golang中 type Formatter interface { Format(f State, verb rune) }
时间: 2024-03-13 17:43:13 浏览: 25
在 Golang 中,`Formatter` 接口是一个非常重要的接口,它定义了一个类型如何格式化自身的方法,该方法是 `Format(f State, verb rune)`。
具体来说,`Formatter` 接口定义了一个 `Format` 方法,该方法接受两个参数:
- `f State`: 表示格式化时需要的状态信息,如宽度、精度等,可以使用 `f` 中提供的方法来获取这些信息。
- `verb rune`: 表示需要进行的格式化操作,如 `%v`、`%d` 等。
在实现 `Formatter` 接口时,我们需要实现 `Format` 方法,根据 `verb` 参数来决定如何进行格式化操作,并将格式化后的结果写入 `f` 中提供的 `Write` 方法中。
下面是一个简单的示例:
```
package main
import (
"fmt"
)
type Person struct {
Name string
Age int
Height float64
}
func (p Person) Format(f fmt.State, verb rune) {
switch verb {
case 'v':
if f.Flag('+') {
fmt.Fprintf(f, "Name=%s, Age=%d, Height=%.2f\n", p.Name, p.Age, p.Height)
return
}
fmt.Fprintf(f, "Person{Name=%s, Age=%d, Height=%.2f}", p.Name, p.Age, p.Height)
case 's':
fmt.Fprintf(f, "Name=%s", p.Name)
case 'd':
fmt.Fprintf(f, "Age=%d", p.Age)
case 'f':
fmt.Fprintf(f, "Height=%.2f", p.Height)
}
}
func main() {
p := Person{"John", 30, 175.0}
fmt.Printf("%v\n", p)
fmt.Printf("%+v\n", p)
fmt.Printf("%s\n", p)
fmt.Printf("%d\n", p)
fmt.Printf("%f\n", p)
}
```
输出结果为:
```
Person{Name=John, Age=30, Height=175.00}
Name=John, Age=30, Height=175.00
Name=John
Age=30
Height=175.000000
```
在这个示例中,我们定义了一个 `Person` 结构体,并实现了 `Format` 方法,根据 `verb` 参数来决定如何进行格式化操作。在 `main` 函数中,我们使用不同的 `verb` 参数来进行格式化输出,可以看到输出结果是不同的。
相关推荐
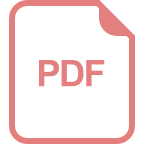
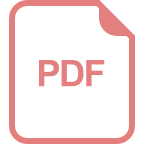














