在C#中从派生类中访问基类的成员的关键字?
时间: 2024-03-03 09:47:39 浏览: 114
在 C# 中,可以使用 `base` 关键字从派生类中访问基类的成员。`base` 关键字表示当前实例的基类实例,可以用于调用基类中的构造函数、方法、属性和字段。
以下是一些使用 `base` 关键字的示例:
1. 调用基类的构造函数
```csharp
class Animal
{
public Animal(string name)
{
Name = name;
}
public string Name { get; set; }
}
class Dog : Animal
{
public Dog(string name) : base(name)
{
// 其他初始化代码
}
}
```
在 `Dog` 类的构造函数中,使用 `base(name)` 调用了 `Animal` 类的构造函数,以初始化 `Name` 属性。
2. 调用基类的方法
```csharp
class Animal
{
public virtual void Eat()
{
Console.WriteLine("Animal is eating.");
}
}
class Dog : Animal
{
public override void Eat()
{
base.Eat(); // 调用基类的方法
Console.WriteLine("Dog is eating.");
}
}
```
在 `Dog` 类的 `Eat` 方法中,使用 `base.Eat()` 调用了 `Animal` 类的 `Eat` 方法。
3. 访问基类的属性和字段
```csharp
class Animal
{
public string Name { get; set; }
}
class Dog : Animal
{
public void PrintName()
{
Console.WriteLine(base.Name); // 访问基类的属性
}
}
```
在 `Dog` 类的 `PrintName` 方法中,使用 `base.Name` 访问了 `Animal` 类的 `Name` 属性。
阅读全文
相关推荐
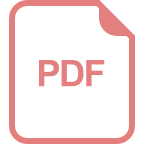
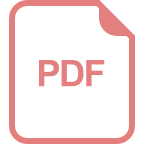


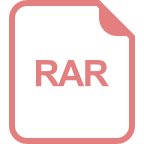
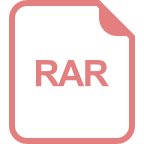
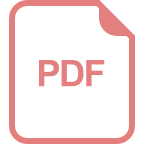
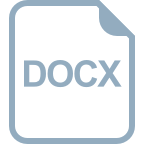
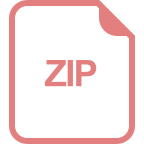
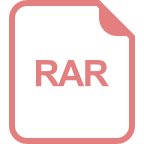
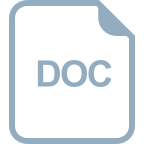
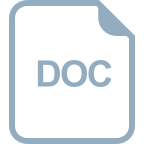
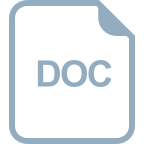
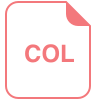


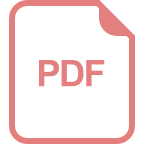
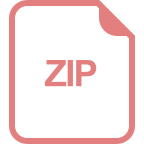