写一个ehcache3整合springboot的配置
时间: 2023-02-13 12:08:48 浏览: 153
要在 Spring Boot 中使用 Ehcache 3,您需要在 classpath 中添加以下依赖:
```
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>3.8.0</version>
</dependency>
```
接下来,您需要在 Spring Boot 应用程序的配置文件(例如 application.yml)中添加以下配置:
```
spring:
cache:
type: ehcache
```
这将告诉 Spring Boot 使用 Ehcache 3 作为缓存提供程序。
您还可以通过添加以下配置来自定义 Ehcache 3 的配置:
```
ehcache:
config: classpath:ehcache.xml
```
在这种情况下,您需要在 classpath 中添加名为 "ehcache.xml" 的配置文件。
另外,如果您希望在应用程序中使用 @Cacheable 注解来缓存方法的结果,则还需要在 Spring Boot 应用程序的启动类(例如 @SpringBootApplication 注解的类)中启用缓存注解:
```
@EnableCaching
@SpringBootApplication
public class MyApplication {
...
}
```
希望这些信息对您有帮助。
相关问题
Ehcache整合了springBoot 然后怎么输出Ehcache中的所有key
要在 Spring Boot 中输出 Ehcache 中的所有 key,需要执行以下步骤:
1. 首先,在项目中引入 Ehcache 依赖:
```
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
</dependency>
```
2. 然后,在 Spring Boot 应用程序中配置 Ehcache 缓存管理器:
```
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
return new EhCacheCacheManager(ehCacheCacheManager().getObject());
}
@Bean
public EhCacheManagerFactoryBean ehCacheCacheManager() {
EhCacheManagerFactoryBean cmfb = new EhCacheManagerFactoryBean();
cmfb.setConfigLocation(new ClassPathResource("ehcache.xml"));
cmfb.setShared(true);
return cmfb;
}
}
```
3. 在应用程序中使用 CacheManager 对象访问缓存数据:
```
@Autowired
private CacheManager cacheManager;
public void listCacheKeys() {
String[] cacheNames = cacheManager.getCacheNames();
for (String cacheName : cacheNames) {
Cache cache = cacheManager.getCache(cacheName);
System.out.println("Cache: " + cache.getName());
Iterator<Cache.Entry<Object, Object>> iter = cache.iterator();
while (iter.hasNext()) {
Cache.Entry<Object, Object> entry = iter.next();
System.out.println("Key: " + entry.getKey());
}
}
}
```
上述代码将会遍历缓存管理器中的所有缓存,并输出每个缓存中的所有 key。
Ehcache整合SpringBoot使用
好的,关于Ehcache整合Spring Boot使用,你可以按照以下步骤进行操作:
1.在pom.xml文件中添加Ehcache的依赖:
```
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>3.8.1</version>
</dependency>
```
2.在application.properties或application.yml文件中配置Ehcache:
```
spring.cache.type=ehcache
```
3.在代码中使用Ehcache:
```java
@Service
public class MyService {
@Cacheable(value = "myCache", key = "#id")
public String getFromCache(String id) {
// 从缓存中获取数据
return "data from cache";
}
@CachePut(value = "myCache", key = "#id")
public void saveToCache(String id, String data) {
// 存储数据到缓存
}
@CacheEvict(value = "myCache", key = "#id")
public void removeFromCache(String id) {
// 从缓存中删除数据
}
}
```
这样就可以使用Ehcache作为缓存存储了。希望对你有帮助!
阅读全文
相关推荐
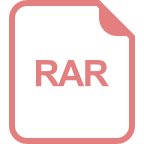
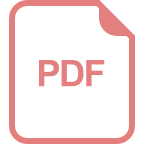








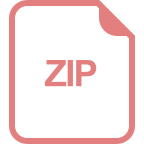
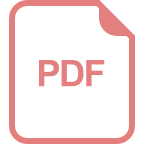
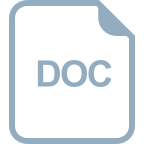
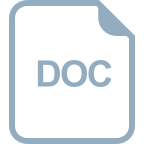

