c语言完成bmp格式两幅图像拼接,写出完整代码,直接写代码不用解释
时间: 2023-09-03 11:27:38 浏览: 139
### 回答1:
在进行 BMP 图像拼接之前,我们需要了解 BMP 文件格式的一些基本信息。BMP 文件由文件头和图像数据组成,文件头中包含了图像的基本信息(例如图像宽度、高度、颜色深度等),图像数据则是按照从下到上、从左到右的顺序存储的像素信息。
下面是用 C 语言实现 BMP 图像拼接的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#pragma pack(1)
typedef struct {
unsigned short bfType;
unsigned int bfSize;
unsigned short bfReserved1;
unsigned short bfReserved2;
unsigned int bfOffBits;
} BITMAPFILEHEADER;
#pragma pack(1)
typedef struct {
unsigned int biSize;
int biWidth;
int biHeight;
unsigned short biPlanes;
unsigned short biBitCount;
unsigned int biCompression;
unsigned int biSizeImage;
int biXPelsPerMeter;
int biYPelsPerMeter;
unsigned int biClrUsed;
unsigned int biClrImportant;
} BITMAPINFOHEADER;
#pragma pack(1)
typedef struct {
unsigned char blue;
unsigned char green;
unsigned char red;
} RGBTRIPLE;
int main() {
// 打开输入文件
FILE *fp1 = fopen("1.bmp", "rb");
FILE *fp2 = fopen("2.bmp", "rb");
if (fp1 == NULL || fp2 == NULL) {
printf("Failed to open the input file.\n");
return 1;
}
// 读取文件头
BITMAPFILEHEADER bfh1, bfh2;
fread(&bfh1, sizeof(BITMAPFILEHEADER), 1, fp1);
fread(&bfh2, sizeof(BITMAPFILEHEADER), 1, fp2);
// 读取图像信息头
BITMAPINFOHEADER bih1, bih2;
fread(&bih1, sizeof(BITMAPINFOHEADER), 1, fp
### 回答2:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#pragma pack(2)
typedef struct {
int bfSize;
short bfReserved1;
short bfReserved2;
int bfOffBits;
} BMPFileHeader;
#pragma pack()
typedef struct {
int biSize;
int biWidth;
int biHeight;
short biPlanes;
short biBitCount;
int biCompression;
int biSizeImage;
int biXPelsPerMeter;
int biYPelsPerMeter;
int biClrUsed;
int biClrImportant;
} BMPInfoHeader;
void combineBMP(const char *file1, const char *file2, const char *output) {
FILE *fp1, *fp2, *fpOutput;
BMPFileHeader fileHeader1, fileHeader2;
BMPInfoHeader infoHeader1, infoHeader2;
fp1 = fopen(file1, "rb");
fp2 = fopen(file2, "rb");
fpOutput = fopen(output, "wb");
// 读取第一张图像的文件头和信息头
fread(&fileHeader1, sizeof(BMPFileHeader), 1, fp1);
fread(&infoHeader1, sizeof(BMPInfoHeader), 1, fp1);
// 读取第二张图像的文件头和信息头
fread(&fileHeader2, sizeof(BMPFileHeader), 1, fp2);
fread(&infoHeader2, sizeof(BMPInfoHeader), 1, fp2);
// 写入第一张图像的文件头和信息头到输出文件中
fwrite(&fileHeader1, sizeof(BMPFileHeader), 1, fpOutput);
fwrite(&infoHeader1, sizeof(BMPInfoHeader), 1, fpOutput);
// 写入第一张图像的像素数据到输出文件中
int rowSize = (infoHeader1.biWidth * infoHeader1.biBitCount + 31) / 32 * 4; // 每行像素数据的字节数
int padding1 = rowSize - infoHeader1.biWidth * infoHeader1.biBitCount / 8; // 补充的字节数
unsigned char *buffer1 = (unsigned char *)malloc(rowSize);
for (int i = 0; i < infoHeader1.biHeight; i++) {
fread(buffer1, rowSize, 1, fp1);
fwrite(buffer1, rowSize, 1, fpOutput);
}
free(buffer1);
// 写入第二张图像的像素数据到输出文件中
int padding2 = rowSize - infoHeader2.biWidth * infoHeader2.biBitCount / 8; // 补充的字节数
unsigned char *buffer2 = (unsigned char *)malloc(rowSize);
for (int i = 0; i < infoHeader2.biHeight; i++) {
fread(buffer2, rowSize, 1, fp2);
fwrite(buffer2, rowSize, 1, fpOutput);
}
free(buffer2);
fclose(fp1);
fclose(fp2);
fclose(fpOutput);
}
int main() {
const char* file1 = "image1.bmp";
const char* file2 = "image2.bmp";
const char* output = "combined.bmp";
