c语言字符串提取数字
时间: 2023-02-05 20:21:57 浏览: 117
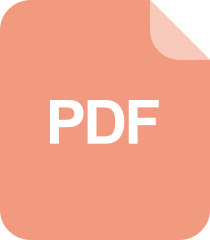
js实现在字符串中提取数字
在 C 语言中,可以使用 sscanf 函数来从字符串中提取数字。
例如,如果要从字符串 "123 45" 中提取两个数字,可以使用以下代码:
```
char str[] = "123 45";
int x, y;
sscanf(str, "%d %d", &x, &y);
```
在上面的代码中,"%d" 表示要提取整数。如果要提取浮点数,可以使用 "%f"。
注意,sscanf 函数可能会返回提取的数据数量,因此可以使用返回值来判断是否成功提取了所有的数据。
例如:
```
if (sscanf(str, "%d %d", &x, &y) != 2) {
printf("Error: Unable to extract 2 numbers from string.\n");
}
```
阅读全文
相关推荐

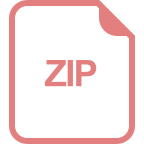
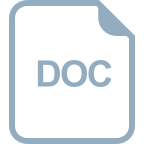







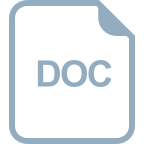




