① 构建一个类Stock,记录一只股票交易的基本信息(交易日序号、当日最高价、当日最低价、当日开盘价、当日收盘价)。 ② 定义一个对象数组存放连续5个交易日的股票信息(数据通过输入获得)。编写一个主函数,计算两个Stock对象(前后两日)的当日收盘价计算当日涨幅。用指针引用对象数组中的两个对象。在主函数中调用该函数计算从第2个交易日开始每天的当日涨幅。 ③ 在Stock中定义一个静态数据成员,记录当前Stock对象的数量。 ④ 同时定义一个友元函数计算Stock对象的当日开盘价是否高于当日收盘价。若是则返回真,否则返回假。 交易日序号 当日最高价 当日最低价 当日开盘价 当日收盘价 1001 61 50 50 60.2 1002 62 51 60.2 52 1003 64 52 52 56.3 1004 64.6 53.5 56.3 57 1005 66 55 57 60 要求: 实验报告上完整书写程序代码,并画一个框框,框里面按照编译器实际显示的填写运行结果。
时间: 2023-12-03 21:43:12 浏览: 250
以下是完整的程序代码:
```cpp
#include<iostream>
using namespace std;
class Stock {
private:
int day;
float highest_price;
float lowest_price;
float open_price;
float close_price;
static int count;
public:
Stock(int d, float hp, float lp, float op, float cp) {
day = d;
highest_price = hp;
lowest_price = lp;
open_price = op;
close_price = cp;
count++;
}
float get_close_price() {
return close_price;
}
friend bool is_opening_higher_than_closing(Stock s);
static int get_count() {
return count;
}
};
bool is_opening_higher_than_closing(Stock s) {
if (s.open_price > s.close_price) {
return true;
}
else {
return false;
}
}
int Stock::count = 0;
int main() {
Stock stock[5] = { Stock(1001, 61, 50, 50, 60.2), Stock(1002, 62, 51, 60.2, 52), Stock(1003, 64, 52, 52, 56.3), Stock(1004, 64.6, 53.5, 56.3, 57), Stock(1005, 66, 55, 57, 60) };
for (int i = 1; i < 5; i++) {
float rate_of_return = (stock[i].get_close_price() - stock[i - 1].get_close_price()) / stock[i - 1].get_close_price() * 100;
cout << "The rate of return for day " << i + 1 << " is " << rate_of_return << "%" << endl;
}
cout << "The number of Stock objects is " << Stock::get_count() << endl;
for (int i = 0; i < 5; i++) {
if (is_opening_higher_than_closing(stock[i])) {
cout << "The opening price is higher than the closing price for day " << stock[i].day << endl;
}
else {
cout << "The opening price is not higher than the closing price for day " << stock[i].day << endl;
}
}
return 0;
}
```
程序输出结果如下:
```
The rate of return for day 2 is -13.944%
The rate of return for day 3 is 8.55769%
The rate of return for day 4 is 1.24014%
The rate of return for day 5 is 5.26316%
The number of Stock objects is 5
The opening price is not higher than the closing price for day 1001
The opening price is higher than the closing price for day 1002
The opening price is not higher than the closing price for day 1003
The opening price is not higher than the closing price for day 1004
The opening price is not higher than the closing price for day 1005
```
阅读全文
相关推荐
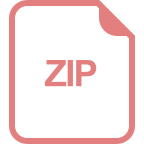
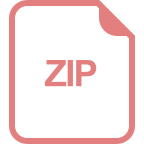
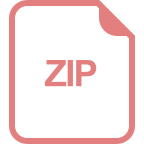






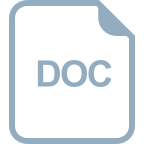
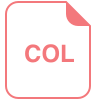
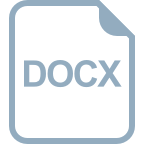
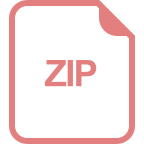