python函数比较三个数的大小
时间: 2023-08-31 15:19:21 浏览: 172
### 回答1:
可以使用以下代码来比较三个数的大小:
```
def compare_three(a, b, c):
if a > b:
if a > c:
print(f"{a} is the largest.")
else:
print(f"{c} is the largest.")
else:
if b > c:
print(f"{b} is the largest.")
else:
print(f"{c} is the largest.")
compare_three(1, 2, 3) # Output: 3 is the largest.
compare_three(3, 2, 1) # Output: 3 is the largest.
compare_three(2, 3, 1) # Output: 3 is the largest.
```
在上面的代码中,我们定义了一个名为`compare_three`的函数,它接受三个参数`a`、`b`和`c`,并比较它们的大小。首先,我们比较`a`和`b`的大小。如果`a`大于`b`,我们再比较`a`和`c`的大小。如果`a`大于`c`,则输出`a`是最大的;否则输出`c`是最大的。如果`a`不大于`b`,则我们比较`b`和`c`的大小。如果`b`大于`c`,则输出`b`是最大的;否则输出`c`是最大的。
### 回答2:
下面是一个用Python编写的函数,用于比较三个数的大小:
```python
def compare_numbers(a, b, c):
if a > b and a > c:
return a, "是最大的数"
elif b > a and b > c:
return b, "是最大的数"
elif c > a and c > b:
return c, "是最大的数"
else:
return "这三个数中存在相同的最大值"
```
该函数接受三个参数 `a`、`b` 和 `c`,分别代表三个要比较的数。通过使用条件语句 `if` 和 `elif`,函数会比较三个数的大小,并返回最大的数以及附带的说明信息。如果三个数中存在相同的最大值,则会返回一个指示相同最大值的消息。
这是一个简单的函数示例,用于比较三个数的大小。根据运行函数时传入的具体数值,函数会返回相应的比较结果。
### 回答3:
下面是一个用Python编写的函数,用于比较三个数的大小:
```python
def compare_three_numbers(a, b, c):
if a >= b and a >= c:
return a
elif b >= a and b >= c:
return b
else:
return c
num1 = 10
num2 = 20
num3 = 30
largest_number = compare_three_numbers(num1, num2, num3)
print("最大的数是:", largest_number)
```
在这个函数中,我们定义了一个函数`compare_three_numbers`来比较三个数的大小。这个函数接受三个参数`a`,`b`和`c`,分别表示三个待比较的数。
首先,我们使用`if`语句和逻辑运算符`>=`来比较`a`和`b`,以及`a`和`c`的大小。如果`a`大于等于`b`并且`a`大于等于`c`,则返回`a`作为最大的数。如果`b`大于等于`a`并且`b`大于等于`c`,则返回`b`作为最大的数。否则,返回`c`作为最大的数。
在主程序中,我们声明了三个数字`num1`,`num2`和`num3`作为要比较的数字。然后,我们调用`compare_three_numbers`函数,并将`num1`,`num2`和`num3`作为参数传递给它。最后,我们打印出最大的数。
在这个例子中,我们假设`num1`等于10,`num2`等于20,`num3`等于30。因此,最大的数是30,将会被打印出来。
相关推荐
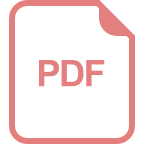
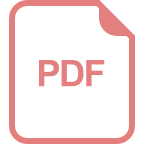














