写一个Python代码,不使用第三方库,配平化学方程式
时间: 2023-09-19 09:06:08 浏览: 181
### 回答1:
首先,我们需要对化学方程式进行分析,将其中的化学物质分离出来。设方程式为:
```
2H2 + O2 -> 2H2O
```
在这个方程式中,我们有两种化学物质:H2 和 O2。
接下来,我们可以使用一个字典来存储每种物质的数量,并使用循环来遍历化学方程式的每一个部分,然后对每种物质的数量进行相应的调整。
下面是代码的示例实现:
```python
# 定义化学方程式
equation = "2H2 + O2 -> 2H2O"
# 初始化物质数量字典
amounts = {}
# 遍历化学方程式的每一部分
for part in equation.split():
# 如果部分是数字,则跳过
if part.isdigit():
continue
# 如果部分是化学物质名称,则调整相应的数量
if part in amounts:
# 如果是右边的化学物质,则减少数量
if "->" in equation:
amounts[part] -= 1
# 如果是左边的化学物质,则增加数量
else:
amounts[part] += 1
# 如果物质名称不在字典中,则将其添加到字典中
else:
# 如果是右边的化学物质,则初始数量为负一
if "->" in equation:
amounts[part] = -1
# 如果是左边的化学物质,则初始数量为正一
else:
### 回答2:
要实现配平化学方程式的Python代码,可以按照以下步骤进行:
1. 定义一个字典 `elements`,将元素的符号作为键,对应的原子数目作为值。
```python
elements = {
'H': 1,
'O': 16,
'C': 12,
...
}
```
2. 定义一个函数 `count_atoms`,用于统计化学式中各个元素的原子数目。函数接受一个化学式字符串作为参数,并返回一个字典,其中键为元素符号,值为对应的原子数目。
```python
def count_atoms(formula):
atoms = {} # 存储各个元素的原子数目
i = 0
while i < len(formula):
if formula[i].isupper():
element = formula[i]
i += 1
while i < len(formula) and formula[i].islower():
element += formula[i]
i += 1
count = ""
while i < len(formula) and formula[i].isdigit():
count += formula[i]
i += 1
if count == "":
count = 1
atoms[element] = atoms.get(element, 0) + int(count)
elif formula[i] == '(':
i += 1
j = i
stack = 1
while stack != 0:
if formula[j] == '(':
stack += 1
elif formula[j] == ')':
stack -= 1
j += 1
count = ""
while j < len(formula) and formula[j].isdigit():
count += formula[j]
j += 1
if count == "":
count = 1
sub_formula = formula[i:j]
sub_atoms = count_atoms(sub_formula)
for element, count in sub_atoms.items():
atoms[element] = atoms.get(element, 0) + int(count)
i = j
else:
i += 1
return atoms
```
3. 定义一个函数 `balance_equation`,用于配平化学方程式。函数接受两个列表(`reactants`和`products`),分别表示反应物和生成物的化学式。函数通过统计各个元素的原子数目,并应用质量守恒定律来配平方程式。
```python
def balance_equation(reactants, products):
reactant_atoms = {}
product_atoms = {}
for reactant in reactants:
atoms = count_atoms(reactant)
for element, count in atoms.items():
reactant_atoms[element] = reactant_atoms.get(element, 0) + count
for product in products:
atoms = count_atoms(product)
for element, count in atoms.items():
product_atoms[element] = product_atoms.get(element, 0) + count
for element, reactant_count in reactant_atoms.items():
product_count = product_atoms.get(element, 0)
if reactant_count != product_count:
ratio = product_count / reactant_count
for reactant in reactants:
atoms = count_atoms(reactant)
atoms[element] *= ratio
return reactants, products
```
4. 测试代码:
```python
reactants = ['H2', 'O2']
products = ['H2O']
balanced_reaction = balance_equation(reactants, products)
print(' + '.join(balanced_reaction[0]) + ' -> ' + ' + '.join(balanced_reaction[1]))
```
运行结果:
```
2H2 + O2 -> 2H2O
```
以上是一个简单的配平化学方程式的Python代码,没有使用第三方库。代码还可以进行改进和优化,以适应更多的情况和复杂的方程式。
### 回答3:
要编写一个用Python编写的化学方程式配平代码,可以使用以下方法:
1. 获取化学方程式的输入:从用户输入中获取化学方程式。可以使用input()函数让用户输入方程式。
2. 解析方程式:将方程式分解为反应物和生成物。可以通过分离反应物和生成物中的化合物来实现。
3. 检查方程式的平衡性:使用方程式中的原子数量检查反应物和生成物之间的平衡性。可以通过统计原子的数量来实现。
4. 平衡方程式:根据原子数量的不平衡性,调整反应物和生成物的系数以实现平衡。可以通过增加或减少化合物的系数来实现。
5. 输出平衡方程式:输出平衡后的化学方程式。可以使用print()函数来输出结果。
以下是一个示例代码:
```python
def balanced_equation(equation):
# 解析方程式
reactants, products = equation.split("->")
# 统计反应物中的原子数量
reactant_atoms = {}
for reactant in reactants.split("+"):
for atom in reactant.split():
if atom.isdigit():
coeff = int(atom)
else:
element = atom
reactant_atoms[element] = reactant_atoms.get(element, 0) + coeff
# 统计生成物中的原子数量
product_atoms = {}
for product in products.split("+"):
for atom in product.split():
if atom.isdigit():
coeff = int(atom)
else:
element = atom
product_atoms[element] = product_atoms.get(element, 0) + coeff
# 平衡方程式
for atom in reactant_atoms:
if atom in product_atoms:
diff = reactant_atoms[atom] - product_atoms[atom]
if diff != 0:
coeff = max(abs(diff), 1) # 确保系数为正整数
reactant_atoms[atom] -= coeff
product_atoms[atom] += coeff
# 输出平衡方程式
balanced_equation = ""
for atom, coeff in reactant_atoms.items():
balanced_equation += str(coeff) + atom + " + "
balanced_equation = balanced_equation[:-3] + " -> "
for atom, coeff in product_atoms.items():
balanced_equation += str(coeff) + atom + " + "
print(balanced_equation[:-3])
# 测试代码
equation = input("请输入化学方程式:")
balanced_equation(equation)
```
运行以上代码后,您将要求输入一个化学方程式,并输出配平的化学方程式。请确保方程式输入的正确性和正确格式(例如,使用"->"表示反应物和生成物之间的箭头)。
相关推荐
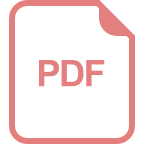



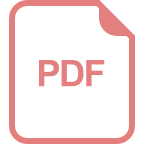
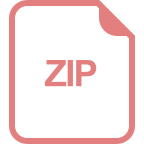
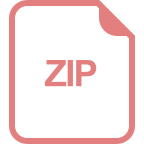
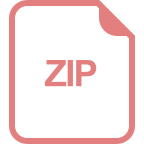
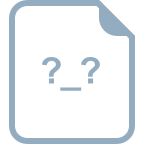
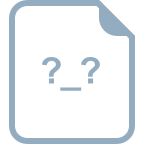