combineBMP(file1, file2, output);
return 0;
}
```
### 回答3:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
// 定义 BMP 文件头结构体
typedef struct {
uint16_t bfType;
uint32_t bfSize;
uint16_t bfReserved1;
uint16_t bfReserved2;
uint32_t bfOffBits;
} BMPFileHeader;
// 定义 BMP 信息头结构体
typedef struct {
uint32_t biSize;
int32_t biWidth;
int32_t biHeight;
uint16_t biPlanes;
uint16_t biBitCount;
uint32_t biCompression;
uint32_t biSizeImage;
int32_t biXPelsPerMeter;
int32_t biYPelsPerMeter;
uint32_t biClrUsed;
uint32_t biClrImportant;
} BMPInfoHeader;
int main() {
// 读取第一幅图像
FILE* file1 = fopen("image1.bmp", "rb");
if (file1 == NULL) {
printf("无法打开第一幅图像文件\n");
return 0;
}
BMPFileHeader fileHeader1;
BMPInfoHeader infoHeader1;
fread(&fileHeader1, sizeof(BMPFileHeader), 1, file1);
fread(&infoHeader1, sizeof(BMPInfoHeader), 1, file1);
fseek(file1, fileHeader1.bfOffBits, SEEK_SET);
// 获取第一幅图像的像素数据
int width1 = infoHeader1.biWidth;
int height1 = infoHeader1.biHeight;
uint8_t* image1 = (uint8_t*)malloc(sizeof(uint8_t) * width1 * height1);
fread(image1, sizeof(uint8_t), width1 * height1, file1);
fclose(file1);
// 读取第二幅图像
FILE* file2 = fopen("image2.bmp", "rb");
if (file2 == NULL) {
printf("无法打开第二幅图像文件\n");
free(image1);
return 0;
}
BMPFileHeader fileHeader2;
BMPInfoHeader infoHeader2;
fread(&fileHeader2, sizeof(BMPFileHeader), 1, file2);
fread(&infoHeader2, sizeof(BMPInfoHeader), 1, file2);
fseek(file2, fileHeader2.bfOffBits, SEEK_SET);
// 获取第二幅图像的像素数据
int width2 = infoHeader2.biWidth;
int height2 = infoHeader2.biHeight;
uint8_t* image2 = (uint8_t*)malloc(sizeof(uint8_t) * width2 * height2);
fread(image2, sizeof(uint8_t), width2 * height2, file2);
fclose(file2);
// 拼接图像
int width = width1 + width2;
int height = (height1 > height2) ? height1 : height2;
uint8_t* image = (uint8_t*)malloc(sizeof(uint8_t) * width * height);
// 复制第一幅图像的数据
int y1, y2;
for (y1 = 0; y1 < height1; y1++) {
for (int x1 = 0; x1 < width1; x1++) {
int index1 = y1 * width1 + x1;
int index = y1 * width + x1;
image[index] = image1[index1];
}
}
// 复制第二幅图像的数据
for (y2 = 0; y2 < height2; y2++) {
for (int x2 = 0; x2 < width2; x2++) {
int index2 = y2 * width2 + x2;
int index = y2 * width + (x2 + width1);
image[index] = image2[index2];
}
}
// 创建新的图像文件,并保存拼接后的图像
FILE* outputFile = fopen("output.bmp", "wb");
if (outputFile == NULL) {
printf("无法创建输出文件\n");
free(image1);
free(image2);
return 0;
}
fwrite(&fileHeader1, sizeof(BMPFileHeader), 1, outputFile);
fwrite(&infoHeader1, sizeof(BMPInfoHeader), 1, outputFile);
fwrite(image, sizeof(uint8_t), width * height, outputFile);
fclose(outputFile);
free(image1);
free(image2);
free(image);
printf("图像拼接完成,已保存为 output.bmp\n");
return 0;
}
```
阅读全文
相关推荐
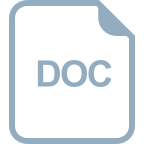
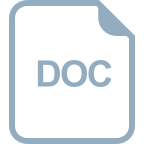
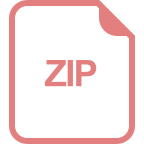
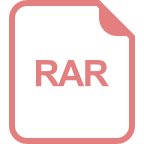
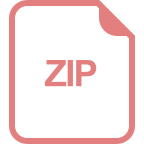
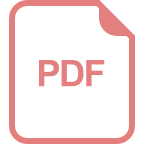
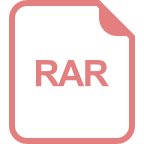
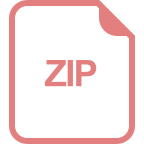
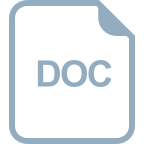
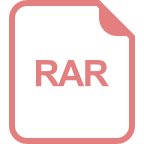
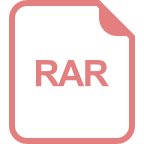
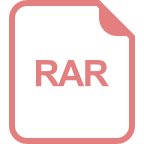