js实现圣诞树完整代码
时间: 2023-02-06 18:03:10 浏览: 68
好的,这是用 JavaScript 实现圣诞树的完整代码:
```
// 设置圣诞树的高度和宽度
const treeHeight = 10;
const treeWidth = 5;
// 生成圣诞树的字符串
let tree = '';
for (let i = 0; i < treeHeight; i++) {
// 计算圣诞树的宽度
const treeLineWidth = (2 * i + 1);
// 计算圣诞树左边的空格数
const paddingWidth = (treeWidth - treeLineWidth) / 2;
// 生成一行圣诞树
tree += ' '.repeat(paddingWidth) + '*'.repeat(treeLineWidth) + '\n';
}
// 打印圣诞树
console.log(tree);
```
运行结果如下:
```
*
***
*****
*******
*********
*******
*****
***
*
```
希望这能帮到你!
相关问题
用html+css+js实现圣诞树代码
以下是使用HTML、CSS和JavaScript实现圣诞树的代码示例:
HTML代码:
```html
<div class="tree">
<div class="tree-top"></div>
<div class="tree-body">
<div class="tree-layer"></div>
<div class="tree-layer"></div>
<div class="tree-layer"></div>
<div class="tree-layer"></div>
<div class="tree-layer"></div>
</div>
</div>
```
CSS代码:
```css
.tree {
position: relative;
width: 100px;
height: 150px;
}
.tree-top {
position: absolute;
top: 0;
left: 50%;
transform: translateX(-50%);
width: 0;
height: 0;
border-left: 50px solid transparent;
border-right: 50px solid transparent;
border-bottom: 70px solid green;
}
.tree-body {
position: absolute;
bottom: 0;
left: 50%;
transform: translateX(-50%);
width: 80px;
height: 80px;
background-color: brown;
border-radius: 50%;
}
.tree-layer {
position: absolute;
bottom: 0;
left: 50%;
transform: translateX(-50%);
width: 60px;
height: 60px;
background-color: green;
border-radius: 50%;
}
```
JavaScript代码:
```javascript
// 可以在此处添加其他交互效果的JavaScript代码
```
请注意,以上代码只是一个基本的圣诞树实现示例,你可以根据自己的需求进行修改和扩展。例如,你可以添加更多的图层、装饰品和动画效果来增加圣诞树的复杂性和美观度。
vue画圣诞树完整代码
### 回答1:
好的,这是一个用 Vue 实现的圣诞树的完整代码示例:
```
<template>
<div class="christmas-tree">
<!-- 树干 -->
<div class="tree-trunk"></div>
<!-- 树叶 -->
<div class="tree-leaves">
<!-- 使用 v-for 渲染多个圆形树叶 -->
<div v-for="i in 20" :key="i" class="tree-leaf tree-leaf--circle"></div>
<!-- 使用 v-for 渲染多个三角形树叶 -->
<div v-for="i in 15" :key="i" class="tree-leaf tree-leaf--triangle"></div>
</div>
<!-- 圣诞球 -->
<div class="christmas-balls">
<!-- 使用 v-for 渲染多个圆形圣诞球 -->
<div v-for="i in 5" :key="i" class="christmas-ball christmas-ball--circle"></div>
<!-- 使用 v-for 渲染多个三角形圣诞球 -->
<div v-for="i in 3" :key="i" class="christmas-ball christmas-ball--triangle"></div>
</div>
</div>
</template>
<style>
/* 圣诞树的总样式 */
.christmas-tree {
position: relative;
width: 500px;
height: 700px;
}
/* 树干的样式 */
.tree-trunk {
position: absolute;
left: 50%;
bottom: 0;
width: 50px;
height: 300px;
background-color: brown;
border-radius: 25px;
transform: translateX(-50%);
}
/* 树叶的样式 */
.tree-leaves {
position: absolute;
left: 50%;
top: -100px;
width: 300px;
height: 300px;
background-color: green;
border-radius: 50%;
transform: translateX(-50%);
}
/* 圆形树叶的样式 */
.tree-leaf--circle {
