怎么在ehcache配置类中开始计算命中率功能
时间: 2023-09-06 18:05:11 浏览: 136
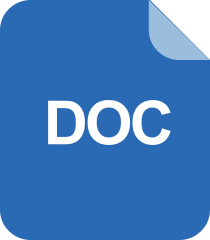
Ehcache缓存配置.doc
### 回答1:
在 ehcache 的配置类中开启命中率计算功能,你需要在配置类中指定相应的属性。
首先,在配置类中定义一个 `Cache` 对象,并指定其属性:
```
CacheConfiguration cacheConfiguration = new CacheConfiguration()
.name("myCache")
.maxEntriesLocalHeap(10000)
.eternal(false)
.timeToIdleSeconds(3600)
.timeToLiveSeconds(10800)
.diskExpiryThreadIntervalSeconds(3600)
.memoryStoreEvictionPolicy(MemoryStoreEvictionPolicy.LRU)
.persistence(new PersistenceConfiguration().strategy(Strategy.LOCALTEMPSWAP));
```
然后,在配置类中创建一个 `CacheManager` 对象,并使用前面定义的 `CacheConfiguration` 对象创建一个新的缓存:
```
CacheManager cacheManager = CacheManager.create();
Cache cache = new Cache(cacheConfiguration);
cacheManager.addCache(cache);
```
最后,你可以使用 `cache.getStatistics()` 方法来获取缓存的统计信息,包括命中率。
例如,你可以使用以下代码来获取命中率:
```
float hitRate = cache.getStatistics().getCacheHitRate();
```
你也可以在配置类中指定是否开启缓存统计功能,例如:
```
cacheConfiguration.enableStatistics(true);
```
请注意,开启缓存统计功能会增加系统的开销,因此在生产环境中应该谨慎使用。
### 回答2:
在ehcache配置类中启用命中率功能可以通过以下步骤完成:
1. 在ehcache.xml配置文件中添加一个名为"statistics"的元素,如下所示:
```xml
<cache name="myCache" maxEntriesLocalHeap="100" statistics="true">
...
</cache>
```
2. 在ehcache配置类中创建一个CacheManager实例,并在方法configure中配置缓存属性,如下所示:
```java
@Configuration
@EnableCaching
public class EhcacheConfig extends CachingConfigurerSupport {
@Bean
@Override
public CacheManager cacheManager() {
EhCacheCacheManager cacheManager = new EhCacheCacheManager();
cacheManager.setCacheManager(ehCacheManagerFactoryBean().getObject());
return cacheManager;
}
@Bean
public EhCacheManagerFactoryBean ehCacheManagerFactoryBean() {
EhCacheManagerFactoryBean cacheManagerFactoryBean = new EhCacheManagerFactoryBean();
cacheManagerFactoryBean.setConfigLocation(new ClassPathResource("ehcache.xml"));
cacheManagerFactoryBean.setShared(true);
return cacheManagerFactoryBean;
}
@Override
public KeyGenerator keyGenerator() {
return new SimpleKeyGenerator();
}
}
```
3. 在需要进行命中率统计的方法上,使用@Cacheable注解,如下所示:
```java
@Service
public class MyService {
@Cacheable("myCache")
public String getData(String key) {
// 从数据库或其他数据源获取数据的逻辑
...
}
}
```
4. 在应用程序中运行时,ehcache会自动统计每个缓存的命中率,并将统计数据打印到控制台。你也可以选择使用MBean查看缓存的命中率。
通过以上配置,你可以在ehcache配置类中启用命中率统计功能,并使用@Cacheable注解进行缓存操作。在应用程序运行期间,你可以监控缓存的命中率,并根据统计信息对缓存策略进行优化。
### 回答3:
在ehcache配置类中,可以通过添加CacheEventListener来实现命中率的计算功能。
首先,创建一个自定义的CacheEventListener类,实现接口CacheEventListener,并重写其中的方法。在方法中编写相应的逻辑来计算缓存的命中率。
添加以下方法来记录缓存的命中次数和总的查询次数:
```
@Override
public void notifyElementHit(Ehcache cache, Element element) throws CacheException {
// 缓存命中时,命中次数加1
int hitCount = cache.getHitCount();
cache.put(new Element("hitCount", hitCount + 1));
// 总的查询次数加1
int queryCount = cache.getQueryCount();
cache.put(new Element("queryCount", queryCount + 1));
}
```
然后,在ehcache配置文件中,配置CacheEventListener:
```
<cache name="exampleCache"
maxEntriesLocalHeap="100"
eternal="false"
timeToIdleSeconds="60"
timeToLiveSeconds="120">
...
<cacheEventListenerFactory class="com.example.CustomCacheEventListenerFactory"/>
</cache>
```
注意,需要将CustomCacheEventListenerFactory替换为自定义的CacheEventListener类的工厂类。
最后,在应用程序中使用ehcache时,可以通过以下方式获取命中率:
```
Cache cache = cacheManager.getCache("exampleCache");
int hitCount = (int)cache.get("hitCount").getObjectValue();
int queryCount = (int)cache.get("queryCount").getObjectValue();
double hitRate = (double)hitCount / queryCount;
System.out.println("缓存命中率:" + hitRate);
```
这样,就在ehcache的配置类中添加了命中率计算功能。当缓存命中时,命中次数和总的查询次数会更新,然后通过计算得到命中率。
阅读全文
相关推荐
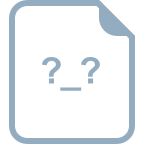
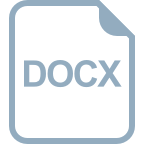
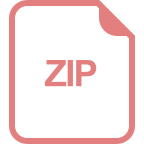
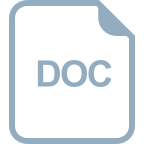
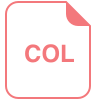
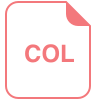
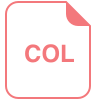
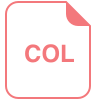
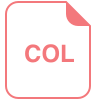
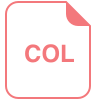
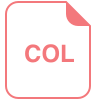
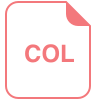
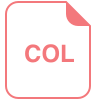
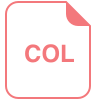
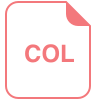
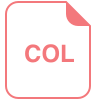
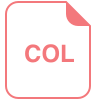
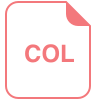