用c语言编写mystrcmp函数
时间: 2023-02-09 18:41:13 浏览: 141
在 C 语言中,你可以使用 strcmp 函数来比较两个字符串的大小。
它的原型如下:
```
int strcmp(const char *s1, const char *s2);
```
其中,s1 和 s2 分别是要比较的两个字符串。如果 s1 小于 s2,则 strcmp 返回一个负数;如果 s1 等于 s2,则 strcmp 返回 0;如果 s1 大于 s2,则 strcmp 返回一个正数。
例如,你可以使用 strcmp 函数来比较两个字符串的大小:
```
#include <stdio.h>
#include <string.h>
int main()
{
char *s1 = "hello";
char *s2 = "world";
int result = strcmp(s1, s2);
if (result < 0)
printf("%s is less than %s\n", s1, s2);
else if (result > 0)
printf("%s is greater than %s\n", s1, s2);
else
printf("%s is equal to %s\n", s1, s2);
return 0;
}
```
如果你想编写一个自己的 mystrcmp 函数,可以使用以下代码:
```
#include <stdio.h>
int mystrcmp(const char *s1, const char *s2)
{
while (*s1 && (*s1 == *s2))
{
s1++;
s2++;
}
return *s1 - *s2;
}
int main()
{
char *s1 = "hello";
char *s2 = "world";
int result = mystrcmp(s1, s2);
if (result < 0)
printf("%s is less than %s\n", s1, s2);
else if (result > 0)
printf("%s is greater than %s\n", s1, s2);
else
printf("%s is equal to %s\n", s1, s2);
return 0;
}
```
在这个代码中,我们使用一个循环来比较两个字符串的每一个字符。
阅读全文
相关推荐






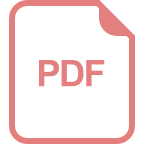

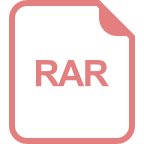

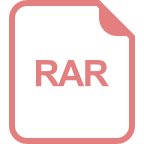





