使用golang读取ini的配置文件,配置文件包含多个服务器信息,每个服务器中包含服务器的ID、访问地址、用户名、密码、启动的服务,启动的服务可以存在多个,每个服务有自己的启动参数,每个服务必须包含启动的ID以及其他的参数,提供读取和写入配置文件的方法和参考的ini文件
时间: 2023-09-06 10:05:05 浏览: 110
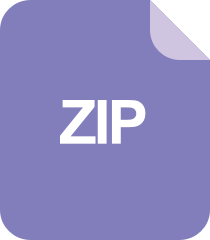
一个golang写的websocket通讯服务器
### 回答1:
使用 Golang 读取 ini 配置文件,您可以使用第三方库 "gcfg" 来实现。
首先,您需要安装 gcfg:
```
go get github.com/go-ini/ini
```
然后,在您的代码中引入 ini 库:
```
import "github.com/go-ini/ini"
```
接下来,您可以使用 Load 方法读取 ini 文件:
```
cfg, err := ini.Load("config.ini")
if err != nil {
// 处理读取配置文件出错的情况
}
```
读取完成后,您就可以使用 cfg 对象来访问配置文件中的信息了。比如,要读取服务器的 ID,您可以这样做:
```
serverID := cfg.Section("server").Key("id").String()
```
如果您想写入配置文件,可以使用 Save 方法:
```
err = cfg.SaveTo("config.ini")
if err != nil {
// 处理写入配置文件出错的情况
}
```
参考 ini 文件示例:
```ini
[server]
id = 123
address = example.com
username = user
password = password
[service]
service1.id = 1
service1.param1 = value1
service1.param2 = value2
service2.id = 2
service2.param1 = value3
service2.param2 = value4
```
### 回答2:
使用golang读取ini的配置文件,可以使用第三方库go-ini来实现。以下是一个示例代码:
```go
package main
import (
"fmt"
"gopkg.in/ini.v1"
)
type Server struct {
ID int
Address string
Username string
Password string
Services []Service
}
type Service struct {
ID int
Params []string
}
func main() {
cfg, err := ini.Load("config.ini")
if err != nil {
fmt.Println("无法加载配置文件:", err)
return
}
serverCount := cfg.Section("").Key("ServerCount").MustInt(0)
servers := make([]Server, serverCount)
for i := 0; i < serverCount; i++ {
serverSection := fmt.Sprintf("Server%d", i+1)
server := Server{
ID: cfg.Section(serverSection).Key("ID").MustInt(0),
Address: cfg.Section(serverSection).Key("Address").String(),
Username: cfg.Section(serverSection).Key("Username").String(),
Password: cfg.Section(serverSection).Key("Password").String(),
}
serviceCount := cfg.Section(serverSection).Key("ServiceCount").MustInt(0)
services := make([]Service, serviceCount)
for j := 0; j < serviceCount; j++ {
serviceSection := fmt.Sprintf("Service%d", j+1)
service := Service{
ID: cfg.Section(serviceSection).Key("ID").MustInt(0),
Params: cfg.Section(serviceSection).Key("Params").Strings(","),
}
services[j] = service
}
server.Services = services
servers[i] = server
}
// 读取配置文件内容
for _, server := range servers {
fmt.Printf("服务器ID: %d\n", server.ID)
fmt.Printf("访问地址: %s\n", server.Address)
fmt.Printf("用户名: %s\n", server.Username)
fmt.Printf("密码: %s\n", server.Password)
for _, service := range server.Services {
fmt.Printf("启动的服务ID: %d\n", service.ID)
fmt.Printf("启动参数: %v\n", service.Params)
}
}
}
```
配置文件`config.ini`的内容如下:
```ini
[Server]
ServerCount = 1
Server1.ID = 1
Server1.Address = example.com
Server1.Username = admin
Server1.Password = pass123
Server1.ServiceCount = 2
[Service]
Service1.ID = 1
Service1.Params = param1,param2,param3
[Service]
Service2.ID = 2
Service2.Params = paramA,paramB,paramC
```
上述示例代码会将配置文件中的服务器信息及其各自的服务信息读取出来,并打印出来。你可以根据实际需求修改代码中的数据结构与打印方式。同样的方式,你也可以将修改后的配置信息写入到配置文件中。
### 回答3:
读取配置文件的方法可以通过使用`github.com/go-ini/ini`包来实现。该包提供了读取和写入INI文件的方法。
首先需要引入该包,使用`import "github.com/go-ini/ini"`导入。然后可以通过调用`ini.Load(filepath)`函数来加载INI文件。
读取配置文件的方法可以通过以下步骤实现:
1. 使用`ini.Load(filepath)`函数加载配置文件。
2. 使用`ini.Section(sectionName)`函数获取相应的章节。
3. 使用`section.Key(keyName).String()`函数获取指定键的值。
写入配置文件的方法可以通过以下步骤实现:
1. 使用`ini.Load(filepath)`函数加载配置文件。
2. 使用`ini.Section(sectionName)`函数获取相应的章节。
3. 使用`section.NewKey(keyName, value)`函数创建一个新键,并设置其值。
4. 使用`section.SaveTo(filepath)`函数将更改保存到配置文件。
下面是一个参考的INI文件示例:
```
[Server1]
ID=1
Address=192.168.0.1
Username=user1
Password=pass1
Services=Service1,Service2
[Server2]
ID=2
Address=192.168.0.2
Username=user2
Password=pass2
Services=Service3
[Service1]
ID=1
Param1=value1
Param2=value2
[Service2]
ID=2
Param1=value3
Param2=value4
[Service3]
ID=3
Param1=value5
Param2=value6
```
希望以上回答对你有帮助!
阅读全文
相关推荐
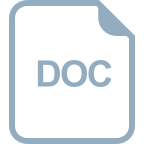
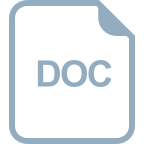






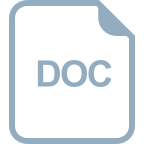
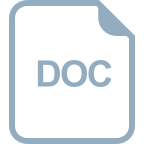
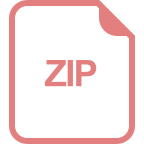
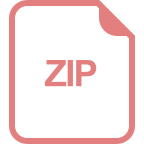
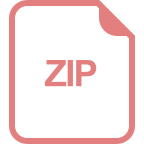