Python话网格背景的心电图
时间: 2023-08-14 22:37:03 浏览: 292
要绘制网格背景的心电图,您可以使用Python中的matplotlib库。以下是一些示例代码,可以帮助您开始:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成示例数据
x = np.linspace(0, 10, 1000)
y = np.sin(x)
# 绘制心电图
fig, ax = plt.subplots(figsize=(8, 5))
# 设置y轴范围和标签
ax.set_ylim([-2, 2])
ax.set_ylabel('Voltage (mV)')
# 设置x轴范围和标签
ax.set_xlim([0, 10])
ax.set_xlabel('Time (s)')
# 绘制网格线
ax.grid(True, linestyle='--', which='both')
# 绘制心电图线
ax.plot(x, y)
# 显示图形
plt.show()
```
此代码将生成一个大小为8x5英寸的图形,并在其中绘制心电图。网格线使用虚线样式绘制,并在x和y轴上显示标签。您可以根据需要更改这些设置。
相关问题
python绘制网格背景的心电图
要使用Python绘制网格背景的心电图,可以使用matplotlib库来实现。首先,需要导入matplotlib库,并创建一个新的图表对象。然后,可以使用plot函数来绘制心电图的波形数据。
为了绘制网格背景,可以使用grid函数来添加网格线。可以设置网格线的样式、颜色和透明度,使得网格背景更加清晰和易于阅读。
在绘制心电图的波形数据之前,可以先设置好图表的标题、坐标轴标签和图例等内容,以增强可视化效果。可以使用xlabel、ylabel和title函数来设置坐标轴标签和标题,使用legend函数来添加图例。
在绘制完成后,可以使用savefig函数保存心电图为图片文件,或者使用show函数直接显示在屏幕上。
最后,可以将整个绘制过程封装成一个函数或者类,以便于复用和扩展。这样可以更加方便地生成不同类型和不同风格的心电图,提高代码的可维护性和可扩展性。
总之,使用Python的matplotlib库可以方便地绘制网格背景的心电图,通过合理的设置参数和调用函数,可以生成美观和具有专业水准的心电图可视化效果。
读取心电图数据python画出心电图
在Python中读取心电图(ECG)数据并将其绘制成图表通常需要几个步骤:
1. **导入必要的库**:首先,你需要导入如`pandas`, `numpy`, 和 `matplotlib`等库。例如:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
```
2. **加载数据**:假设数据是以CSV或其他文本文件的形式存在,你可以使用`pandas.read_csv()`函数读取:
```python
ecg_data = pd.read_csv('ecg_data.csv')
```
或者如果是二进制文件,可以使用`pickle`或`h5py`库。
3. **预处理数据**:数据可能包含多个通道的心电信号,需要根据实际情况提取感兴趣的数据。例如,选择"Lead II"或"Lead III":
```python
signal_channel = ecg_data['Lead II']
```
4. **绘制心电图**:
```python
time = ecg_data['Time'] # 时间信息也可能在数据集中
plt.plot(time, signal_channel)
plt.xlabel('时间 (秒)')
plt.ylabel('电压 (mV)')
plt.title('ECG信号')
plt.show()
```
5. **调整图形细节**:你可以根据需要添加网格线、标记特定事件(如QRS波、T波)、或者其他自定义元素。
阅读全文
相关推荐






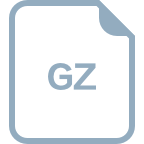