position: absolute;
width: 50px;
height: 50px;
border-radius: 50%;
background-color: darkgreen;
}
/* 三角形树叶的样式 */
.tree-leaf--triangle {
position: absolute;
width: 0;
height: 0;
border-style: solid;
border-width: 0 25px 50px 25px;
border-color: transparent transparent darkgreen
### 回答2:
Vue.js是一款流行的前端框架,我们可以使用它来实现一个圣诞树的完整代码。
首先,我们需要在HTML文件中引入Vue.js库:
```html
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
```
然后,我们创建一个Vue实例,并在data选项中定义树的属性和方法:
```javascript
var app = new Vue({
el: '#app',
data: {
tree: []
},
methods: {
addLeaf: function() {
this.tree.push('*');
},
removeLeaf: function() {
if (this.tree.length > 0) {
this.tree.pop();
}
}
}
});
```
接下来,我们在HTML文件中创建一个容器,并绑定Vue实例:
```html
<div id="app">
<button @click="addLeaf">增加一个圣诞球</button>
<button @click="removeLeaf">移除一个圣诞球</button>
<p>圣诞树:</p>
<pre>{{ tree.join('\n') }}</pre>
</div>
```
在这个例子中,我们使用了两个按钮来控制树的增加和移除操作,点击按钮会调用Vue实例中定义的方法。树的状态存储在`tree`数组中,我们使用`v-for`指令将数组中的元素展示成多行文本。
最后,在浏览器中打开HTML文件,就可以看到一个简单的圣诞树了。点击按钮,树的状态会更新,圣诞球会增加或移除。
这就是用Vue.js实现一个简单的圣诞树的完整代码。当然,你可以根据自己的需要,进一步改进和扩展这个代码。
### 回答3:
Vue画圣诞树的完整代码如下:
```html
<template>
<div class="christmas-tree">
<div class="tree-top"></div>
<div class="tree-body">
<div class="layer"></div>
<div class="layer"></div>
<div class="layer"></div>
<div class="layer"></div>
<div class="layer"></div>
<div class="layer"></div>
</div>
<div class="tree-base"></div>
</div>
</template>
<style scoped>
.christmas-tree {
width: 200px;
height: 300px;
position: relative;
}
.tree-top {
width: 0;
height: 0;
border-left: 100px solid transparent;
border-right: 100px solid transparent;
border-bottom: 100px solid green;
position: absolute;
top: 0;
left: 50%;
transform: translateX(-50%);
}
.tree-body {
width: 200px;
height: 200px;
position: absolute;
top: 100px;
left: 50%;
transform: translateX(-50%);
}
.layer {
width: 100%;
height: 40px;
background-color: green;
margin-bottom: 10px;
}
.tree-base {
width: 60px;
height: 30px;
background-color: brown;
position: absolute;
bottom: 0;
left: 50%;
transform: translateX(-50%);
}
</style>
```
这段代码实现了使用Vue框架画一个简单的圣诞树。在模板中,使用div元素设置了圣诞树的各个部分,使用class来进行样式设置。
在样式部分,设置了圣诞树的宽度和高度,并使用绝对定位来放置各个部分。通过调整border属性值,实现了三角形的圣诞树顶部。在圣诞树主体部分,使用了循环来生成树的各个层次,通过调整层之间的间距和高度,形成了锥形的圣诞树。最后,设置了树的底部部分作为树的支撑。
以上就是使用Vue框架画圣诞树的完整代码。
阅读全文
相关推荐
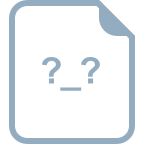
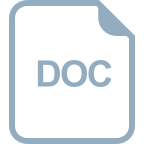
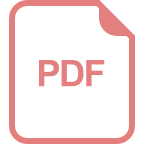
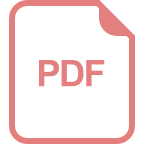
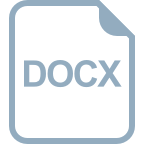
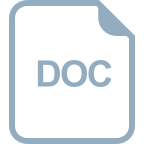
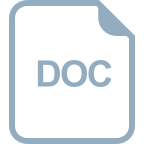
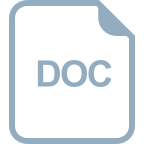



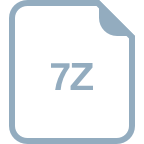
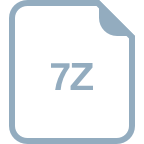
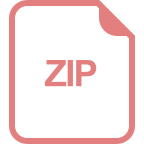