首先随机产生两组各100个随机数,并分别存入到data1.dat和data2.dat中。要求: 编程将随机数从小到大排序后保存 将两组文件中的数据读出并输出到屏幕验证 编程将上题中的两组文件中的数据合并。要求: 将两组文件中的数据按照从小到大的顺序合并 输出(保存)到磁盘文件“result.dat”中
时间: 2023-02-06 12:28:27 浏览: 110
首先,我们需要使用 Python 的 random 模块来生成随机数。
```python
import random
# 生成 100 个随机数
data1 = [random.randint(0, 100) for _ in range(100)]
data2 = [random.randint(0, 100) for _ in range(100)]
# 将随机数保存到文件中
with open('data1.dat', 'w') as f:
for number in data1:
f.write(str(number) + '\n')
with open('data2.dat', 'w') as f:
for number in data2:
f.write(str(number) + '\n')
```
然后,我们可以使用 Python 的 sort 方法将数据从小到大排序:
```python
# 对数据进行排序
data1.sort()
data2.sort()
# 将排序后的数据保存到文件中
with open('data1.dat', 'w') as f:
for number in data1:
f.write(str(number) + '\n')
with open('data2.dat', 'w') as f:
for number in data2:
f.write(str(number) + '\n')
```
接下来,我们可以使用 Python 的 open 函数读取文件中的数据,并将数据输出到屏幕上:
```python
# 读取文件中的数据
with open('data1.dat', 'r') as f:
data1 = [int(line.strip()) for line in f]
with open('data2.dat', 'r') as f:
data2 = [int(line.strip()) for line in f]
# 输出数据
print(data1)
print(data2)
```
最后,我们可以将两组数据合并,并使用 Python 的 open 函数将数据保存到新的文件中:
```python
# 合并数据
result = data1 + data2
# 对数据进行排序
result.sort()
# 将排序后的数据保存到文件中
with open('result.dat', 'w') as
相关推荐
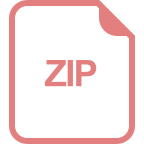














