c++实现二叉排序树
时间: 2023-02-06 18:25:41 浏览: 142
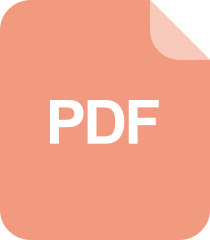
C++实现二叉排序树(插入、删除、遍历)
二叉排序树是一种特殊的二叉树,它的特点是满足二叉排序树的任意一个节点的值都大于它的左子树中的任意节点的值,而小于它的右子树中的任意节点的值。
下面是 c 语言实现二叉排序树的算法:
1. 定义二叉排序树的结构体,包含节点值、左右子节点指针。
struct BinarySortTree {
int val;
struct BinarySortTree *left;
struct BinarySortTree *right;
};
2. 定义插入节点的函数,在二叉排序树中插入新节点时,需要按照二叉排序树的特点进行比较和移动。
void insert(struct BinarySortTree **root, int val) {
// 如果根节点为空,则新建节点并将其设为根节点
if (*root == NULL) {
*root = (struct BinarySortTree*)malloc(sizeof(struct BinarySortTree));
(*root)->val = val;
(*root)->left = (*root)->right = NULL;
}
// 如果根节点不为空,则比较新节点的值和根节点的值,决定插入到根节点的左子树还是右子树
else {
if (val < (*root)->val) {
insert(&(*root)->left, val);
} else {
insert(&(*root)->right, val);
}
}
}
3. 定义中序遍历函数,用于输出二叉排序树的节点值。
void inorder(struct BinarySortTree *root) {
if (root != NULL) {
inorder(root->left);
printf("%d ", root->val);
inorder(root
阅读全文
相关推荐
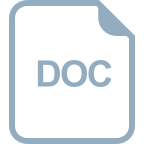
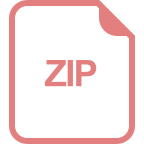















