java编写程序,使用IntetAddress类实现根据域名查找I地址的功能。
时间: 2023-09-22 17:06:41 浏览: 182
### 回答1:
在Java中,可以使用IntetAddress类来实现根据域名查找IP地址的功能。
下面是一个例子:
```
import java.net.InetAddress;
public class DomainToIP {
public static void main(String[] args) {
try {
// 获取域名对应的IP地址
InetAddress address = InetAddress.getByName("www.example.com");
String ip = address.getHostAddress();
System.out.println("IP地址: " + ip);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在上面的程序中,我们使用IntetAddress类的getByName()方法来获取域名对应的IP地址。getByName()方法接受一个域名字符串作为参数,并返回一个InetAddress对象。然后,我们使用getHostAddress()方法来获取IP地址。
最后,我们输出了IP地址。
希望这个例子能帮助你。
### 回答2:
Java编写程序,使用InetAddress类可以很方便地实现根据域名查找IP地址的功能。
首先,我们需要使用InetAddress类的静态方法`getByName()`,它接收一个表示域名的字符串参数,并返回一个InetAddress对象。
下面是一个示例代码:
```java
import java.net.InetAddress;
import java.net.UnknownHostException;
public class IPFinder {
public static void main(String[] args) {
String domainName = "www.example.com";
try {
InetAddress address = InetAddress.getByName(domainName);
System.out.println("域名:" + domainName);
System.out.println("IP地址:" + address.getHostAddress());
} catch (UnknownHostException e) {
System.out.println("无法找到域名的IP地址:" + domainName);
}
}
}
```
在上述代码中,首先指定要查询的域名为"www.example.com",然后通过`InetAddress.getByName()`方法获取到对应的InetAddress对象。在try块中,我们使用`getHostAddress()`方法获取到该域名的IP地址,并输出到控制台上。
如果无法找到域名对应的IP地址,会抛出UnknownHostException异常,在catch块中输出相应的错误信息。
需要注意的是,在使用InetAddress类时,有可能会抛出UnknownHostException异常,因此我们需要适当地处理这种异常情况。
通过以上代码,我们就可以实现根据域名查找IP地址的功能了。
### 回答3:
Java编写程序,使用InetAddress类实现根据域名查找IP地址的功能非常简单。以下是一个示例代码:
```java
import java.net.InetAddress;
import java.net.UnknownHostException;
public class DomainToIP {
public static void main(String[] args) {
// 域名
String domain = "www.example.com";
try {
// 获取InetAddress对象(可能有多个IP地址)
InetAddress[] addresses = InetAddress.getAllByName(domain);
// 遍历IP地址
for (InetAddress address : addresses) {
System.out.println("域名" + domain + "对应的IP地址为:" + address.getHostAddress());
}
} catch (UnknownHostException e) {
e.printStackTrace();
}
}
}
```
运行以上代码,将输出域名`www.example.com`对应的IP地址。
首先,我们创建一个`InetAddress`对象,然后调用`getAllByName`方法,传入域名作为参数。该方法将返回一个`InetAddress`数组,包含了该域名对应的所有IP地址。接着,我们可以通过遍历这些`InetAddress`对象,使用`getHostAddress`方法获取IP地址并进行输出。
请注意,如果指定的域名无法解析或者没有对应的IP地址,将会抛出`UnknownHostException`异常。因此,在实际应用中,我们应该在代码中对异常进行合理的处理。
阅读全文
相关推荐





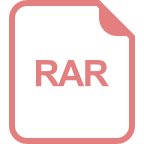
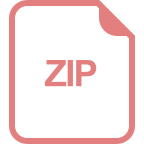


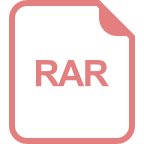


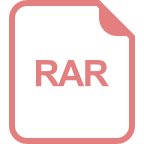


