nodejs如何传递文件名和文件内容给powershell执行文件写入操作
时间: 2023-09-22 13:06:45 浏览: 111
### 回答1:
Node.js 可以使用 child_process 模块来执行 PowerShell 脚本。你可以通过向 spawn() 函数传递文件名和文件内容作为参数来实现这一目的。
下面是一个例子:
```javascript
const { spawn } = require('child_process');
const fileName = 'example.txt';
const fileContent = 'This is the content of the file.';
const powershell = spawn('powershell.exe', [`"echo ${fileContent} > ${fileName}"`]);
powershell.stdout.on('data', function (data) {
console.log(data.toString());
});
powershell.stderr.on('data', function (data) {
console.error(data.toString());
});
powershell.on('exit', function (code) {
console.log('Child process exited with code ' + code.toString());
});
```
在上面的例子中,我们使用 spawn() 函数调用 PowerShell,并传递了一个字符串参数,该字符串包含了要执行的命令,即 `echo ${fileContent} > ${fileName}`。这条命令会在 PowerShell 中执行,并将 fileContent 的内容写入到名为 fileName 的文件中。
需要注意的是,如果文件已经存在,则会覆盖原来的文件内容。如果要在文件中追加内容,可以使用 `>>` 符号来代替 `>` 符号。
### 回答2:
在Node.js中传递文件名和文件内容给PowerShell执行文件写入操作可以通过child_process模块来实现。
首先,需要使用child_process模块中的spawn函数创建一个新的PowerShell进程。在spawn函数的参数中,指定PowerShell的执行命令以及需要执行的PowerShell脚本。
然后,通过stdin将文件名和文件内容发送给PowerShell脚本。可以使用fs模块中的readFileSync函数读取文件内容,然后将文件名和文件内容使用管道传输给PowerShell进程。
接下来,在PowerShell脚本中,可以使用$Args变量来获取从stdin读取的文件名和文件内容。使用Out-File命令将文件内容写入指定的文件名中。
最后,在Node.js中,可以通过监听PowerShell进程的exit事件来确定PowerShell脚本是否执行完毕,并根据结果进行相应的处理(如输出成功或失败信息)。
以下是一个示例代码:
```javascript
const { spawn } = require('child_process');
const fs = require('fs');
const fileName = 'example.txt';
const fileContent = 'Hello, World!';
const powershell = spawn('powershell.exe', [
'-ExecutionPolicy',
'ByPass',
'-NoProfile',
'-NoExit',
'-Command',
'-'
]);
powershell.stdin.write(`$Args[0] | Out-File -FilePath $Args[1] -Encoding utf8`, 'utf8');
powershell.stdin.write('\n');
powershell.stdin.write(fileContent);
powershell.stdin.write('\n');
powershell.stdin.write(fileName);
powershell.stdin.write('\n');
powershell.stdin.end();
powershell.on('exit', (code) => {
if (code === 0) {
console.log('文件写入成功');
} else {
console.log('文件写入失败');
}
});
```
在上述代码中,通过powershell.stdin.write()方法将文件内容和文件名发送给PowerShell进程。注意,每次发送完数据后要添加换行符,以便PowerShell脚本能够正确接收数据。
在PowerShell脚本中,使用$Args[0]来获取文件内容,使用$Args[1]来获取文件名,并通过Out-File命令将文件内容写入指定的文件名中。
最后,在Node.js中,可以通过监听PowerShell进程的exit事件来确定PowerShell脚本是否执行成功,根据返回的退出码进行相应的处理。
### 回答3:
要将文件名和文件内容传递给PowerShell执行文件写入操作,你可以使用Node.js的`child_process`模块将PowerShell脚本作为子进程执行,并通过标准输入流传递参数。
首先,你需要在Node.js中引入`child_process`模块:
```javascript
const { spawn } = require('child_process');
```
然后,可以使用`spawn`函数创建PowerShell的子进程,并传递脚本和参数:
```javascript
const fileName = 'example.txt';
const fileContent = 'Hello, World!';
const child = spawn('powershell', [
'-executionpolicy',
'bypass',
'-File',
'writeToFile.ps1',
'-fileName',
fileName,
'-fileContent',
fileContent
]);
```
在上面的示例中,我们假设PowerShell脚本文件名为`writeToFile.ps1`,该脚本将通过参数`-fileName`接收文件名,参数`-fileContent`接收文件内容。
接下来,你需要在PowerShell脚本中获取这些参数并执行文件写入操作。可以使用`process.argv`来获取传入的参数:
```powershell
param(
[string]$fileName = "",
[string]$fileContent = ""
)
Set-Content -Path $fileName -Value $fileContent
```
上述PowerShell脚本使用了`param`关键字定义了两个参数`$fileName`和`$fileContent`,并使用`Set-Content`命令将文件内容写入指定的文件。
完整的代码示例:
```javascript
const { spawn } = require('child_process');
const fileName = 'example.txt';
const fileContent = 'Hello, World!';
const child = spawn('powershell', [
'-executionpolicy',
'bypass',
'-File',
'writeToFile.ps1',
'-fileName',
fileName,
'-fileContent',
fileContent
]);
child.on('error', (err) => {
console.error(`执行PowerShell脚本时遇到错误: ${err}`);
});
child.on('exit', (code, signal) => {
if (code === 0) {
console.log('文件写入操作已成功执行');
} else {
console.error(`PowerShell脚本退出时遇到错误: ${code}`);
}
});
```
请确保将脚本文件`writeToFile.ps1`与Node.js代码文件放在同一个目录下。
相关推荐
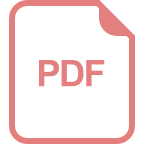













