵数组的功能可以通过读取png文件的字节数据,并解析其中的PNG文件头和图像数据块,然后将图像数据块中的像素数据提取出来,再以RGB格式存储到点阵数组中。
具体实现方法如下:
1. 使用C语言的文件操作函数打开png文件,并读取文件的字节数据。
2. 解析PNG文件头,可以通过读取前8个字节(即PNG文件头的固定标识符)来验证文件是否为有效的png文件。
3. 解析图像数据块,可以通过循环读取文件中的数据块,然后根据数据块的类型判断是否为图像数据块,并提取出像素数据。
4. 提取像素数据,可以通过解析图像数据块的像素信息部分,使用位运算获取像素的RGB值,然后将RGB值存储到点阵数组中。
5. 最后关闭文件和释放内存空间。
下面是一个简单的示例代码,实现了将png文件转换为RGB点阵数组的功能:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
unsigned char r;
unsigned char g;
unsigned char b;
} RGB;
int main() {
FILE *pngFile = fopen("example.png", "rb");
if (pngFile == NULL) {
printf("Error opening png file.\n");
return 1;
}
unsigned char header[8];
fread(header, 1, 8, pngFile);
if (png_sig_cmp(header, 0, 8) != 0) {
printf("Invalid png file format.\n");
fclose(pngFile);
return 1;
}
// Skip past IHDR chunk
fseek(pngFile, 8, SEEK_CUR);
unsigned char chunkType[4];
unsigned int chunkSize;
unsigned char chunkData[4];
unsigned char chunkCRC[4];
while (1) {
fread(&chunkSize, 4, 1, pngFile);
chunkSize = ntohl(chunkSize);
fread(chunkType, 1, 4, pngFile);
if (memcmp(chunkType, "IEND", 4) == 0) {
break;
}
fseek(pngFile, chunkSize, SEEK_CUR);
fread(chunkCRC, 1, 4, pngFile);
}
// Read pixel data
unsigned int width, height;
fread(&width, 4, 1, pngFile);
fread(&height, 4, 1, pngFile);
width = ntohl(width);
height = ntohl(height);
RGB *pixelData = malloc(sizeof(RGB) * width * height);
for (int i = 0; i < width * height; i++) {
fread(&pixelData[i].r, 1, 1, pngFile);
fread(&pixelData[i].g, 1, 1, pngFile);
fread(&pixelData[i].b, 1, 1, pngFile);
}
fclose(pngFile);
// Use pixelData for further operations
free(pixelData);
return 0;
}
```
请注意,上述代码只是一个简单的示例,可能无法处理包含压缩或有多个图层的png文件。如果需要处理更复杂的情况,可以参考PNG文件格式规范进行扩展。
### 回答3:
要将PNG图片文件转化为RGB点阵数组,可以使用C语言实现。以下是一个简单的实现过程:
首先,需要了解PNG图片文件的结构。PNG文件由不同的块组成,其中包含了图像的宽度、高度、位深度、颜色类型等信息。
接下来,读取PNG文件的头部信息。PNG文件的头部长度固定为8个字节,可以通过fread函数读取。
然后,读取每个块的数据。PNG文件中的每个块都包含一个长度字段,用来指示块的长度。可以使用fread函数读取长度字段,并将其转化为整数。接着,读取块的类型字段,并根据不同的块类型进行相应的处理。
如果是IDAT块,表示图像数据块,需要读取块中的数据,并进行解压缩处理。PNG文件中的图像数据是经过压缩的,需要使用合适的压缩算法进行解压缩,将压缩后的数据转化为原始的RGB数据。
最后,将RGB数据存储到一个数组中。可以先计算出RGB点阵数组的大小,并动态分配内存空间。然后,将解压缩得到的RGB数据按照一定的顺序存储到数组中。
需要注意的是,以上仅是一个简单的实现过程,实际上PNG文件的解析还涉及其他一些细节,如校验和的计算、滤波器的应用等。而且,解压缩算法也需要根据PNG文件中压缩的方式进行相应的处理。
总之,将PNG图片文件转化为RGB点阵数组可以通过C语言实现,但是实际的实现过程比较复杂,需要更详细的了解PNG文件的结构和算法的知识。
相关推荐
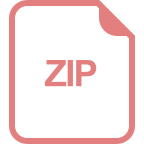

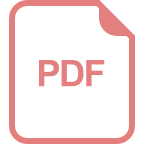
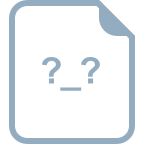
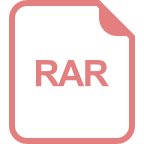
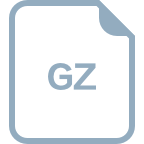
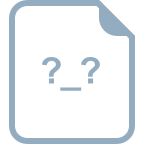