Java用mapreduce输出数据保存到本地先写main代码
时间: 2023-08-31 16:19:23 浏览: 112
### 回答1:
首先,您需要配置Hadoop环境并导入相关的Java包,然后可以使用MapReduce API来编写Java代码。
具体来说,您需要实现Mapper和Reducer接口,分别处理输入数据并输出中间结果。然后,您需要实现Job类来将Mapper和Reducer组装在一起,并设置作业的输入输出路径以及相关的参数。最后,您需要在main方法中调用Job的waitForCompletion方法来提交作业并等待它完成。
示例代码如下:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class WordCount {
public static class WordCountMapper
extends Mapper<Object, Text, Text, IntWritable>{
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(Object key, Text value, Context context
) throws IOException, InterruptedException {
String[] words = value.toString().split(" ");
for (String w : words) {
word.set(w);
context.write(word, one);
}
}
}
public static class WordCountReducer
extends Reducer<Text,IntWritable,Text,IntWritable> {
private IntWritable result = new IntWritable();
public void reduce(Text key, Iterable<IntWritable> values,
Context context
) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
result.set(sum);
context.write(key, result);
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job =
### 回答2:
Java中使用MapReduce输出数据并保存到本地需要编写主要的代码。下面是一个示例的主要代码,该代码使用MapReduce输出数据并将结果保存到本地。
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.io.NullWritable;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
import java.io.IOException;
public class MapReduceToSaveDataLocally {
public static class MapClass extends Mapper<Object, Text, Text, NullWritable> {
@Override
protected void map(Object key, Text value, Context context) throws IOException, InterruptedException {
// 处理输入的数据并输出到Reducer
// 这里是一个示例,你可以根据自己的需求进行修改
context.write(value, NullWritable.get());
}
}
public static class ReduceClass extends Reducer<Text, NullWritable, Text, NullWritable> {
@Override
protected void reduce(Text key, Iterable<NullWritable> values, Context context) throws IOException, InterruptedException {
// 处理Mapper输出的数据并保存到本地
// 这里是一个示例,你可以根据自己的需求进行修改
context.write(key, NullWritable.get());
}
}
public static void main(String[] args) throws IOException, ClassNotFoundException, InterruptedException {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "MapReduce to Save Data Locally");
job.setJarByClass(MapReduceToSaveDataLocally.class);
job.setMapperClass(MapClass.class);
job.setReducerClass(ReduceClass.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(NullWritable.class);
// 设置输入和输出路径
FileInputFormat.setInputPaths(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
// 删除已存在的输出目录
FileSystem fs = FileSystem.get(conf);
fs.delete(new Path(args[1]), true);
// 提交MapReduce作业并等待完成
int returnValue = job.waitForCompletion(true) ? 0 : 1;
System.exit(returnValue);
}
}
```
以上是一个示例的Java代码,用于使用MapReduce将数据保存到本地。你可以根据自己的需求进行适当的修改,例如修改Mapper和Reducer类的逻辑以及设置实际的输入和输出路径。请注意,你需要正确配置Hadoop相关的环境并提供正确的输入和输出路径参数才能成功运行该代码。
### 回答3:
在Java中使用MapReduce输出数据并保存到本地,首先需要编写Main代码。以下是一个简单的示例代码:
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
import java.io.IOException;
public class MapReduceMain {
public static class Map extends Mapper<LongWritable, Text, Text, Text> {
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
// Mapper的逻辑
// 将输入数据进行处理,并将结果写入Context中,作为Reducer的输入
}
}
public static class Reduce extends Reducer<Text, Text, Text, Text> {
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
// Reducer的逻辑
// 对Mapper的输出进行聚合处理,并将最终结果写入Context中,作为输出
}
}
public static void main(String[] args) throws IOException, ClassNotFoundException, InterruptedException {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "MapReduce Example");
job.setJarByClass(MapReduceMain.class);
job.setMapperClass(Map.class);
job.setReducerClass(Reduce.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path("input_path")); // 设置输入路径
FileOutputFormat.setOutputPath(job, new Path("output_path")); // 设置输出路径
FileSystem fs = FileSystem.get(conf);
if (fs.exists(new Path("output_path"))) {
fs.delete(new Path("output_path"), true); // 如果输出路径已存在,则删除之前的结果
}
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在上述示例代码中,首先定义了两个内部类`Map`和`Reduce`,分别继承自`Mapper`和`Reducer`。在`Map`类的`map`方法中,可以编写自定义的Mapper逻辑,将输入数据进行处理并将结果写入Context中。在`Reduce`类的`reduce`方法中,可以编写自定义的Reducer逻辑,对Mapper的输出进行聚合处理,并将最终结果写入Context中。
在`main`方法中,首先创建一个`Configuration`对象,并通过`Job`类创建一个MapReduce任务对象。设置任务的各项属性,包括输入路径、输出路径、Mapper和Reducer的类、输出键值对的类型等。在设置完属性后,通过`FileSystem`对象检查输出路径是否已经存在,如果存在则删除之前的结果。最后调用`job.waitForCompletion(true)`方法提交任务,并通过`System.exit`方法等待任务完成。
请注意,上述示例代码中未包含Mapper和Reducer的具体实现逻辑,需要根据实际需求进行编写。同时,输入路径和输出路径需要根据具体的文件系统设置正确的路径。
阅读全文
相关推荐
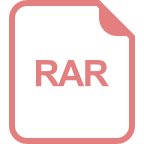
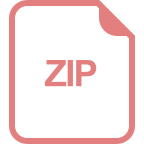
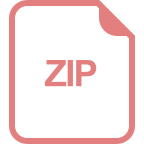


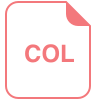
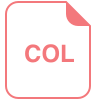
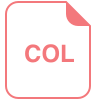
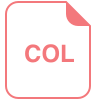




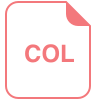
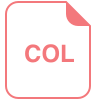
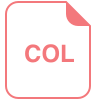
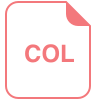
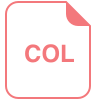
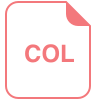