zip 多层解压 Java
时间: 2023-02-06 08:07:46 浏览: 133
在 Java 中,可以使用 java.util.zip 包中的类来解压缩 zip 文件。
下面是一个示例代码,展示了如何解压缩多层 zip 文件:
```
import java.io.*;
import java.util.zip.*;
public class Main {
public static void main(String[] args) throws IOException {
// 将要解压缩的 zip 文件路径
String zipFilePath = "path/to/zip/file.zip";
// 解压后文件的存放目录
String destinationDirectory = "path/to/destination/directory";
// 创建解压缩对象
ZipInputStream zipIn = new ZipInputStream(new FileInputStream(zipFilePath));
// 创建缓冲区
byte[] buffer = new byte[1024];
// 获取 zip 文件中的下一个文件
ZipEntry entry = zipIn.getNextEntry();
// 循环读取文件
while (entry != null) {
// 解压缩文件名
String filePath = destinationDirectory + File.separator + entry.getName();
if (!entry.isDirectory()) {
// 如果是文件,则解压缩
extractFile(zipIn, filePath, buffer);
} else {
// 如果是目录,则创建目录
File dir = new File(filePath);
dir.mkdir();
}
// 获取下一个文件
entry = zipIn.getNextEntry();
}
zipIn.close();
}
// 解压缩文件
private static void extractFile(ZipInputStream zipIn, String filePath, byte[] buffer) throws IOException {
// 创建输出流
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(filePath));
// 循环读取数据
int read = 0;
while ((read = zipIn.read(buffer)) != -1) {
bos.write(buffer, 0, read);
}
bos.close();
}
}
```
在这段代码中,我们使用了 ZipInputStream 类来读取 zip 文件,并使用 getNextEntry 方法获取 zip 文
阅读全文
相关推荐







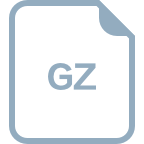
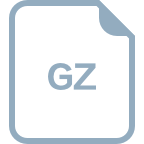
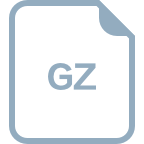
