nodejs如何创建桌面快捷方式
时间: 2023-09-22 21:06:44 浏览: 403
### 回答1:
在 Node.js 中创建桌面快捷方式的方法有以下几种:
1. 使用 `node-shortcut` 库:
可以使用 `node-shortcut` 库来创建桌面快捷方式。安装方法:
```
npm install node-shortcut
```
然后,可以使用以下代码来创建快捷方式:
```
const shortcut = require('node-shortcut');
shortcut.create('C:\\path\\to\\your\\application.exe', 'My Shortcut', {
target: 'C:\\path\\to\\your\\application.exe',
cwd: 'C:\\path\\to\\your',
icon: 'C:\\path\\to\\your\\icon.ico',
description: 'My application shortcut',
workingDir: 'C:\\path\\to\\your'
}, function(err) {
if (err) {
console.error(err);
} else {
console.log('Shortcut created successfully');
}
});
```
2. 使用 `child_process` 模块的 `exec` 方法:
可以使用 Node.js 的 `child_process` 模块的 `exec` 方法来执行系统命令,从而创建快捷方式。安装方法:
```
npm install child_process
```
然后,可以使用以下代码来创建快捷方式:
```
const childProcess = require('child_process');
const targetPath = 'C:\\path\\to\\your\\application.exe';
const shortcutPath = 'C:\\path\\to\\your\\shortcut.lnk';
const iconPath = 'C:\\path\\to\\your\\icon.ico';
const description = 'My application shortcut';
const command = `powershell -Command "$WshShell = New-Object -ComObject WScript.Shell; $Shortcut = $WshShell.CreateShortcut('${shortcutPath}'); $Shortcut.TargetPath = '${targetPath}'; $Shortcut.IconLocation = '${iconPath}'; $Shortcut.Description = '${description}'; $Shortcut.Save();"`;
childProcess.exec(command, function(err, stdout, stderr) {
if (err) {
console.error(err);
} else {
console.log('Shortcut created successfully');
}
});
```
3. 使用 `node-windows` 库:
可以使用 `node-windows
### 回答2:
在Node.js中创建桌面快捷方式可以通过使用`windows-shortcuts`模块实现。以下是使用该模块在Windows操作系统中创建桌面快捷方式的步骤:
首先,通过以下命令安装`windows-shortcuts`模块:
```bash
npm install windows-shortcuts
```
接下来,在Node.js文件中导入`windows-shortcuts`模块:
```javascript
const createShortcut = require('windows-shortcuts').create;
```
然后,创建一个新的快捷方式对象,指定目标文件路径、快捷方式名称和输出路径:
```javascript
const shortcut = createShortcut({
target: 'C:\\path\\to\\your\\file.exe',
name: 'My Shortcut',
output: 'C:\\Users\\Username\\Desktop' // 保存到桌面
});
```
在此示例中,`target`属性指定了所需创建快捷方式的文件路径,`name`属性指定了快捷方式的名称(你可以根据需要自定义),`output`属性指定了快捷方式的输出路径。
最后,使用`shortcut.save()`方法保存快捷方式文件:
```javascript
shortcut.save();
```
此方法将在指定的输出路径中创建一个快捷方式文件。
完整的创建桌面快捷方式的示例代码如下:
```javascript
const createShortcut = require('windows-shortcuts').create;
const shortcut = createShortcut({
target: 'C:\\path\\to\\your\\file.exe',
name: 'My Shortcut',
output: 'C:\\Users\\Username\\Desktop' // 保存到桌面
});
shortcut.save();
```
请注意,此方法仅适用于Windows操作系统。如果你的应用程序需要在其他操作系统上创建桌面快捷方式,你需要使用相应的模块或方法来实现。
### 回答3:
在Node.js中,可以使用第三方模块`node-os-utils`来创建桌面快捷方式。以下是基本的步骤:
1. 首先,确保已在您的项目中安装了`node-os-utils`模块。您可以使用以下命令来安装该模块:
```
npm install node-os-utils
```
2. 在您的Node.js文件中,引入所需的模块:
```javascript
const { Shortcut } = require('node-os-utils');
```
3. 使用`Shortcut`类创建一个`shortcut`对象:
```javascript
const shortcut = new Shortcut();
```
4. 使用`create`方法创建一个桌面快捷方式。您需要提供快捷方式的目标路径、名称和图标路径(可选):
```javascript
const targetPath = '/path/to/your/application.exe';
const shortcutName = 'My Application';
const iconPath = '/path/to/your/application.ico';
shortcut.create(targetPath, shortcutName, iconPath)
.then(() => {
console.log('桌面快捷方式已创建');
})
.catch((error) => {
console.error('创建桌面快捷方式时出错:', error);
});
```
5. 运行上述Node.js文件,如果一切顺利,您将在桌面上看到一个名为`My Application`的快捷方式。
请注意,以上步骤假设您的操作系统是Windows。如果您使用的是其他操作系统,可能需要使用其他模块或方法来创建桌面快捷方式。
相关推荐
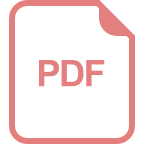
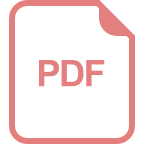














