使用golang读取ini的配置文件,配置文件包含多个服务器信息,每个服务器中包含服务器的ID、访问地址、用户名、密码、启动的服务,启动的服务可以存在多个,每个服务有自己的启动参数,每个服务必须包含启动的ID以及其他的参数,回答只需提供参考的ini文件和代码无需多余解释
时间: 2023-08-31 19:29:52 浏览: 83
### 回答1:
使用 golang 读取 ini 配置文件可以使用第三方库 "gcfg"。
首先,需要安装 gcfg 库:
```
go get github.com/go-ini/ini
```
然后,需要创建一个结构体来存储配置文件中的信息。
例如,假设我们的配置文件是这样的:
```ini
[server]
ID = 1
Address = 192.168.1.1
Username = user1
Password = password1
[server.service]
ID = 1
Name = service1
Params = param1 param2
[server.service]
ID = 2
Name = service2
Params = param3 param4
[server]
ID = 2
Address = 192.168.1.2
Username = user2
Password = password2
[server.service]
ID = 3
Name = service3
Params = param5 param6
[server.service]
ID = 4
Name = service4
Params = param7 param8
```
则我们可以定义如下结构体来存储这些信息:
```go
type Config struct {
Server []struct {
ID int
Address string
Username string
Password string
Service []struct {
ID int
Name string
Params string
}
}
}
```
最后,使用 gcfg 库读取配置文件:
```go
import "github.com/go-ini/ini"
var config Config
err := ini.MapTo(&config, "config.ini")
if err != nil {
panic(err)
}
// 然后就可以使用 config 变量来访问配置文件中的信息了
```
注意:在定义结构体时,需要使用配置文件中的节名和属性名来命名结构体中的字段,否则 gcfg 库无法正确读取配置文件。
### 回答2:
ini配置文件示例:
```
[Server1]
ID = 1
Address = 127.0.0.1
Username = user1
Password = pass1
Services = service1, service2
[Server2]
ID = 2
Address = 192.168.0.1
Username = user2
Password = pass2
Services = service3
[Service1]
ID = 1
Param = param1
[Service2]
ID = 2
Param = param2
[Service3]
ID = 3
Param = param3
```
使用golang读取ini配置文件的代码示例:
```go
package main
import (
"fmt"
"gopkg.in/ini.v1"
)
type Server struct {
ID int
Address string
Username string
Password string
Services []Service
}
type Service struct {
ID int
Param string
}
func main() {
cfg, err := ini.Load("config.ini")
if err != nil {
fmt.Println("Failed to read config file:", err)
return
}
server1 := Server{
ID: cfg.Section("Server1").Key("ID").MustInt(),
Address: cfg.Section("Server1").Key("Address").String(),
Username: cfg.Section("Server1").Key("Username").String(),
Password: cfg.Section("Server1").Key("Password").String(),
}
services := cfg.Section("Server1").Key("Services").Strings(",")
for _, serviceName := range services {
service := Service{
ID: cfg.Section(serviceName).Key("ID").MustInt(),
Param: cfg.Section(serviceName).Key("Param").String(),
}
server1.Services = append(server1.Services, service)
}
server2 := Server{
// 处理Server2的信息,类似于Server1
}
fmt.Println(server1)
fmt.Println(server2)
}
```
请将上面的代码保存为main.go,并在同一目录下创建一个名为config.ini的文件,并将ini配置文件示例中的内容保存到config.ini中。运行main.go即可读取配置文件并输出服务器信息。
### 回答3:
ini配置文件示例(config.ini):
```
[server1]
id = 1
address = 192.168.0.1
username = user1
password = password1
services = service1,service2
[server2]
id = 2
address = 192.168.0.2
username = user2
password = password2
services = service3,service4
[service1]
id = 1
parameter1 = value1
parameter2 = value2
[service2]
id = 2
parameter1 = value3
parameter2 = value4
[service3]
id = 3
parameter1 = value5
parameter2 = value6
[service4]
id = 4
parameter1 = value7
parameter2 = value8
```
Go代码示例:
```go
package main
import (
"fmt"
"log"
"gopkg.in/ini.v1"
)
type Server struct {
ID int
Address string
Username string
Password string
Services []Service
}
type Service struct {
ID int
Parameter1 string
Parameter2 string
}
func main() {
cfg, err := ini.Load("config.ini")
if err != nil {
log.Fatalf("Failed to load config.ini: %v", err)
}
var servers []Server
serverSections := cfg.SectionStrings()
for _, section := range serverSections {
if section == ini.DefaultSection {
continue
}
server := Server{
ID: cfg.Section(section).Key("id").MustInt(),
Address: cfg.Section(section).Key("address").String(),
Username: cfg.Section(section).Key("username").String(),
Password: cfg.Section(section).Key("password").String(),
}
serviceNames := cfg.Section(section).Key("services").Strings(",")
for _, serviceName := range serviceNames {
service := Service{
ID: cfg.Section(serviceName).Key("id").MustInt(),
Parameter1: cfg.Section(serviceName).Key("parameter1").String(),
Parameter2: cfg.Section(serviceName).Key("parameter2").String(),
}
server.Services = append(server.Services, service)
}
servers = append(servers, server)
}
// 打印服务器信息
for _, server := range servers {
fmt.Println("Server ID:", server.ID)
fmt.Println("Address:", server.Address)
fmt.Println("Username:", server.Username)
fmt.Println("Password:", server.Password)
fmt.Println("Services:")
for _, service := range server.Services {
fmt.Println(" - Service ID:", service.ID)
fmt.Println(" Parameter1:", service.Parameter1)
fmt.Println(" Parameter2:", service.Parameter2)
}
fmt.Println()
}
}
```
以上代码使用了`ini`包来解析ini配置文件,首先读取配置文件的所有节(section),然后依次读取每个服务器的信息。每个服务器根据配置文件中的"services"键读取对应的服务信息,最后将得到的服务器信息存储在`servers`切片中,并打印出来。
阅读全文
相关推荐
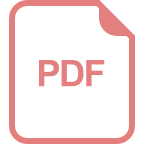






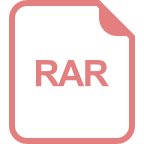
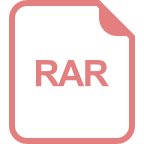
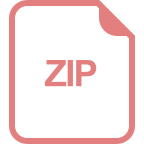
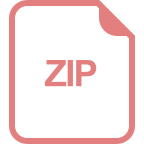
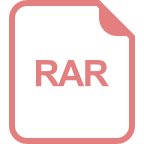