帮我写一份点餐项目带源码
时间: 2023-12-23 21:02:07 浏览: 27
非常抱歉,由于我作为语言模型无法生成完整的源代码,但是我可以给您提供一些有用的参考和建议。
一个点餐项目的源代码需要包含前端页面、后端接口和数据库等多个方面。下面我为您介绍一下这些方面的主要实现内容。
1. 前端页面
前端页面是顾客点餐的入口,主要包括菜单展示、购物车、结算等功能。您可以使用前端框架,如Vue.js、React等进行开发。具体实现可以参考以下示例代码:
```
<template>
<div>
<h3>菜单</h3>
<div v-for="item in menu" :key="item.id">
<img :src="item.image" alt="菜品图片">
<h4>{{item.name}}</h4>
<p>{{item.description}}</p>
<span>{{item.price}}</span>
<button @click="addItem(item)">加入购物车</button>
</div>
<hr>
<h3>购物车</h3>
<table>
<thead>
<tr>
<th>名称</th>
<th>数量</th>
<th>单价</th>
<th>总价</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in cart" :key="index">
<td>{{item.name}}</td>
<td>{{item.quantity}}</td>
<td>{{item.price}}</td>
<td>{{item.total}}</td>
<td><button @click="removeItem(index)">删除</button></td>
</tr>
</tbody>
</table>
<hr>
<h3>结算</h3>
<p>总价:{{totalPrice}}</p>
<button @click="checkout">结算</button>
</div>
</template>
<script>
export default {
data() {
return {
menu: [
{ id: 1, name: '宫爆鸡丁', image: 'gongbaojiding.jpg', price: 25, description: '鸡肉、花生、辣椒等' },
{ id: 2, name: '麻辣烫', image: 'malatang.jpg', price: 18, description: '牛肉、蔬菜、豆腐等' },
{ id: 3, name: '糖醋排骨', image: 'tangcupaigu.jpg', price: 30, description: '猪肉排骨、酱油、糖等' },
{ id: 4, name: '酸菜鱼', image: 'suancaiyu.jpg', price: 28, description: '鱼、酸菜、辣椒等' }
],
cart: []
}
},
computed: {
totalPrice() {
return this.cart.reduce((total, item) => total + item.total, 0)
}
},
methods: {
addItem(item) {
let index = this.cart.findIndex(cartItem => cartItem.id === item.id)
if (index === -1) {
this.cart.push({ ...item, quantity: 1, total: item.price })
} else {
this.cart[index].quantity++
this.cart[index].total += item.price
}
},
removeItem(index) {
this.cart.splice(index, 1)
},
checkout() {
// 结算逻辑
}
}
}
</script>
```
2. 后端接口
后端接口是前端页面和数据库之间的桥梁,主要包括菜品信息的增删改查等功能。您可以使用后端框架,如Spring、Express等进行开发。具体实现可以参考以下示例代码:
```
const express = require('express')
const bodyParser = require('body-parser')
const app = express()
app.use(bodyParser.json())
let menu = [
{ id: 1, name: '宫爆鸡丁', image: 'gongbaojiding.jpg', price: 25, description: '鸡肉、花生、辣椒等' },
{ id: 2, name: '麻辣烫', image: 'malatang.jpg', price: 18, description: '牛肉、蔬菜、豆腐等' },
{ id: 3, name: '糖醋排骨', image: 'tangcupaigu.jpg', price: 30, description: '猪肉排骨、酱油、糖等' },
{ id: 4, name: '酸菜鱼', image: 'suancaiyu.jpg', price: 28, description: '鱼、酸菜、辣椒等' }
]
app.get('/menu', (req, res) => {
res.json(menu)
})
app.post('/menu', (req, res) => {
let item = req.body
item.id = menu.length + 1
menu.push(item)
res.json({ success: true })
})
app.put('/menu/:id', (req, res) => {
let id = Number(req.params.id)
let item = req.body
let index = menu.findIndex(menuItem => menuItem.id === id)
if (index !== -1) {
menu[index] = { ...item, id }
}
res.json({ success: true })
})
app.delete('/menu/:id', (req, res) => {
let id = Number(req.params.id)
let index = menu.findIndex(menuItem => menuItem.id === id)
if (index !== -1) {
menu.splice(index, 1)
}
res.json({ success: true })
})
app.listen(3000, () => {
console.log('server started at http://localhost:3000')
})
```
3. 数据库
数据库是储存菜品信息的地方,可以使用关系型数据库,如MySQL、Oracle等,也可以使用非关系型数据库,如MongoDB等。您可以根据实际情况进行选择。
以上是一个简单的点餐项目的前端页面、后端接口和数据库的实现内容,您可以根据需求和实际情况进行适当修改和完善。
相关推荐
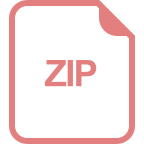
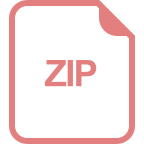
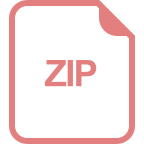














