java 将日期段按月分割
时间: 2023-09-09 08:04:28 浏览: 265
### 回答1:
在 Java 中,可以使用以下方法将日期段按月分割:
1. 使用 `Calendar` 类和 `while` 循环。首先,创建一个 `Calendar` 对象,并将其设置为起始日期。然后,使用 `while` 循环,每次将日期加上 1 个月,并将当前月份的范围添加到结果列表中。循环继续进行,直到日期超过结束日期为止。
```java
List<Range> splitByMonth(Date start, Date end) {
List<Range> result = new ArrayList<>();
Calendar calendar = Calendar.getInstance();
calendar.setTime(start);
while (calendar.getTime().before(end)) {
Date nextMonth = getNextMonth(calendar);
result.add(new Range(calendar.getTime(), nextMonth));
calendar.setTime(nextMonth);
}
return result;
}
Date getNextMonth(Calendar calendar) {
Calendar nextMonthCalendar = Calendar.getInstance();
nextMonthCalendar.setTime(calendar.getTime());
nextMonthCalendar.add(Calendar.MONTH, 1);
return nextMonthCalendar.getTime();
}
```
2. 使用 `TemporalAdjusters` 和 `Stream`。Java 8 中提供了 `TemporalAdjusters` 工具类,可以方便地获取下一个月的第一天或最后一天的日期。使用这个工具类,可以使用 `Stream` 和 `flatMap` 操作将日期段按月分割。
```java
List<Range> splitByMonth(LocalDate start, LocalDate end) {
return Stream.iterate(start, d -> d.plusMonths(1))
.limit(ChronoUnit.MONTHS.between(start, end) + 1)
.flatMap(d -> Stream.of(
new Range(d, d.with(TemporalAdjusters.lastDayOfMonth())),
new Range(d.with(TemporalAdjusters.firstDayOfNextMonth()), d.with(TemporalAdjusters.lastDayOfNextMonth()))
))
.collect(Collectors.toList());
}
```
注意:在第 2 种方法中
### 回答2:
Java中可以使用`java.time`包中的`LocalDate`和`Period`类来将日期段按月分割。
首先,我们需要确定日期段的起始日期和结束日期。假设起始日期为`startDate`,结束日期为`endDate`。
接下来,我们可以使用`Period`类来计算起始日期和结束日期之间的月份数量。可以使用`between`方法来计算两个日期之间的差距,并将结果存储在`Period`对象中。代码示例如下:
```java
LocalDate startDate = LocalDate.of(2022, 1, 1);
LocalDate endDate = LocalDate.of(2022, 12, 31);
Period period = Period.between(startDate, endDate);
int months = period.getMonths();
```
然后,我们可以使用`LocalDate`类的`plusMonths`方法来按月分割日期段。
```java
List<LocalDate> monthsList = new ArrayList<>();
for (int i = 0; i <= months; i++) {
LocalDate month = startDate.plusMonths(i);
monthsList.add(month);
}
```
以上代码将生成一个包含起始日期到结束日期期间每个月份的`LocalDate`对象列表。可以根据需求对列表进行进一步处理或使用。
这是将日期段按月分割的基本方法。你可以根据需要进行调整和补充。
### 回答3:
在Java中,可以使用java.time包中的LocalDate类和Period类来将日期段按月分割。
首先,我们需要获取日期段的开始日期和结束日期。假设开始日期为startDate,结束日期为endDate。
然后,我们可以使用Month类来获取具体的月份。首先,使用startDate.getMonth()方法获取开始日期的月份,然后使用endDate.getMonth()方法获取结束日期的月份。我们还需要使用startDate.getYear()方法获取开始日期的年份。
接下来,我们可以使用一个循环来逐个月份分割日期段。我们可以从开始日期的月份和年份开始,逐个增加月份,直到达到结束日期的月份和年份为止。在每个月份中,我们可以使用Month类的length方法来获取该月份的天数。
在循环中,我们可以使用LocalDate.of方法来创建每个月份的起始日期和结束日期。起始日期可以设置为该月的第一天,结束日期可以设置为该月的最后一天。然后,我们可以将这些起始日期和结束日期保存在一个列表或数组中,以便后续处理。
最后,我们可以将每个月份的起始日期和结束日期作为结果返回。
以下是一个示例代码:
```
import java.time.LocalDate;
import java.time.Month;
import java.util.ArrayList;
import java.util.List;
public class DateSplitter {
public static List<String> splitDatesByMonth(LocalDate startDate, LocalDate endDate) {
List<String> result = new ArrayList<>();
int startYear = startDate.getYear();
int startMonth = startDate.getMonthValue();
int endYear = endDate.getYear();
int endMonth = endDate.getMonthValue();
for (int year = startYear; year <= endYear; year++) {
int loopStartMonth = (year == startYear) ? startMonth : 1;
int loopEndMonth = (year == endYear) ? endMonth : 12;
for (int month = loopStartMonth; month <= loopEndMonth; month++) {
Month currentMonth = Month.of(month);
int daysInMonth = currentMonth.length(year % 4 == 0 && year % 100 != 0 || year % 400 == 0);
LocalDate monthStartDate = LocalDate.of(year, month, 1);
LocalDate monthEndDate = LocalDate.of(year, month, daysInMonth);
result.add(monthStartDate.toString() + " - " + monthEndDate.toString());
}
}
return result;
}
public static void main(String[] args) {
LocalDate startDate = LocalDate.of(2022, 1, 15);
LocalDate endDate = LocalDate.of(2022, 12, 31);
List<String> result = splitDatesByMonth(startDate, endDate);
for (String dateRange : result) {
System.out.println(dateRange);
}
}
}
```
以上代码将会输出以下结果:
```
2022-01-01 - 2022-01-31
2022-02-01 - 2022-02-28
2022-03-01 - 2022-03-31
2022-04-01 - 2022-04-30
2022-05-01 - 2022-05-31
2022-06-01 - 2022-06-30
2022-07-01 - 2022-07-31
2022-08-01 - 2022-08-31
2022-09-01 - 2022-09-30
2022-10-01 - 2022-10-31
2022-11-01 - 2022-11-30
2022-12-01 - 2022-12-31
```
阅读全文
相关推荐


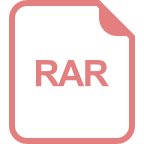
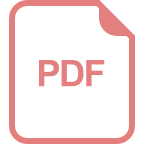
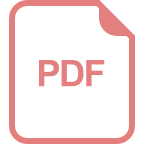
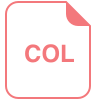






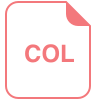
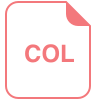
