使用protobufjs的encode方法生成的buffer和之前的对象不一致,怎么回事
时间: 2023-02-09 19:41:15 浏览: 146
protobuf.js 是一个用于在 JavaScript 中使用 Protocol Buffers 的库。当使用 protobuf.js 的 encode 方法时,它会将 JavaScript 对象编码为二进制数据。
如果在使用 encode 方法生成的 buffer 与之前的对象不一致,可能是由于以下几种原因:
1. 使用的是错误的编码规则:确保在调用 encode 方法时使用的是正确的编码规则。
2. 对象的属性发生了改变:在编码对象之前,如果对象的属性发生了改变,则会导致编码后的结果与原对象不一致。
3. 编码规则有误:如果编码规则定义有误,则编码后的结果可能与原对象不一致。
如果确认无误,可以尝试使用 decode 方法将 buffer 解码为 JavaScript 对象,并与原对象进行比较,以了解编码后的结果与原对象的差异。
相关问题
如何在前端中使用protobuf(vue篇)
在前端中使用 Protobuf(Protocol Buffers)可以通过以下步骤:
1. 安装依赖:首先,你需要在 Vue 项目中安装 protobuf.js 库。可以使用 npm 或者 yarn 进行安装:
```bash
npm install protobufjs
```
2. 定义你的 Protobuf 消息:在 Vue 项目中,你可以创建一个 `.proto` 文件来定义你的消息结构。例如,创建一个名为 `message.proto` 的文件,并在其中定义你的消息类型。
```protobuf
syntax = "proto3";
message MyMessage {
string name = 1;
int32 age = 2;
}
```
3. 编译 Protobuf 文件:使用 protobuf.js 的命令行工具 `pbjs` 来编译你的 `.proto` 文件。在项目的根目录下运行以下命令:
```bash
npx pbjs -t static -w es6 -o src/protobufBundle.js path/to/message.proto
```
这将生成一个名为 `protobufBundle.js` 的文件,其中包含了编译后的 Protobuf 消息定义。
4. 引入 protobuf.js 和生成的 bundle 文件:在 Vue 组件中引入 protobuf.js 和生成的 bundle 文件。
```javascript
import protobuf from 'protobufjs';
import protobufBundle from './protobufBundle';
// 加载编译后的 Protobuf 消息定义
const root = protobuf.parse(protobufBundle).root;
const MyMessage = root.lookupType('MyMessage');
```
5. 使用 Protobuf 消息:现在你可以在 Vue 组件中使用 Protobuf 消息了。例如,你可以创建一个表单,将用户输入的值存储到 Protobuf 消息中,并进行序列化和发送。
```javascript
export default {
data() {
return {
name: '',
age: 0,
serializedMessage: '',
};
},
methods: {
sendMessage() {
// 创建一个新的消息对象
const message = MyMessage.create({
name: this.name,
age: this.age,
});
// 序列化消息对象
const buffer = MyMessage.encode(message).finish();
// 将序列化后的消息发送到服务器等等
// ...
// 将序列化后的消息保存到组件的数据中,以备显示或其他用途
this.serializedMessage = buffer;
},
},
};
```
这样,你就可以在 Vue 组件中使用 Protobuf 消息了。记得根据你的实际需求来修改和扩展上述代码。
前端项目如何使用protostuff来进行序列化反序列化
首先,需要在前端项目中引入 `protostuff` 库。可以通过 npm 安装或者使用 `<script>` 标签引入。
接下来,需要定义用于序列化和反序列化的数据结构。可以使用 protobuf 定义数据结构,然后通过 `protostuff` 的 `Message` 类来创建 JavaScript 类。
例如,假设我们有一个 protobuf 文件定义了一个 `Person` 消息类型:
```protobuf
syntax = "proto3";
message Person {
string name = 1;
int32 age = 2;
}
```
我们可以使用 `protoc` 编译器来生成对应的 JavaScript 代码:
```
protoc --js_out=import_style=commonjs,binary:. person.proto
```
然后在前端项目中引入生成的代码:
```javascript
const ProtoBuf = require('protobufjs');
const proto = require('./person_pb');
// create a Person message
const person = new proto.Person();
person.setName('Alice');
person.setAge(30);
// serialize the message to a Uint8Array
const buffer = proto.Person.encode(person).finish();
// deserialize the message from a Uint8Array
const decodedPerson = proto.Person.decode(buffer);
console.log(decodedPerson.getName()); // output: "Alice"
console.log(decodedPerson.getAge()); // output: 30
```
在这个例子中,我们使用 `Person` 类来创建一个 `person` 对象,并设置其属性。然后将其序列化为一个 `Uint8Array`,并在反序列化时使用 `Person` 类来解码该数组并还原为 `decodedPerson` 对象。
需要注意的是,`protostuff` 库本身并不支持在浏览器中使用,因为它依赖于 Node.js 的 `Buffer` 类。但是可以使用一些类似 `buffer` 的库,如 `typedarray-to-buffer` 或 `base64-arraybuffer`,将 `Uint8Array` 转换为 `buffer`,然后再进行序列化和反序列化操作。
阅读全文
相关推荐
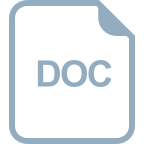
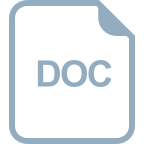
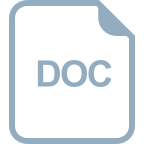
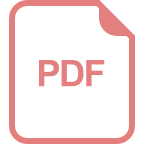
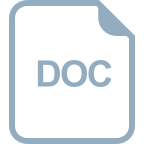
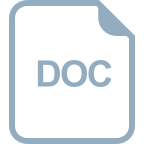
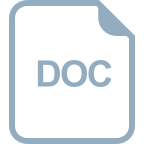