写一个语音NLMS自适应滤波的函数
时间: 2023-12-26 19:05:43 浏览: 152
下面是一个语音NLMS自适应滤波的函数,供参考:
```python
import numpy as np
def nlms_filter(input_signal, desired_signal, filter_order, step_size, leak):
"""
Nonlinear least mean squares (NLMS) adaptive filter for speech signal denoising.
Parameters:
input_signal: array-like, 1d
The noisy speech signal to be filtered.
desired_signal: array-like, 1d
The clean speech signal used as reference for the filtering.
filter_order: int
The order of the adaptive filter.
step_size: float
The step size parameter for the NLMS algorithm.
leak: float
The leakage parameter, used to reduce the bias in the filter coefficients.
Returns:
output_signal: array-like, 1d
The filtered speech signal.
"""
# Initialize filter coefficients and output signal
filter_coeffs = np.zeros(filter_order)
output_signal = np.zeros(len(input_signal))
# Iterate over the input signal, updating the filter coefficients and generating the
# output signal sample by sample
for n in range(filter_order, len(input_signal)):
# Extract the current block of input samples and the current desired signal sample
current_input = input_signal[n - filter_order : n]
current_desired = desired_signal[n]
# Compute the output signal for the current sample
current_output = np.dot(current_input, filter_coeffs)
# Compute the error between the current desired signal sample and the current output
current_error = current_desired - current_output
# Update the filter coefficients using the NLMS algorithm
scale_factor = np.dot(current_input, current_input) + 1e-8 # Add a small number to avoid division by zero
delta_coeffs = step_size * current_error * current_input / scale_factor
filter_coeffs += delta_coeffs + leak * filter_coeffs
# Save the current output signal sample
output_signal[n] = current_output
return output_signal
```
请注意,在这里提供的代码仅供参考,您应该理解每个参数和操作的含义,并根据您的具体需求进行调整和修改。此外,该算法也可能存在一些缺陷和局限性,需要仔细评估和验证其效果。
阅读全文
相关推荐
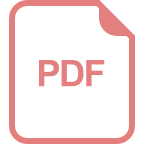
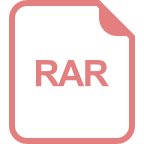
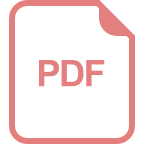















