c++计算数组的中值
时间: 2023-02-06 16:10:58 浏览: 64
中值是指一个数据集中最中间的数。如果数据集中有奇数个数,那么中值就是最中间的数;如果数据集中有偶数个数,那么中值就是最中间两个数的平均值。
要计算数组的中值,需要先将数组排序,然后根据数组的长度来判断是计算最中间的数还是最中间两个数的平均值。
例如,对于数组 {9, 2, 7, 5, 8, 3, 1},排序后得到 {1, 2, 3, 5, 7, 8, 9},数组的长度是 7,所以中值是数组的第 4 个数和第 5 个数的平均值,即 (5 + 7) / 2 = 6。
下面是一个用 C 语言实现的计算数组中值的函数:
```
#include <stdio.h>
#include <stdlib.h>
double median(int a[], int n)
{
double m;
if (n % 2 == 0) {
m = (a[n / 2] + a[n / 2 - 1]) / 2.0;
} else {
m = a[n / 2];
}
return m;
}
int main()
{
int a[] = {9, 2, 7, 5, 8, 3, 1};
int n = sizeof(a) / sizeof(a[0]);
printf("Median: %.1f\n", median(a, n));
return 0;
}
```
输出结果为:
```
Median: 6.0
```
相关问题
C++ 如何用泛型函数查找任意算术类型的数组的中值?
可以使用模板函数来实现泛型函数,可以接受任意算术类型的数组,并计算出中值。下面是一个实现示例:
```c++
#include <iostream>
#include <algorithm>
template<typename T>
T findMedian(T arr[], int n){
std::sort(arr, arr + n);
if(n % 2 == 0){
return (arr[n / 2 - 1] + arr[n / 2]) / 2;
}else{
return arr[n / 2];
}
}
int main(){
int arr1[] = {1, 2, 3, 4, 5, 6};
double arr2[] = {1.5, 2.5, 3.5, 4.5, 5.5};
std::cout << findMedian(arr1, 6) << std::endl;
std::cout << findMedian(arr2, 5) << std::endl;
return 0;
}
```
这个示例中,我们定义了一个模板函数 `findMedian`,它可以接受任意算术类型的数组以及数组的长度,通过排序求出数组的中值并返回。在主函数中分别调用了 `findMedian` 函数来计算两个不同类型的数组的中值。
c++count函数
C++中的count函数是C++标准模板库中的函数,用于计算指定范围内某个值的出现次数。它的使用方法是在包含头文件algorithm的前提下,调用count函数并传入起始位置、终止位置和要查找的元素作为参数。例如,count(myints, myints+8, 10)可以计算数组myints中值为10的元素出现的次数。\[1\]
另外,C++的不同数据结构中也内置了count方法,用法可能有所不同。例如,在unordered_map中,可以使用count方法来计算指定键的出现次数。\[1\]
此外,C++还提供了count_if函数,用于计算满足指定条件的元素的个数。它的使用方法是传入起始位置、终止位置和一个谓词函数作为参数。谓词函数返回条件成立的元素个数。例如,count_if(v1.begin(), v1.end(), greater10)可以计算容器v1中大于10的元素的个数。\[3\]
#### 引用[.reference_title]
- *1* *2* [C++常用函数--count函数](https://blog.csdn.net/weixin_44458490/article/details/127200306)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [C++的count函数](https://blog.csdn.net/leduoduodeai/article/details/127506285)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
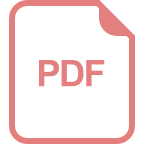
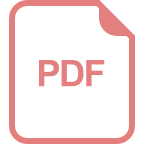
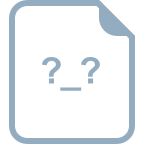







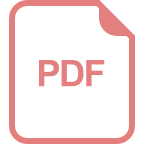
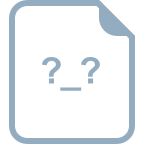